Stack vs Heap: In C#, Stack and Heap are two important memory allocation structures that every programmer should understand.
The Stack is a Last-In-First-Out (LIFO) data structure that temporarily stores data with a short lifespan, such as function call parameters and local variables.
It is used for short-term memory allocation, ideal for data that is needed only within the scope of a method or function. The Data in the Stack memory is automatically deallocated when the method’s scope (function or block) is exited.
Stack memory is typically faster to allocate and deallocate than heap memory but has a fixed size, which can lead to stack overflow errors if exhausted.
On the other hand, A Heap is a dynamic memory allocation structure used for objects with a longer lifespan, but be aware of managing their memory properly (or relying on the garbage collector in C#).Â
Objects created using the new
keyword are typically allocated on the Heap.
The main difference between the two is that Stack memory is allocated and deallocated in a predictable and deterministic manner, while Heap memory is allocated dynamically and can lead to fragmentation over time.
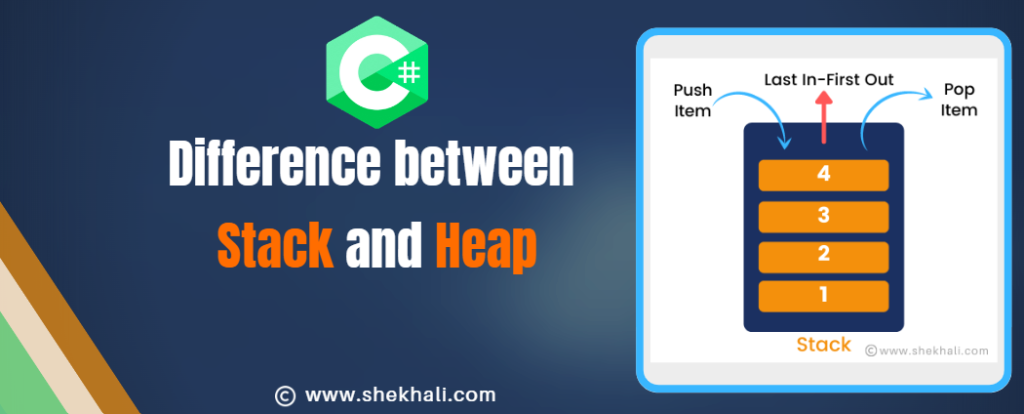
In this article, we will explore Stack and Heap’s memory, the key differences between them, their advantages and disadvantages, and when to use one over the other.
Table of Contents
What is Stack Memory?
Stack memory is a type of memory that stores temporary variables created by functions and gets freed after the function call is completed.
It is a linear data structure that follows the Last-In-First-Out (LIFO) principle.
It is useful when you need last-in, first-out access to elements.
Adding an element to the stack is called a push operation, and removing an element from the stack is called a pop operation.
The Stack memory is used to store methods, local variables, and reference variables.
Syntax:
Stack stack = new Stack();
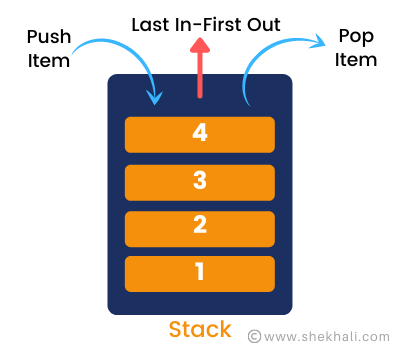
C# Stack Example
The following is an example of adding and accessing elements from the stack in C#.
using System;
using System.Collections;
namespace StackExample
{
class Program
{
public static void Main(string[] args)
{
// Creating and initializing a new Stack.
Stack stack = new Stack();
stack.Push(1);
stack.Push(2);
stack.Push(3);
stack.Push(4);
// Reading elements from the stack
foreach(var item in stack)
{
Console.Write($"{item} ,");
}
Console.ReadLine();
}
// Output: 4, 3, 2, 1,
}
}
What is Heap Memory?
Heap memory is a region of memory that is dynamically allocated during a program’s runtime. Unlike stack memory, heap memory is not freed automatically, so it is the responsibility of the Garbage Collector (GC) or programmer to deallocate it once it is no longer needed manually.
This type of memory is used for storing global variables or objects that need to persist for the duration of the program.
The Heap memory can grow or shrink dynamically based on the program’s memory requirements.
The Garbage Collector (GC) is responsible for freeing heap memory by automatically managing and reclaiming a program’s memory that is no longer being used. It tracks which objects in a heap are still in use and frees the memory associated with unneeded objects, making it available for future use.
Example 1: Heap Memory Allocation
int[] arr = new int[10];
In this example, the array arr
is stored in heap memory. This is because we use the new
operator, which dynamically allocates memory on the heap. The reference to the array is stored on the stack, whereas the actual data is stored in a heap.
C# Stack vs Heap: Key Differences
The following are the key differences between stack and heap in C#:
01. Allocation and Deallocation:
- Stack memory is automatically allocated and deallocated, whereas heap memory is manually allocated and deallocated by the Programmer or Garbage Collector (GC).
02. Access:
- Stack memory is fast and efficient but with limited access.
- Heap memory is slower but allows for greater flexibility and access.
03. Order:
- Stack memory follows a Last-In-First-Out (LIFO) order.
- Heap memory does not follow any specific order.
04. Size:
- The size of the stack is fixed and pre-allocated.
- The heap size can grow or shrink dynamically based on the program’s memory requirements.
05. Lifetime:
- The lifetime of stack memory is limited to the lifetime of the function call.
- The lifetime of heap memory persists until it is explicitly freed.
06. Memory Management:
- The system handles stack memory management.
- The programmer must handle heap memory management.
Advantages of using Stack include:
- Fast access times due to the predictable allocation and deallocation of memory.
- Easy to manage due to its simple and deterministic nature.
- Memory is automatically cleaned up when the function is executed.
- Value types, such as int, float, char, and structs, are directly stored in the Stack.
- Stack holds a variable exclusively defined within the function and not used elsewhere.
Advantages of using Heap include:
- Heap does not have any limit on memory size. Its large memory size allows for storing objects with a longer lifespan.
- Dynamic memory allocation allows for efficient memory use as it grows and shrinks as needed.
- It allows us to access variables globally.
- When you define a value type in a class, that value type will store in the Heap memory. Arrays, classes, objects, and static variables are stored in the Heap.
- No need for manual memory management as the .NET runtime automatically performs garbage collection on the Heap memory.
Disadvantages of using Stack
Using Stack can come with some drawbacks, including:
- Limited memory size leads to the risk of stack overflow if too many objects are created on the Stack.
- We can’t access elements in the middle of the Stack; It is only possible through sequential access.
- Limited functionality as it only supports LIFO (Last-In-First-Out) operations, making it unsuitable for specific data structures.
- Slow performance compared to other data structures like arrays, linked lists, and hash tables, as it requires more memory operations for adding and removing elements.
Disadvantages of using Heap
The disadvantage of using Heap memory include the following:
- Limited memory size leads to the risk of stack overflow if too many objects are created on the Stack.
- We can’t access stack elements in the middle of the Stack. It is only possible through sequential access.
- Limited functionality as it only supports LIFO (Last-In-First-Out) operations, making it unsuitable for specific data structures.
- Performance compared to other data structures like arrays, linked lists, and hash tables, as it requires more memory operations for adding and removing elements.
When to use the Heap or Stack in C#?
In C#, you should use the Stack for data with a short lifespan and small memory footprints, such as local variables and function call parameters. The Stack is a LIFO (Last-In-First-Out) data structure that is fast and efficient for temporary storage.
On the other hand, you should use the Heap memory for data with a longer lifespan and potentially large memory footprint, such as objects created with the ‘new’ keyword.
The Heap is a dynamic memory allocation structure that provides a large memory size for storing objects but can lead to slower access times and fragmentation over time.
Stack vs Heap
The following is the comparison between the stack and heap in c#.
Feature | Stack Memory | Heap Memory |
---|---|---|
Allocation: | Automatically managed by C# runtime. | Requires manual memory management |
Speed: | Fast and efficient in allocating and deallocating memory. | Slower in allocating and deallocating memory. |
Purpose: | Storing temporary data such as function call parameters, local variables, and function return addresses. | Storing longer-lived data such as objects and their data members. |
Data Structures: | A stack is ideal for small or temporary data structures. | Heap is suitable for large and dynamic data structures. |
Accessibility: | Limited to the function or thread that created the data. | Multiple Functions or Threads can access it. |
FAQs:
Q: What is Stack memory in C#?
Stack memory is a LIFO (Last-In-First-Out) data structure used for the temporary storage of data in C#. It is used to store data with a short lifespan, such as function call parameters and local variables.
Q: Explain Stack vs Heap memory in C#?
The Stack is a linear memory allocation structure that temporarily stores data with a short lifespan, such as function call parameters and local variables.
On the other hand, the Heap is a hierarchical memory allocation structure that enables dynamic memory allocation for objects with a longer lifespan, such as global variables and objects created with the new
keyword.
Q: What is the difference between Stack and Heap memory in terms of how they are used for value and reference types in C#?
Value types, such as int, float, char, and structs, directly store the data in the memory location allocated in the Stack. It means that when the function that created the value type returns, the memory for the value type is automatically cleaned up.
Reference types, such as objects and arrays, store a reference to the memory location where the data is stored in the Heap.
It allows for dynamic memory allocation and the ability for objects to persist throughout the application’s lifetime. The Heap memory is managed through garbage collection, which reclaims memory when objects are no longer used.
References: Stack Overflow-stack-vs-heap, guru99 – stack and heap memory
You might also like:
- Properties in C#
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- URL vs URI: The Ultimate Guide to Understanding the difference between URL and URI
- Readonly vs const in C#
- Fields vs Properties In C#
- C# Operators with [Examples]
- Converting Celsius to Fahrenheit in C#
- Difference Between if-else and switch
- Local vs Global Variables
- C# Struct vs Class
- Difference between interface and abstract class In C#
- C# Queue with examples
- Web API vs web service: Top 10+ Differences between web API and web service in C#
- C# Polymorphism: Different types of polymorphism in C# with examples
- C# Stack
- Understanding the Difference between == and Equals() Method in C#
- Difference between SortedList and SortedDictionary in C#
- C# Hashtable vs Dictionary vs HashSet
We would love to hear your thoughts on this post. Please leave a comment below and share it with others.
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024