A Tuple<T>
is a data structure that allows you to combine multiple elements of different data types into a single object.
It was first introduced with .NET Framework 4.0 and allowed a maximum of eight elements to be stored. Attempting to store more than eight elements will result in a compiler error.
This article will explore how to work with C# Tuples and demonstrate their usage with simple code examples.
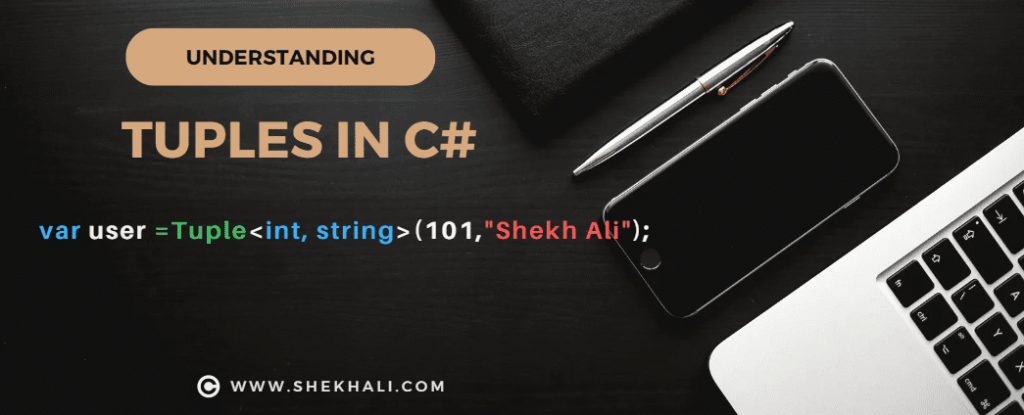
Table of Contents
- 1 Creating a Tuple in C#
- 2 C# Tuple Deconstruction
- 3 C# Tuples: Returning Multiple Values from a Method
- 4 Usage of Tuple in C#
- 5 Features of Tuples:
- 6 How to access elements from a Tuple?
- 7 Creating Nested Tuple in C#
- 8 How to use a tuple in a method?
- 9 Frequently Asked Questions (FAQs)
- 9.1 Q: What are C# Tuples, and why are they used?Â
- 9.2 Q: How do I create a Tuple in C#?Â
- 9.3 Q: Can I have different numbers of elements in a Tuple?
- 9.4 Q: How do I access elements within a Tuple?
- 9.5 Q: Can I modify the elements within a Tuple?
- 9.6 Q: How can I return multiple values from a method using Tuples?
- 9.7 Q: Can I pass Tuples as method arguments?Â
- 9.8 Q: Can I compare Tuples for equality?
- 9.9 Q: Why do C# tuples consume less memory?
- 9.10 Q: What is the maximum size of a tuple in C#?
- 9.11 Q: Is it better to use a tuple or a dictionary?
- 9.12 Related
Syntax:
Tuple<T1, T2, T3, T4, T5, T6, T7, TRest>
Creating a Tuple in C#
You can use the Tuple class to create a Tuple in C#. There are various ways to create a Tuple, depending on the number of elements you want to include.
- Creating a Tuple with Two Elements
Let’s start by creating a Tuple containing two elements, such as an integer and a string:
using System;
class Program
{
static void Main()
{
// Creating a Tuple with two elements: int and string
Tuple<int, string> studentInfo = new Tuple<int, string>(28, "Shekh Ali");
// Accessing elements of the Tuple
int age = studentInfo.Item1; // 28
string name = studentInfo.Item2; // "Shekh Ali"
// Printing the Tuple elements
Console.WriteLine($"Age: {age}, Name: {name}");
}
}
- Creating a Tuple with Three Elements
You can also create Tuples with more elements:
using System;
class Program
{
static void Main()
{
// Creating a Tuple with three elements: a boolean, a string, and an integer
Tuple<bool, string, int> data = new Tuple<bool, string, int>(true, "Hello World", 42);
// Accessing elements of the Tuple
bool flag = data.Item1; // true
string message = data.Item2; // "Hello World"
int number = data.Item3; // 42
// Printing the Tuple elements
Console.WriteLine($"Flag: {flag}, Message: {message}, Number: {number}");
}
}
C# Tuple Deconstruction
In C#, it is possible to deconstruct a Tuple into separate variables.Â
C# provides a static helper class called Tuple
, which allows you to create a Tuple<T>
instance without explicitly specifying the type of each element. This approach enhances the readability and conciseness of your code, as shown below.
C# Tuple example:
using System;
class Program
{
static void Main()
{
// Creating a Tuple with three elements
var employeeInfo = Tuple.Create(22, "Roman", true);
// Deconstructing the Tuple into separate variables
(int age, string name, bool isEmployed) = employeeInfo;
// Printing the deconstructed elements
Console.WriteLine($"Age: {age}, Name: {name}, isEmployed: {isEmployed} ");
}
}
Output:
Age: 22, Name: Roman, isEmployed: True
C# Tuples: Returning Multiple Values from a Method
We can use Tuples to return multiple values from a method. Before Tuples was introduced in C#, it was usually achieved using out parameters or creating custom classes.
using System;
class Program
{
// A method that returns multiple values as a Tuple
static Tuple<int, int> Divide(int dividend, int divisor)
{
int quotient = dividend / divisor;
int remainder = dividend % divisor;
// Returning multiple values as a Tuple
return Tuple.Create(quotient, remainder);
}
static void Main()
{
int dividend = 17;
int divisor = 4;
// Calling the method and receiving multiple values in a Tuple
var result = Divide(dividend, divisor);
// Deconstructing the Tuple into separate variables
(int quotient, int remainder) = result;
// Printing the result
Console.WriteLine($"Quotient: {quotient}, Remainder: {remainder}");
}
}
Output:
Quotient: 4, Remainder: 1
Usage of Tuple in C#
Tuples in C# provide a convenient way to work with multiple values as a single entity. They have various use cases, making them valuable in different scenarios. Here are some common usages of Tuple in C#:
- Returning Multiple Values from a Method: C# Tuples are often used when you need to return more than one value from a method without creating a custom class or using ref or out parameters.
- Grouping Different Data Types: Tuples allow you to group elements of different data types together, which is helpful when you need to temporarily handle related data as a single unit.
- Deconstructing Tuples: You can easily deconstruct a Tuple into separate variables, providing a cleaner and more readable way to access individual elements.
- Passing Multiple Parameters: Tuples can be used to pass multiple parameters to a method as a single argument, simplifying the method signature.
- Dictionary and LINQ Operations: Tuples are useful when you need to perform operations on collections or dictionaries and store temporary intermediate results.
- Error Handling: In some cases, Tuples are used to handle errors or exceptions along with additional error information.
- Anonymous Types: Tuples can be used as anonymous types when you need to represent a small, temporary data structure without defining a custom class.
- Pairs or Triplets: Tuples can represent pairs or triplets of elements, providing a straightforward alternative to using dedicated data structures.
- Temporary Data Storage: When you need to hold related values together for a short period, Tuples offer a lightweight solution without creating complex data structures.
- Pattern Matching: Tuples can be used in pattern-matching scenarios, where you want to match specific combinations of values in different cases.
Features of Tuples:
- Tuple allows storing multiple data types in a single lightweight data structure.
- Tuples support the == and != operators.
- C# Tuples enable a method to return multiple values without using the out Keyword.
- You can store duplicate elements in tuple types.
- It allows multiple values to be passed to a method with a single parameter.
- Tuple properties are read-only, ensuring they cannot be modified after creation.
- The size of a Tuple is fixed and cannot be changed once created.
Example 3:
Let’s see an example of creating a Tuple to store User information.
// C# program to demonstrate the tuple types in C#
using System;
namespace TupleExample
{
class Program
{
public static void Main(string[] args)
{
// Creating Tuple of three values Name, Id, and Address
var user = new Tuple<string, int, string>("Shekh Ali", 101, "Delhi");
Console.WriteLine("====== User's Information ======");
Console.WriteLine($" Name :{user.Item1}");
Console.WriteLine($" Id : { user.Item2}");
Console.WriteLine($" Address : {user.Item3}");
Console.ReadLine();
}
}
}
Output:
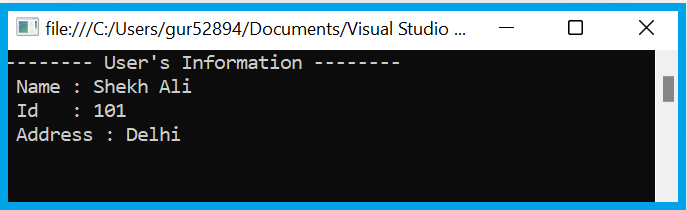
C# Tuple Example Using Create() Method
We can use static methods of the Tuple class to create a Tuple without providing the type of each element. In the following code snippet, we are using Tuple.Create() method to create a tuple.
using System;
namespace TupleExample
{
class Program
{
public static void Main(string[] args)
{
var User = Tuple.Create("Shekh Ali",28, "Delhi");
var TupleMaxLimit = Tuple.Create(1, 2, 3, 4, 5, 6, 7, 8);
Console.WriteLine($"The {nameof(User)} has the following elements: {User}");
Console.WriteLine($"The {nameof(TupleMaxLimit)} has the following elements: {TupleMaxLimit}");
Console.ReadLine();
}
}
}
Output:
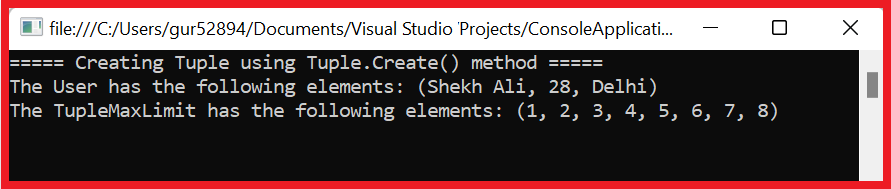
How to access elements from a Tuple?
Tuple elements can be accessed using the Item property.
For example: Item1, Item2, Item3, etc., up to the Item7 property. The Item1 property returns the first element, the Item2 property returns the second element, and so on. The last element (8th element) is returned using the Tuple’s Rest property.
Example: Accessing elements from the Tuple.
var numberList = Tuple.Create(1, 2, 3, 4, 5, 6, 7, "Eight");
Console.WriteLine(numberList.Item1); // returns 1
Console.WriteLine(numberList.Item2); // returns 2
Console.WriteLine(numberList.Item3); // returns 3
Console.WriteLine(numberList.Item4); // returns 4
Console.WriteLine(numberList.Item5); // returns 5
Console.WriteLine(numberList.Item6); // returns 6
Console.WriteLine(numberList.Item7); // returns 7
Console.WriteLine(numberList.Rest); // returns (Eight)
Console.WriteLine(numberList.Rest.Item1); // returns Eight
Console.ReadLine();
In general, the 8th position is designated for the nested tuple, which can be accessed via the Rest property.
Creating Nested Tuple in C#
You can create a tuple within another tuple. A tuple inside a tuple is called a nested tuple.
If you want your tuple to contain more than 8 elements, you can do this by nesting another tuple object as the 8th element. The last nested tuple can be accessed using the Tuple’s Rest property. To access the elements of the nested tuple, use the property. Rest.Item1.Item<ElementNumber>
Example: Nested Tuples in C#
The following code snippet illustrates how you can create a nested tuple.
// C# program to demonstrate the Nested tuple in C#
using System;
namespace NestedTupleExample
{
class Program
{
public static void Main(string[] args)
{
// Nested Tuple Example 1
var nestedTuple = Tuple.Create(100, "Shekh Ali", new Tuple<int, string>(101, "Roman"));
Console.WriteLine(nestedTuple.Item1); // 100
Console.WriteLine(nestedTuple.Item2); // Shekh Ali
Console.WriteLine(nestedTuple.Item3.Item1); // 101
Console.WriteLine(nestedTuple.Item3.Item2); // Roman
// Nested Tuple Example 2
var numberList = Tuple.Create(1, 2, 3, 4, 5, 6, 7, Tuple.Create(8, 9, 10));
Console.WriteLine(numberList.Item1); // returns 1
Console.WriteLine(numberList.Item2); // returns 2
Console.WriteLine(numberList.Item7); // returns 7
Console.WriteLine(numberList.Rest.Item1); // returns (8,9,10)
Console.WriteLine(numberList.Rest.Item1.Item1); // 8
Console.WriteLine(numberList.Rest.Item1.Item2); // 9
Console.WriteLine(numberList.Rest.Item1.Item3); // 10
Console.ReadLine();
}
}
}
The nested tuple object can be placed anywhere in the sequence. It is, however, recommended that the nested tuple be placed at the end of the sequence so that it can be accessed using the Tuple’s Rest
property.
How to use a tuple in a method?
A tuple can be used when you need to pass a data set as a single parameter of a method without using the ref and out parameters.
Let’s create a C# program to demonstrate the tuple as a method parameter in C#.
using System;
namespace TupleExample
{
class Program
{
public static void Main(string[] args)
{
// Tuple as method parameter
var tuple = Tuple.Create(100, "Shekh Ali");
Program.DisplayUser(tuple);
Console.ReadLine();
}
static void DisplayUser(Tuple<int, string> user)
{
Console.WriteLine($"Id = { user.Item1}");
Console.WriteLine($"Name = { user.Item2}");
}
// Output:
// Id = 100
// Name = Shekh Ali
}
}
Frequently Asked Questions (FAQs)
Q: What are C# Tuples, and why are they used?Â
C# Tuples are lightweight data structures that allow grouping multiple elements of different data types into a single object. They are used to temporarily hold related data as a single unit, making it convenient to work with multiple values together.
Q: How do I create a Tuple in C#?Â
You can create a Tuple in C# using the Tuple class constructor or the Tuple.Create method. For example:
// Using Tuple constructor Tuple<int, string> myTuple = new Tuple<int, string>(42, "Hello");
// Using Tuple.Create method var anotherTuple = Tuple.Create(1.5, true, "Example");
Q: Can I have different numbers of elements in a Tuple?
Yes, C# Tuples support different numbers of elements, ranging from one to eight. This flexibility allows you to choose the appropriate Tuple size based on your specific needs.
Q: How do I access elements within a Tuple?
You can access elements of a Tuple using properties like Item1, Item2, and so on, corresponding to the position of the elements.
Example:
Tuple myTuple = new Tuple(42, “Hello”);
int number = myTuple.Item1; // Accessing the first element
string message = myTuple.Item2; // Accessing the second element
Q: Can I modify the elements within a Tuple?
No, Tuple properties are read-only, meaning once a Tuple is created, its elements cannot be changed. Tuples are immutable data structures.
Q: How can I return multiple values from a method using Tuples?
You can use Tuples to return multiple values from a method without using the out
keyword. Simply, Create a Tuple within the method and return it.
For example:
static Tuple GetValues()
{
int number = 42;
string message = “Hello”;
return Tuple.Create(number, message);
}
Q: Can I pass Tuples as method arguments?Â
Yes, Tuples can be passed as method arguments, allowing you to send multiple values as a single parameter.
For example:
void ProcessTuple(Tuple data)
{
// Access and use data.Item1 and data.Item2 here
}
// Usage:
ProcessTuple(Tuple.Create(42, “Hello”));
Q: Can I compare Tuples for equality?
Yes, Tuples support the == and != operators, allowing you to compare their values for equality. However, remember that Tuples compare their elements for value equality, not reference equality.
Q: Why do C# tuples consume less memory?
Tuples are stored in a single block of memory and are immutable, so no extra space is required to store new objects.
Q: What is the maximum size of a tuple in C#?
Tuples have a limit to hold a maximum of 8 elements. If you want to create a Tuple with more than eight elements, you must create nested Tuples.
Q: Is it better to use a tuple or a dictionary?
It depends on the specific use case. If you need a simple, lightweight data structure to group multiple values of different datatypes temporarily, a Tuple is a good choice. However, if you require key-value pairs and fast data retrieval based on keys, a Dictionary would be more appropriate.
References: MSDN-Tuple types, tutorialsteacher- C#-Tuple
Recommended Articles:
- C# Struct with [Examples]
- Understanding Class vs Struct vs Record in C# with examples
- Difference Between if-else and switch: A Side-by-Side Comparison of If-Else and Switch Case
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- C# Struct vs Class |Top 15+ Differences Between C# Struct and Class
- 10 Difference between interface and abstract class In C#
- C# Hashtable vs Dictionary
- C# Nullable types: How do you work with nullable types in C#?
- What is Web API? Everything You Need to Learn
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024