In software development, designing modular and maintainable applications is very important. This is where architectural patterns come into play. One such popular pattern is the 3-Tier Architecture, which provides a structured approach to organizing code in separate layers.
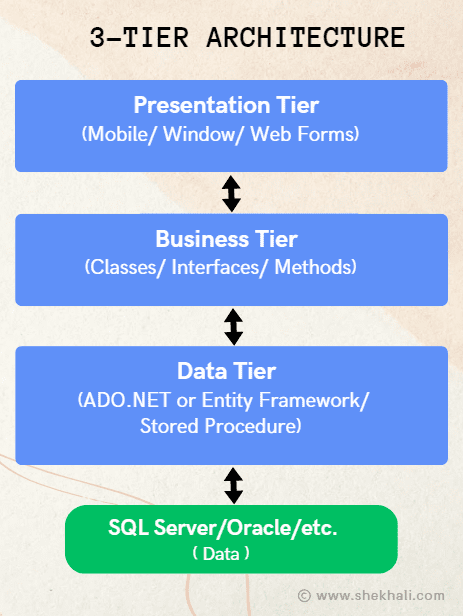
In this article, we will dive deep into the concept of 3-Tier Architecture and explore how to implement it using C#.
Table of Contents
- 1 What is 3-Tier Architecture?
- 2 Components of 3-Tier Architecture
- 3 Presentation Tier
- 4 Business tier:Â
- 5 Data Tier:Â
- 6 What are the Pros and Cons of 3-Tier Application Design?
- 7 Advantages of 3-Tier Architecture:
- 8 Drawbacks of 3-Tier Architecture:
- 9 Tier vs. Layer Architecture:
- 10 FAQs: 3-Tier Architecture in C#
- 10.1 Q: What is 3-tier architecture in C# .NET?
- 10.2 Q: How does 3-tier architecture improve maintainability?
- 10.3 Q: Can you explain the role of the Presentation Layer?
- 10.4 Q: What functions does the Business Logic Layer serve?
- 10.5 Q: How does the Data Access Layer handle database interactions?
- 10.6 Q: How does 3-tier architecture improve collaboration among developers?
- 10.7 Related
What is 3-Tier Architecture?
3-tier architecture, or multi-tier architecture, is a client-server architecture that separates an application into three distinct yet interconnected logical and physical computing tiers: the Presentation, the Business Logic, and the Data Tier.Â
These three tiers are like separate modules, each handling a specific job. They work together, but each part can be designed, developed, and maintained on a separate platform.
The key benefit of the three-tier architecture is its capacity to accommodate concurrent development. As each tier operates on its dedicated platform, separate development teams can concurrently work, update, or scale individual tiers without adversely impacting the remaining tiers.
Components of 3-Tier Architecture
The 3-Tier Architecture contains three main components:
Presentation Tier
The presentation tier is the user interface layer responsible for displaying information and collecting user input. It consists of the User Interface (UI) and focuses on providing a smooth and intuitive user experience.
The presentation tier is a top-level tier that can run on a web browser, desktop application, or a graphical user interface (GUI).
For Example:
A Web UI (presentation tiers) are usually developed using HTML, CSS and JavaScript. Depending on the platform, mobile or desktop applications can be written in various languages, such as C#, Python, Java, etc.
Example: Presentation Layer (UI):
Create your user interfaces, forms, and controls using Visual Studio or another IDE. Ensure they interact seamlessly with the business logic layer.
// MainForm.cs
public partial class MainForm : Form
{
private readonly BusinessLogicLayer _bll;
public MainForm()
{
InitializeComponent();
_bll = new BusinessLogicLayer();
}
private void SubmitButton_Click(object sender, EventArgs e)
{
string userInput = InputTextBox.Text;
string result = _bll.ProcessInput(userInput);
ResultLabel.Text = result;
}
}
Business tier:Â
The Business tier also known as the logic tier or middle tier, is the heart of the application. It contains the application’s business logic, rules, and calculations. It processes user inputs from the presentation layer and communicates with the data access layer. In C#, this layer involves defining classes that encapsulate business rules.
Example: Business tier:
// BusinessLogicLayer.cs
public class BusinessLogicLayer
{
private readonly DataAccessLayer _dal;
public BusinessLogicLayer()
{
_dal = new DataAccessLayer();
}
public string ProcessInput(string input)
{
// Apply business logic to the input
// Example: Perform calculations or validations
return _dal.SaveProcessedData(input);
}
}
Data Tier:Â
The Data tier or DAL( Data Access Layer) connects the business tier with the database or data storage. It handles data retrieval, storage, and manipulation.
In C#, this layer includes methods for querying the database using techniques like ADO.NET or Entity Framework.
Here is a simplified data access example using Entity Framework:
// DataAccessLayer.cs
using System;
using System.Data.Entity;
public class DataAccessLayer
{
public string SaveProcessedData(string data)
{
try
{
using (var dbContext = new YourDbContext()) // Replace with your actual DbContext class
{
var dataEntity = new DataEntity
{
ProcessedData = data,
Timestamp = DateTime.UtcNow
};
dbContext.DataEntities.Add(dataEntity);
dbContext.SaveChanges();
}
return "Data saved successfully!";
}
catch (Exception ex)
{
return $"An error occurred: {ex.Message}";
}
}
}
What are the Pros and Cons of 3-Tier Application Design?
The following are the Pros and Cons of a 3-tier application design.
Advantages of 3-Tier Architecture:
- Modularity and Maintainability: 3-tier architecture enforces a clear separation of concerns between layers. This modularity makes it easier to maintain and update the application. Changes in one layer are less likely to impact other layers, leading to better code maintainability.
- Reusability: Each layer can be reused independently in other projects. This promotes code reuse, reduces redundant development efforts, and improves productivity.
- Scalability: The architecture allows for individual scaling of layers based on the application’s needs. For example, if the data access layer requires more resources due to increased database interactions, it can be scaled independently without affecting the presentation or business logic layers.
- Collaboration: Developers with specialized expertise can work on different layers simultaneously, promoting collaboration within development teams. This separation also makes it easier to assign tasks based on the skills of individual team members.
- Flexibility: Since the layers are decoupled, it’s possible to replace or upgrade specific layers without affecting the application’s overall functionality. This flexibility is beneficial when adopting new technologies or making architectural improvements.
Drawbacks of 3-Tier Architecture:
- Complexity: Implementing and managing a 3-tier architecture can be more complex than a monolithic approach, especially for smaller projects. It requires careful design and coordination between layers.
- Overhead: The communication between layers can introduce some overhead, particularly in terms of performance. This overhead might be negligible for many applications but should be considered for highly performance-sensitive systems.
- Learning Curve: Developers who are new to 3-tier architecture may face a steeper learning curve. Understanding the responsibilities of each layer and establishing proper communication can require additional training.
- Deployment and Configuration: Deploying a 3-tier application may require a more sophisticated configuration, especially if the layers are distributed across different servers or environments.
- Increased Development Time: Designing and implementing the additional layers in a 3-tier architecture can extend development time, especially when compared to a monolithic approach. However, the long-term benefits of modularity and maintainability often outweigh this initial investment.
- Potential for Overengineering: A 3-tier architecture might be overkill for small or simple applications. In such cases, the added complexity may not provide significant benefits, and a simpler architecture could be more appropriate.
Tier vs. Layer Architecture:
Layer Architecture:
- What it is: A ‘layer’ is a way to organize different functions or responsibilities within a piece of software.
- Example: Think of a cake with layers – each layer has a specific job (like the Top layer being the presentation, the middle layer being the business logic, and the bottom layer handling data access).
- How it works: Layers collaborate with each other using well-defined interfaces, like team members in a group following certain guidelines.
- Contacts App Example: The Contacts app on your phone has three layers – presentation, business logic, and data handling – all working together.
Tier Architecture:
- What it is: A ‘tier’ is a way to physically separate parts of the software, with each part potentially running on different machines or servers.
- Example: Picture a building with different floors – each floor represents a tier (like the first floor being the presentation, the second floor being the business logic, and the third floor handling data storage).
- How it works: Tiers communicate with each other, but they run on separate infrastructure, like different teams working on different floors of a building.
- Contacts App Example: If the Contacts app were designed in such a way that each layer ran on a separate device (presentation on your phone, business logic on a server, and data storage on a cloud server), it would be a three-tier application.
Key Difference between Layer and tier architecture:
- Layer refers to how functions are organized within the software.
- Tier refers to how parts of the software are physically separated and can run on different infrastructures/ machines.
FAQs: 3-Tier Architecture in C#
Q: What is 3-tier architecture in C# .NET?
The 3-tier architecture is a software design pattern that divides an application into three interconnected layers: the Presentation tier (UI), the Business Logic tier, and the Data tier. These 3- tiers can be physically separated and can run on different machines.
Q: How does 3-tier architecture improve maintainability?
By separating concerns into separate layers, changes in one layer are less likely to impact other layers. This isolation makes maintenance more manageable, as updates can be made to a specific layer without affecting the entire application.
Q: Can you explain the role of the Presentation Layer?
The Presentation Layer is the user interface of the application. It’s responsible for displaying information to users and collecting user input. This layer can be a web interface (HTML, CSS, JavaScript), a desktop application with GUI elements, or any other means of user interaction.
Q: What functions does the Business Logic Layer serve?
The Business Logic Layer (BLL) contains the application’s business rules, logic, and calculations. It processes inputs from the presentation layer, performs necessary operations, and communicates with the data access layer to retrieve or store data.
Q: How does the Data Access Layer handle database interactions?
The Data Access Layer (DAL) is responsible for interacting with the database or data storage. It handles tasks such as data retrieval, storage, and manipulation. In C#, DAL can use technologies like ADO.NET or Entity Framework to connect to and manage the database such as SQL Server, Oracle, etc.
Q: How does 3-tier architecture improve collaboration among developers?
It allows developers with different expertise to work on separate layers simultaneously. This promotes collaboration within development teams, allowing experts in UI design, business logic, and data management to focus on their respective areas.
References: IBM-Three tier architecture
Recommended Articles:
- C# Tutorial
- Understanding C# Class and Objects
- C# Struct vs Class
- Class vs Struct vs Record in C#
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- Understanding SOLID design principle in C#
- C# Interface vs Abstract class
- C# Early Binding vs Late Binding
- C# For Loop
- C# While Loop
- if else vs switch condition
- C# Operators (Ternary) with examples
- Switch Statement
- Jump statements in C#
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024
- How to Reverse an Array in C# with Examples - February 12, 2024