In this article, we will learn in detail about the C# foreach loop with real-world code examples.
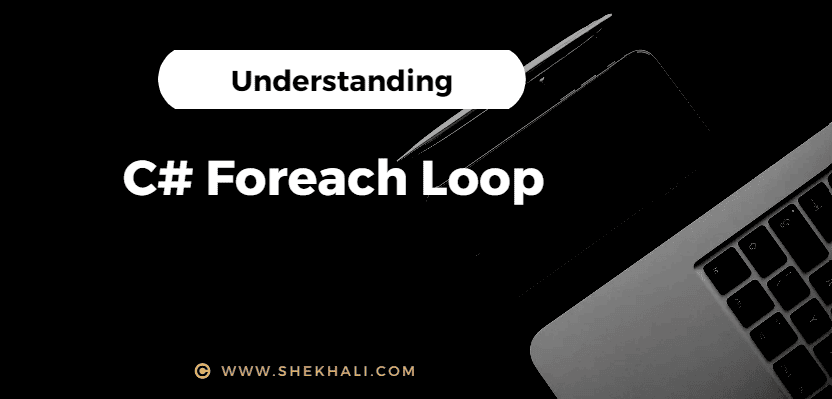
Table of Contents
- 1 Understanding the C# foreach loop
- 2 Advanced Techniques
- 3 C# foreach loop with a Dictionary
- 4 Differences between the For loop and Foreach loop in C#
- 5 Conclusion:
- 6 FAQs – C# Foreach Loop
- 6.1 Q1: What is the purpose of the foreach loop in C#?
- 6.2 Q2: Can the C# foreach loop iterate in both forward and backward directions?
- 6.3 Q3: What types of collections can the foreach loop handle?
- 6.4 Q4: Can I break out of a foreach loop prematurely?
- 6.5 Q5: How does the foreach loop differ from the for loop in C#?
- 6.6 Related
Understanding the C# foreach loop
The foreach loop in C# is used to iterate over collections such as arrays, lists, or any other type that implements the IEnumerable interface.
It iterates through each element of a collection without the need for explicit indexing.
Syntax
The basic syntax of the foreach
loop is as follows:
foreach (data_type variable_name in collection)
{
// Code to be executed for each item
}
- foreach: This keyword is the beginning of the foreach loop statement, which indicates that a loop is starting.
- data_type: Here,
data_type
represents the data type of the elements in the collection. It could be any valid C# data type, such as int, string, or a custom class type. - variable_name: This is the name given to the variable that represents the current element in the collection.
- in: The
in
keyword separates the variable declaration from the collection being iterated. - collection: This is the collection of elements over which the foreach loop iterates. It can be an array, a list, or any other data structure that implements the IEnumerable interface.
- Within the curly braces, you place the code that you want to be executed for each element in the collection.
Example 1: Iterating Through Arrays Using foreach Loop
Here is a basic program to understand the workings of the foreach loop.
In this program, we have created an array with 5 elements from 0-4, and the foreach
loop iterates through each element in the numbers
array to print them to the console.
using System;
class ArrayExample
{
static void Main()
{
// creating an array
int[] numbers = { 1, 2, 3, 4, 5 };
Console.WriteLine("Printing Numbers Using Foreach Loop:");
foreach (var number in numbers)
{
Console.WriteLine(number);
}
}
}
Output:
Printing Numbers Using Foreach Loop:
1
2
3
4
5
Example 2: Working with Lists Using foreach Loop
Lists are another commonly used collection in C#. Let’s see how the foreach loop simplifies working with lists.
using System;
using System.Collections.Generic;
class ListExample
{
static void Main()
{
List<string> fruits = new List<string> { "Apple", "Banana", "Orange", "Grapes" };
foreach (string fruit in fruits)
{
Console.WriteLine(fruit);
}
}
}
Output:
Apple
Banana
Orange
Grapes
Advanced Techniques
Getting Index and Value Using Foreach Loop
In some scenarios, you might need both the index and the value of each element during iteration using the foreach loop. C# 7 introduced the ValueTuple
feature, which allows us to achieve this more conveniently.
using System;
using System.Linq;
class IndexAndValueExample
{
static void Main()
{
string[] colors = { "Red", "Green", "Blue", "Black"};
foreach ((int index, string color) in colors.Select((value, i) => (i, value)))
{
Console.WriteLine($"Index: {index}, Color: {color}");
}
}
}
Output:
Index: 0, Color: Red
Index: 1, Color: Green
Index: 2, Color: Blue
Index: 3, Color: Black
Code Explanation:
In this code example, we use the Select
LINQ method along with ValueTuple to iterate through both the index and value of each element in the colors
array.
Iterating Over a Multidimensional Array Using Foreach Loop
The foreach loop in C# is not limited to one-dimensional arrays; it can seamlessly handle multidimensional arrays as well.
using System;
class MultiDimensionalArrayExample
{
static void Main()
{
int[,] matrix = { { 1, 2 }, { 3, 4 }, { 5, 6 } };
foreach (var element in matrix)
{
Console.Write($"{element} ");
}
}
}
Output:
1 2 3 4 5 6
This code example showcases the flexibility of the foreach
loop in traversing a two-dimensional array.
C# foreach loop with a Dictionary
In C#, a foreach loop seamlessly iterates through key-value pairs in a dictionary. We can use the KeyValuePair<TKey, TValue>
structure to access each key and value within the dictionary.
Let’s explore an example of using the C# foreach loop with a Dictionary.
using System;
using System.Collections.Generic;
class DictionaryExample
{
static void Main()
{
// Creating a Dictionary with key-value pairs of cities and their population
Dictionary<string, int> cityPopulations = new Dictionary<string, int>
{
{ "New York", 8419600 },
{ "Los Angeles", 3990456 },
{ "Chicago", 2716000 },
{ "Houston", 2328061 },
{ "Phoenix", 1680992 }
};
// Using foreach loop to iterate through the Dictionary
foreach (KeyValuePair<string, int> kvp in cityPopulations)
{
// kvp.Key represents the city name, and kvp.Value represents the population
Console.WriteLine($"City: {kvp.Key}, Population: {kvp.Value}");
}
}
}
Output:
City: New York, Population: 8419600
City: Los Angeles, Population: 3990456
City: Chicago, Population: 2716000
City: Houston, Population: 2328061
City: Phoenix, Population: 1680992
Differences between the For loop and Foreach loop in C#
The following are the Differences between the “for loop” and “foreach loop in C#”:
- Iteration Control:
- The for loop Executes a statement or block of statements until a specified condition becomes false, and it requires the definition of a minimum and maximum limit.
- The foreach loop executes a statement or block of statements for each element in the array and eliminates the need to explicitly define minimum and maximum limits.
- Direction of Iteration:
- The for loop Allows iteration in both forward and backward directions, such as from index 0 to 9 and from index 9 to 0.
- The foreach loop Restricts iteration to the forward direction only; it does not support backward iteration.
- Variable Declaration:
- A for loop requires three variable declarations for initialization, condition checking, and increment/decrement.
- A foreach loop Involves five variable declarations for accessing the current element, its index, and the array or collection itself.
- Performance Considerations:
- A for loop generally performs better in scenarios where direct control over the loop structure is essential, especially in situations with complex loop logic.
- A foreach loop offers a more concise and expressive syntax for iterating over collections but may have slightly lower performance due to the creation of a copy, making it ideal for simpler and more readable code.
- Code Readability:
- A for loop can be more verbose and requires explicit control over loop variables, making it suitable for situations where fine-grained control is necessary.
- A foreach loop promotes cleaner and more readable code by abstracting away the details of indexing, which makes it a preferred choice when simplicity and readability are prioritized.
Conclusion:
In conclusion, The foreach
loop is designed to iterate through elements in an array, collection, or other enumerable types without explicit index management. Its straightforward syntax makes it an ideal choice for simplifying iteration processes in C#. It provides a cleaner alternative to traditional For loops.
Through real-world examples, we’ve explored the foreach loop’s applicability in various scenarios, from basic array traversal to handling multidimensional structures.
References-MSDN-Iteration Statements
FAQs – C# Foreach Loop
Q1: What is the purpose of the foreach loop in C#?
The foreach loop in C# is designed to simplify the process of iterating over elements in a collection. It provides a cleaner and more readable syntax compared to traditional for loops.
The foreach loop is particularly useful for traversing arrays, lists, and other enumerable structures.
Q2: Can the C# foreach loop iterate in both forward and backward directions?
No, the foreach loop in C# can only iterate in the forward direction. It does not support backward iteration through a collection, Unlike the for loop.
Q3: What types of collections can the foreach loop handle?
The foreach
loop is versatile and can iterate over collections that implement the IEnumerable interface, such as arrays, lists, and other enumerable data structures.
Q4: Can I break out of a foreach loop prematurely?
Yes, you can break out of a foreach loop prematurely using the break
statement. This allows you to exit the loop before it completes all iterations based on a specific condition.
Q5: How does the foreach loop differ from the for loop in C#?
The for loop Executes a statement or block of statements until a specified condition becomes false, and it need to define minimum and maximum limits.
The foreach loop on the other hand, executes a statement or block of statements for each element in the array or collection, and don’t need to define explicitly limits.
Related Articles:
- C# For Loop with examples
- C# While Loop with examples
- C# Do While Loop with examples
- if else vs switch condition
- C# Operators (Ternary) with examples
- Switch Statement
- Jump statements in C#
- C# Tutorial
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024
- How to Reverse an Array in C# with Examples - February 12, 2024