In C# programming, class and objects are essential building blocks of object-oriented programming. Objects in C# are like real-world things we can touch or interact with, and classes are like plans or templates to create these objects.
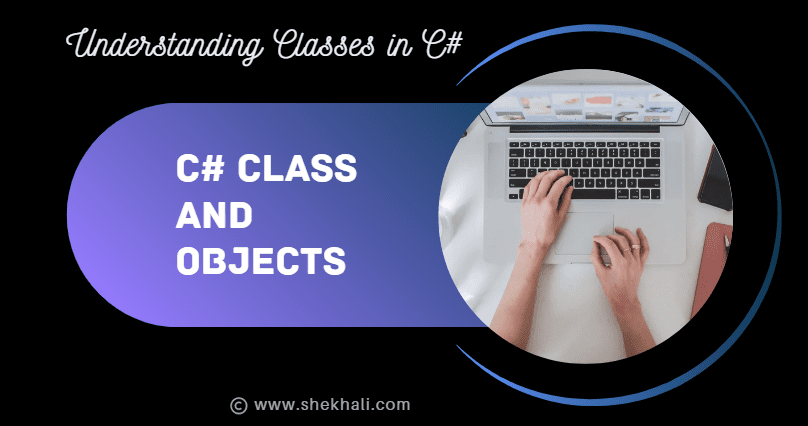
Table of Contents
What are Classes and Objects?
A Class is like a blueprint or a template that defines the properties and behaviors of an object. On the other hand, an Object is an instance of a class created based on the defined blueprint.
Let’s use a simple example to explain this concept better.
Imagine we want to create a “Car” in our program. We’ll define a blueprint called the “Car” class, which will describe what all cars have in common, like their engine, model, color, and how they can accelerate and brake.
Once we have this blueprint, we can make as many car objects as we want, each representing a different car with its own unique details.
Syntax:
The syntax to define a C# class is straightforward.
[AccessModifier] class ClassName
{
// Class members (fields, properties, methods, etc.) go here
}
Let me explain each part of the above syntax:
- Access Modifier (Optional): An access modifier defines the visibility or accessibility of the class. It determines which parts of the code can access the class. The most commonly used access modifiers are:
public
: The class is accessible from any part of the code.private
: The class is only accessible from within its containing class.protected
: The class is accessible from its containing class and derived classes.internal
: The class is accessible within the same assembly (i.e., a group of compiled files that form a logical unit).protected internal
: The class is accessible within the same assembly and from derived classes.
- Class Keyword: The class keyword defines that we are creating a class.
- ClassName: Replace “ClassName” with the desired name of your class.
- Class Members (Optional): Inside the class body, you can define various class members, such as fields, properties, methods, constructors, etc. These members define the behavior and properties of objects created from the class.
Class Definition
In C#, a class is a blueprint or a user-defined data type that serves as a template for creating objects. While a class is just a blueprint, the object is an actual instantiation of the class containing data.
Classes in C# are created using the class
keyword followed by the class name and a block of code containing the class’s members (fields, properties, methods, constructors, etc.). These members define the state and actions associated with objects instantiated from the class.
Define a Class:
// Define a Person class
public class Person
{
// Members of the class ((fields, properties, methods, constructors, etc.) ).
}
Object Definition
An object in C# is a runtime instance of a class that holds data (variables or fields) and behavior (methods) defined in the class. Objects are created using the new keyword and allow access to all class members.
Example:
// Creating an object (instance) of the Person class.
Person personObject = new Person();
Example 1: Creating a Person Class in C#.
Here is an example of a simple class named “Person” with two fields, four properties, and a method:
using System;
public class Person
{
// Private fields
private string firstName;
private string lastName;
// Public properties to access the private fields
public string FirstName
{
get { return firstName; }
set { firstName = value; }
}
public string LastName
{
get { return lastName; }
set { lastName = value; }
}
public int Age { get; set; }
public string Gender { get; set; }
public void SayHello()
{
Console.WriteLine($"Hello, my name is {FirstName} {LastName}, and I am {Age} years old {Gender}.");
}
}
class Program
{
static void Main()
{
// Create an object of the Person class
Person person = new Person();
// Set the values using the public properties
person.FirstName = "Shekh";
person.LastName = "Ali";
person.Age = 25;
person.Gender = "Male";
// Call the SayHello() method on the person object to display the message.
person.SayHello();
}
}
Output:
Hello, my name is Shekh Ali, and I am 25 years old Male.
As you can see in the above code example, We have one class called “Person”. All the Persons have some properties such as First Name, Last Name, Gender, Age, etc. These properties are nothing but the attributes (fields or properties) of the Person class.
Example2: Creating Car Class in C#
Below is a C# code example of a Car class and its objects.
using System;
// Define the Car class
public class Car
{
// Properties to represent car characteristics
public string Make; // The maker of the car, e.g., Toyota, Honda, etc.
public string Model; // The model of the car, e.g., Camry, Civic, etc.
public string Color; // The color of the car, e.g., Red, Blue, etc.
// Method to accelerate the car
public void Accelerate()
{
Console.WriteLine($"The {Make} {Model} is accelerating.");
}
// Method to brake the car
public void Brake()
{
Console.WriteLine($"The {Make} {Model} is braking.");
}
}
class Program
{
static void Main()
{
// Create a Car object based on the Car class blueprint
Car myCar = new Car();
// Set the properties of the car object
myCar.Make = "Toyota";
myCar.Model = "Camry";
myCar.Color = "Blue";
// Call the methods on the car object
myCar.Accelerate(); // Output: The Toyota Camry is accelerating.
myCar.Brake(); // Output: The Toyota Camry is braking.
// Create another Car object with different properties
Car anotherCar = new Car();
anotherCar.Make = "Honda";
anotherCar.Model = "Civic";
anotherCar.Color = "Red";
// Call the methods on the second car object
anotherCar.Accelerate(); // Output: The Honda Civic is accelerating.
anotherCar.Brake(); // Output: The Honda Civic is braking.
}
}
Output:
The Toyota Camry is accelerating.
The Toyota Camry is braking.
The Honda Civic is accelerating.
The Honda Civic is braking.
Types of classes in C#:
Below are the types of classes in C#, along with short definitions for each:
- Abstract Class: An abstract class cannot be instantiated directly. It serves as a blueprint for other classes and can have abstract and concrete members. Abstract members must be implemented in derived classes.
- Concrete Class: A concrete class can be instantiated directly. It provides a complete implementation of all its members and can be used to create objects.
- Sealed Class: A sealed class is a class that cannot be inherited. It prevents other classes from deriving from it, ensuring that the sealed class cannot be used as a base class.
- Partial Class: A partial class allow a class to be divided into multiple files using the partial keyword. It is useful for organizing large classes or when multiple developers work on different sections of the class.
- Static Class: A static class cannot be instantiated and can have only static members. It provides utility methods or constants that can be accessed directly without creating an object.
Important Points About C# Classes:
Here are some important points about C# classes:
- Blueprint for Objects: A class in C# serves as a blueprint or template for creating objects. It defines the structure and behavior of objects belonging to that class.
- Members: A class can contain various members such as fields (variables), properties, methods, events, indexers, constructors, destructors, and nested types.
- Instantiation: Objects are instances of classes. To use a class, you create an object (instance) from it using the new keyword.
- Inheritance: C# supports inheritance, allowing one class (derived or subclass) to inherit properties and behaviors from another class (base or superclass).
- Encapsulation: Classes support encapsulation, which means the internal implementation details are hidden from the outside world. Access modifiers (public, private, protected, etc.) control the visibility of class members.
- Abstraction: Abstraction is achieved through abstract classes and interfaces, which define common behaviors that derived classes must implement.
- Polymorphism: Polymorphism allows objects of different classes to be treated as objects of a common base class during runtime.
- Properties: Properties are a convenient way to expose private fields and provide get and set accessors for reading and modifying their values.
- Methods: Methods are functions defined within a class, representing the actions the objects can perform.
- Constructors: Constructors are special methods called when an object is created. They initialize the object’s state and can be overloaded to support different ways of instantiation.
- Static Members: A class can have static members (fields, properties, and methods) belonging to the class rather than individual objects.
- Access Modifiers: C# provides access modifiers (public, private, protected, internal, protected internal) to control the visibility and accessibility of class members.
- Dispose Pattern: Classes can implement the IDisposable interface to release unmanaged resources when they are no longer needed.
FAQs:
Q1: What is a class in C#?
A class in C# is a data structure containing data members (constants and fields) and function members (methods, properties, events, indexers, operators, instance constructors, and static constructors).
It also supports inheritance, allowing us to create new derived classes that inherit properties and methods from existing base classes.
Q2: What is an object in C#?
An object in C# is an instance of a class. It represents a real-world entity or data structure and can hold data and execute methods defined in the class.
Q3: How do I create a class in C#?
To create a class in C#, use the class keyword followed by the class name and a pair of curly braces. You can define the class members inside the braces, such as fields, properties, and methods.
Q4: How do I create an object in C#?
To create an object in C#, use the new
keyword followed by the class name and parentheses. This calls the class constructor and allocates memory for the object.
Q5: Can I prevent inheritance from a class in C#?
Yes, you can prevent inheritance from a class by using the sealed
keyword. A sealed class cannot be used as a base class, and no other class can derive from it.
References:MSDN-Classes
Recommended Articles:
- C# Struct vs Class
- Class vs Struct vs Record in C#
- C# Interface vs Abstract class
- C# Early Binding vs Late Binding
- C# For Loop
- C# While Loop
- if else vs switch condition
- C# Operators (Ternary) with examples
- Switch Statement
- Jump statements in C#
- C# Tutorial
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025