In this article, we will explore the differences between C# Lock and Mutex classes and understand when to use each of them.
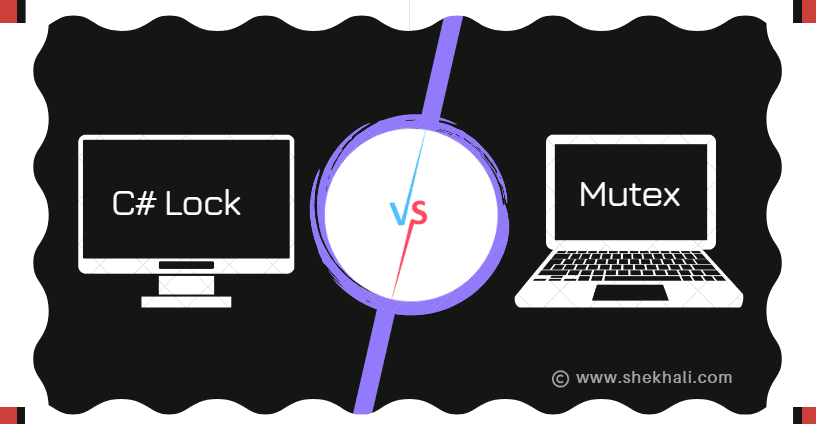
Table of Contents
Problem Statement
Consider a multi-threaded application where multiple threads need to access a critical section of code that modifies a shared resource. How can we ensure that only one thread at a time can execute that section without causing data corruption or race conditions?
To avoid such issues, synchronization techniques are used to coordinate thread access. Two commonly used synchronization mechanisms in C# are the lock statement and the Mutex class.
C# lock and Mutex are both essential tools for synchronizing threads and protecting shared resources. A lock is limited to a particular AppDomain, whereas a Mutex operates at the level of the Operating System, enabling inter-process locking and synchronization (IPC).
C# lock:
The lock statement is a simple and lightweight synchronization mechanism provided by C#. It ensures that only one thread can enter a specific code section at a time. The lock statement takes an object as a parameter, and this object is used to define the lock scope.
Here is the basic syntax of lock.
lock (lockObject)
{
// Code to be executed exclusively by one thread at a time
}
Example: C# Lock Statement
Below is a C# code example that demonstrates the use of Lock
statement.
using System;
using System.Threading;
class Program
{
static int sharedValue = 0;
static object lockObject = new object();
static void IncrementSharedValue()
{
// Using the lock statement to ensure exclusive access to sharedValue
lock (lockObject)
{
// Inside the lock block, only one thread can access this code at a time
// This ensures thread-safety when multiple threads try to increment sharedValue simultaneously
sharedValue++;
}
}
static void Main()
{
const int numThreads = 4;
Thread[] threads = new Thread[numThreads];
for (int i = 0; i < numThreads; i++)
{
threads[i] = new Thread(() =>
{
// Each thread will call IncrementSharedValue method 5000 times
for (int j = 0; j < 5000; j++)
{
IncrementSharedValue();
}
});
threads[i].Start();
}
// Wait for all threads to complete
for (int i = 0; i < numThreads; i++)
{
threads[i].Join();
}
// The final value of sharedValue will depend on the number of threads and the number of increments
Console.WriteLine("Final Shared Value: " + sharedValue);
}
}
Output:
Final Shared Value: 20000
C# Mutex:
Mutex is another synchronization mechanism in C#. Unlike lock, which is limited to a single process, Mutex can be used for inter-process synchronization. It allows multiple threads from different processes to synchronize access to shared resources.
The Mutex class uses the WaitHandle.WaitOne
method to lock and the ReleaseMutex
method to unlock. When a Mutex is closed or disposed, it is automatically released. Also, remember that you can only release a Mutex from the same thread that originally locked it.
Here is how you can use Mutex:
using System.Threading;
Mutex mutex = new Mutex();
void SomeMethod()
{
mutex.WaitOne(); // Waits until the mutex is available
try
{
// Code to be executed exclusively
}
finally
{
mutex.ReleaseMutex(); // Release the mutex
}
}
C# Lock Vs Mutex
Here’s a detailed comparison table summarizing the differences between C# lock
and Mutex
:
Aspect | C# lock | Mutex |
---|---|---|
Purpose: | C# lock synchronizes threads within a single process, which ensures that only one thread can access a shared resource at a time. | Mutex serves the same purpose but extends beyond a single process. It allows threads from different processes to synchronize access to shared resources. (Inter-Process Communication – IPC) |
Scope: | C# lock is limited to a single AppDomain (an isolated execution environment within an application). | Mutex can be used across multiple AppDomains and even different processes, facilitating synchronization between threads in various application instances. |
Keyword: | It is declared by the lock keyword followed by an object to be used as a synchronization token. | Mutex: Requires creating a new Mutex object and using the WaitOne() method to acquire the Mutex and ReleaseMutex() method to release it. |
Syntax: | lock (lockObject) { ... } | Mutex mutex = new Mutex(); ... mutex.WaitOne(); ... mutex.ReleaseMutex(); |
Synchronization Mechanism: | Monitor-based synchronization | Operating System-based synchronization. |
Exception Handling: | Lock automatically releases lock if an exception occurs within the lock block. | Mutex requires try-finally for proper cleanup. |
Portability: | Lock is limited to within the process. | Mutex can work across different processes and AppDomains |
Deadlock Detection: | C# Lock does not have a built-in deadlock detection mechanism. | Mutex provides deadlock detection and prevention |
Object Type Requirement: | Lock in C# requires an object for the lock statement | No specific object type required. a Mutex object is created independently. |
Ease of Use: | The Lock statement is simple to use with automatic lock release. | Mutex requires careful usage with try-finally , to avoid resource leaks. |
Example: C# Mutex
Below is a C# code example that demonstrates the use of Mutex
.
using System;
using System.Threading;
class Program
{
// Mutex to synchronize access to the shared resource
static Mutex mutex = new Mutex();
// Shared resource to be accessed by multiple threads
static int sharedResource = 0;
// Method that increments the shared resource in a thread-safe manner
static void IncrementSharedResource()
{
try
{
// Attempt to acquire the mutex before accessing the shared resource
mutex.WaitOne();
// Inside this block, only one thread can execute at a time
// Simulate some work being done by the thread (e.g., updating a shared resource)
int currentValue = sharedResource;
Thread.Sleep(100); // Simulate some processing time
sharedResource = currentValue + 1;
}
finally
{
// Ensure the mutex is released, even if an exception occurs
mutex.ReleaseMutex();
}
}
static void Main()
{
// Create two threads that will increment the shared resource
Thread thread1 = new Thread(IncrementSharedResource);
Thread thread2 = new Thread(IncrementSharedResource);
// Start the threads
thread1.Start();
thread2.Start();
// Wait for both threads to complete
thread1.Join();
thread2.Join();
// The shared resource should be incremented twice (once by each thread)
Console.WriteLine("Final value of the shared resource: " + sharedResource);
}
}
Output:
Final value of the shared resource: 2
FAQs: C# Lock vs. Mutex
Q: What is the main difference between C# lock and Mutex?
C# lock is used for synchronization within a single process (AppDomain), ensuring exclusive access to a shared resource.
On the other hand, Mutex extends beyond a single process, enabling inter-process synchronization, allowing threads from different processes to synchronize access to shared resources.
Q: When should I use C# lock, and when should I use Mutex?
Use C# lock when you need simple synchronization within a single process, and there is no need to synchronize across different processes.
On the other hand, use Mutex when you require synchronization across multiple processes, for example, in scenarios involving inter-process communication (IPC).
Q: What is the alternative to Lock in C#?
The alternative is a Mutex. Like a C# lock, a Mutex allows synchronization but can function across multiple processes.
Q: Which one is easier to use – C# lock or Mutex?
C# lock is generally easier to use due to its simple syntax and automatic lock release.Â
It does not require additional exception handling like Mutex, making it less error-prone for basic intra-process synchronization. However, proper usage of Mutex with try-finally ensures reliable synchronization across multiple processes.
Recommended Articles:
- Lock keyword in C# with examples
- C# Monitor class with Examples
- Understanding Class vs Struct vs Record in C# with examples
- Difference Between if-else and switch: A Side-by-Side Comparison of If-Else and Switch Case
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- C# Struct vs Class |Top 15+ Differences Between C# Struct and Class
- 10 Difference between interface and abstract class In C#
- C# Hashtable vs Dictionary
- C# Nullable types: How do you work with nullable types in C#?
- What is Web API? Everything You Need to Learn
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024
- How to Reverse an Array in C# with Examples - February 12, 2024