Dispose() and Finalize() are crucial methods for releasing resources in C#. These methods are used to clean up resources, such as database connections and files that are no longer needed. However, there are some significant differences between the dispose and finalize methods in C#.
- The
IDisposable
interface defines the Dispose() method, while the Object class defines the Finalize() method. - Users can explicitly call the Dispose() method, whereas the Finalize() method is automatically called by the garbage collector (GC) before an object is destroyed.
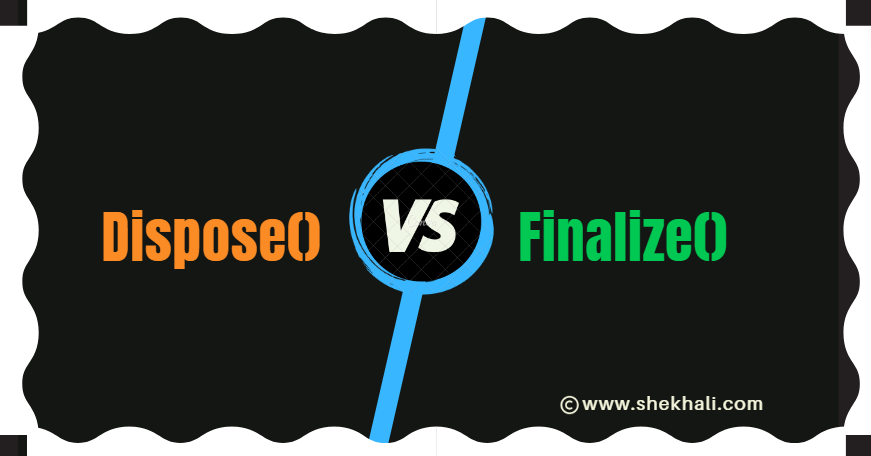
In this article, we will explore the differences between Dispose and Finalize and learn when to use them.
Table of Contents
What is Dispose() method?
The Dispose() method is used to release resources that are no longer needed. It needs to be called explicitly by the developer and is implemented in the IDisposable
interface. This interface is then implemented by types that contain unmanaged resources like file handles, database connections, and memory.
The purpose of the Dispose() method is to release these resources and prevent any potential issues that may arise from not releasing them.
What is Finalize() method?
The Finalize() method is called internally by the garbage collector when an object is being collected. Its purpose is to release any unmanaged resources that are held by that object.
It’s important to note that you cannot call this method directly as it is automatically called by the garbage collector.
Differences between Dispose and Finalize in C#
Here, We have a chart comparing some additional differences between Dispose and Finalize in C#.
Dispose() | Finalize() |
---|---|
The Dispose() method is called explicitly by the developer. | Finalize is called automatically by the garbage collector |
We can use Dispose() method to release both managed and unmanaged resources. | Finalize can only release unmanaged resources. |
Dispose is faster than finalize because it can be called Quickly and explicitly by the user and does not have to wait for the garbage collector to run. | Finalize is slower than dispose this because it is called by the garbage collector and may take some time to run. |
Dispose method belongs to the IDisposable interface. | While Finalize() belongs to the Object class. |
We can call Dispose method multiple times without any issues. | Finalize should only be called once. |
Example: Implementing Dispose() method
Following is a sample code example of how to use the dispose method in C#:
class MyClass : IDisposable
{
// Some unmanaged resource
private IntPtr handle;
public void Dispose()
{
// Release the unmanaged resource
ReleaseHandle(handle);
// Mark the object as disposed
handle = IntPtr.Zero;
GC.SuppressFinalize(this);
}
// Protected implementation of Dispose pattern.
protected virtual void Dispose(bool disposing)
{
if (disposing)
{
// Free any other managed objects here.
//
}
// Release the unmanaged resource
ReleaseHandle(handle);
// Mark the object as disposed
handle = IntPtr.Zero;
}
// Use C# destructor syntax for finalization code.
~MyClass()
{
// Simply call Dispose(false).
Dispose(false);
}
private void ReleaseHandle(IntPtr handle)
{
// Perform any necessary cleanup for the unmanaged resource here
// ...
// Release the handle
handle = IntPtr.Zero;
}
}
Code Explanation:
We have defined a class MyClass that implements the IDisposable interface in the code mentioned above, which allows the object to be disposed when it is no longer required. This class contains an unmanaged resource, represented by an IntPtr handle.
The Dispose() method releases the unmanaged resource by calling the ReleaseHandle()
method and then sets the handle to IntPtr.Zero
to mark the object as disposed. The GC.SuppressFinalize()
method is then called to prevent the object’s finalizer from being called again.
The Dispose(bool) method is a protected implementation of the Dispose pattern that is called by the Dispose() method to perform the actual resource cleanup. The method first checks the value of the disposing parameter, which indicates whether the method is being called from the Dispose() method (ex: disposing is true) or from the object’s finalizer (Ex: disposing is false).
If disposing is true, the method frees any managed objects that have been created. It then releases the unmanaged resource by calling the ReleaseHandle()
method and sets the handle to IntPtr.Zero
.
Disposing objects by Using
block
The using statement is used to automatically call the Dispose method on the object when it goes out of scope.
using (var myObject = new MyClass())
{
// Use the object here
}
The ‘using’ block is a statement used to dispose an object once you’re done with it. Disposing of an object means releasing any resources that the object may be holding onto.
The ‘using’ statement is particularly important for objects that hold onto resources that aren’t automatically released by the garbage collector, such as file handles or database connections.
Example:
Here is another example of how to use the using
block in C#:
using (FileStream fs = new FileStream("testFile.txt", FileMode.Open))
{
// Use the object here.
}
In the above example, We have created a FileStream object and opened it for reading. When the using block is exited, the FileStream object is automatically disposed, which releases the file handle that it was holding onto. This is equivalent to calling the Dispose method on the object manually, like this:
FileStream fileStream = new FileStream("testFile.txt", FileMode.Open);
try
{
// Use the object here.
}
finally
{ // Disposing object
fileStream.Dispose();
}
Using Finalize method in C#
To use the finalize()
method in C#, you must first define a destructor in the class. The garbage collector will call this destructor when the object is being collected. In the example below, the destructor calls the ReleaseHandle()
method to release the unmanaged resource and then sets the handle to null to mark the object as disposed.
It’s important to note that you don’t need to call the finalize() method yourself. Instead, the garbage collector will automatically make a call to it. However, it’s important to remember that finalize() is slower than dispose()
because the garbage collector calls it.
Therefore, it should only be used as a last alternative when an object holds unmanaged resources and doesn’t implement the IDisposable
interface.
class MyClass
{
// Define some unmanaged resource
private IntPtr handle;
// Using C# destructor syntax for finalization code.
~MyClass()
{
// Release the unmanaged resource
ReleaseHandle(handle);
// Mark the object as disposed
handle = IntPtr.Zero;
}
}
Dispose method rules in C#
Below are some important rules to follow when working with the dispose()
method in C#:
- Implement IDisposable interface:Â The
IDisposable
interface needs to be implemented to use the dispose method. You also need to provide a Dispose method implementation in your class. - Release unmanaged resources:Â The Dispose method should release any unmanaged resources held by the object like file handles, database connections, memory, etc.
- Avoid unnecessary garbage collection:Â After calling the Dispose method, you should call the
GC.SuppressFinalize()
method to prevent the Finalize method from being called and avoid unnecessary garbage collection. This is because the Finalize method is not needed if the Dispose method has already released the resources held by the object. - Call Dispose on base class:Â If your class inherits from a class that implements
IDisposable
, you should call the Dispose method on the base class in your implementation of the Dispose method. - Mark object as disposed:Â After releasing the resources, you should set any relevant object fields to null or a default value to mark the object as disposed.
- Handle multiple calls to Dispose:Â Your Dispose method implementation should be able to handle being called multiple times without causing any issues. Subsequent calls to Dispose after the first one can simply return immediately.
- Use the using statement:Â To ensure that the Dispose method is called automatically, you should use the using statement when working with objects that implement
IDisposable
. This will ensure that the Dispose method is called when the object goes out of scope.
Finalize method rules in C#
Here are some rules that you should follow while using the finalize()
method in C#:
- Provide a destructor: You must provide a destructor for your class to use the finalize() method. The destructor is called by the garbage collector when the object is collected.
- Do not call finalize directly: The finalize method should not be called directly because it is automatically called by the garbage collector.
- Avoid using finalize: You should avoid using finalize whenever possible. It is slower than the dispose method and should only be used as a last resort when an object holds on to unmanaged resources and does not implement the
IDisposable
interface. - Do not throw exceptions in finalize: You should not throw exceptions from the finalize method because the garbage collector does not catch such exceptions. If an exception is thrown, it might terminate the process.
FAQs:
Here are some common questions and answers about dispose
and finalize
in C#:
Q: What is the difference between dispose and finalize in C#?
Dispose and Finalize are two methods in C# used to release resources that are no longer needed.
Dispose() method is implemented in the IDisposable interface and is called explicitly by the user. This method can release both managed and unmanaged resources and is faster than Finalize.
On the other hand, Finalize() is a method of the Object class that is called automatically by the garbage collector when an object is being collected. Finalize is used to release only unmanaged resources held by an object and is slower than Dispose due to its automatic invocation by the garbage collector.
Q: When should you use the dispose method?
The Dispose()Â method should be used whenever you have an object that holds an unmanaged resources and implements the IDisposable
interface. Once you are finished using the object, it is necessary to call the Dispose method to release its resources, such as database connections or files. This ensures that the resources are properly released and will not cause issues later on.
Q: When should you use the finalize?
The Finalize() is a method of the Object class that is responsible for performing cleanup tasks before an object is destroyed. It is called automatically by the GC (garbage collector) before removing an object from the memory. It is basically used to release unmanaged resources held by an object.
Q: Is it safe to call dispose multiple times?
Yes, you can call dispose multiple times without any issue. Your implementations of IDisposable.Dispose()
method should tolerate being called multiple times. All subsequent calls to Dispose() can simply return right away after the first call.
Q: Is dispose method is faster than finalize?
Yes, dispose method is faster than finalize because it can be called explicitly and does not have to wait for the garbage collector to run. Finalize is slower because it is called by the garbage collector and may not run for some time.
Reference: MSDN-Finalize method, Implement a Dispose method
You might also like:
- Garbage Collection in C#
- C# Struct vs Class |Top 15+ Differences Between C# Struct and Class
- C# Queue with examples
- Web API vs web service: Top 10+ Differences between web API and web service in C#
- C# Polymorphism: Different types of polymorphism in C# with examples
- C# Stack
- C# Hashtable
- Generic Delegates in C# With Examples
- IEnumerable Interface in C# with examples
- Constructors in C# with Examples
- C# Enum | How to Use enumeration type in C#?
- Properties In C# with examples
- 10 Difference between interface and abstract class In C#
- What is a delegate in C#? Multicast Delegate with Examples
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024
- How to Reverse an Array in C# with Examples - February 12, 2024