When software developers want to write organized, clean, maintainable, and scalable code for their projects, they use design principles. One of these principles is called SOLID, which contains five rules that help to improve software design and structure.
- Single Responsibility Principle (SRP)
- Open-Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
In this article, we will focus on the first rule of SOLID
principle, called the Single Responsibility Principle (SRP) with examples.
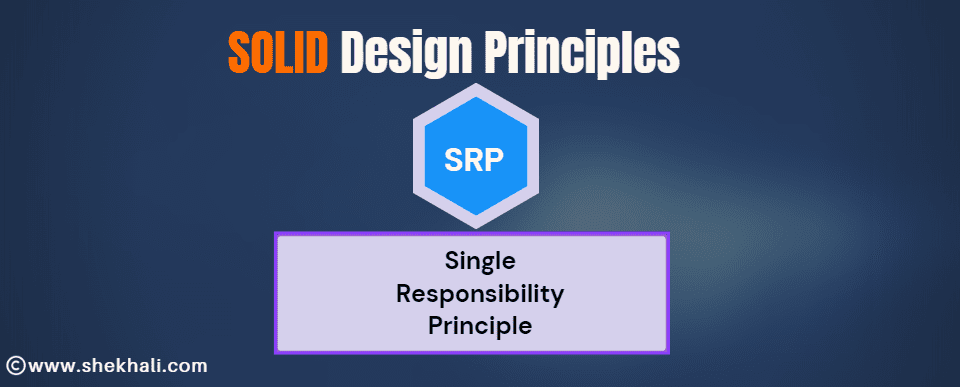
Table of Contents
- 1 What is the Single Responsibility Principle?
- 2 Benefits of the Single Responsibility Principle
- 3 Applying the Single Responsibility Principle in C#
- 4 Understanding SRP Using Real World Example
- 5 Example 2: Without Single Responsibility Principle (SRP)
- 6 Example 2: With Single Responsibility Principle (SRP)
- 7 What are the Potential Downsides of the Single Responsibility Principle SRP?
- 8 Summary:
- 9 FAQs
What is the Single Responsibility Principle?
The Single Responsibility Principle states that a class should have only one reason to change. In other words, each class should have a single responsibility or a single task to accomplish. By adhering to this principle, we can ensure that our code remains modular, maintainable, and easier to understand.
Benefits of the Single Responsibility Principle
The Single Responsibility Principle (SRP) offers several benefits when applied effectively in software development:
- Increased code maintainability: SRP promotes code maintainability by ensuring that each class or module has a single responsibility. It makes it easier to understand, modify, and troubleshoot code, as changes in one area are less likely to impact other unrelated areas.
- Improved testability: Testing becomes simpler when classes have a single responsibility. With a clear understanding of what a class should do, writing focused and particular unit tests becomes easier.
- Enhanced code readability: SRP improves code readability by keeping classes focused and concise. SRP ensures classes have a clear purpose, making it easier for developers to understand their functionality.
- Enhanced code reusability: Classes with well-defined responsibilities can be easily reused in other parts of the application. It reduces code duplication and promotes the use of modular components.
- Reduced code complexity: By following SRP, code complexity is minimized. Each class or module focuses on a specific task, leading to simpler and more manageable code. It makes code easier for us to understand and maintain.
- Better collaboration: The Single Responsibility Principle encourages better collaboration among developers. Each class’s purpose is clearly defined, making it easier for different team members to work on separate parts of the codebase concurrently.
What does “responsibility” mean in the context of the Single Responsibility Principle (SRP)?
In the Single Responsibility Principle (SRP) context, responsibility refers to a specific task, duty, or role that a class or module is assigned to perform. It represents a cohesive and distinct area of functionality or behavior within the software system. Assigning a single responsibility to a class means that it should have only one clear purpose or reason to change.
Applying the Single Responsibility Principle in C#
Let’s consider a simple example of a class that violates the Single Responsibility Principle (SRP) and then refactor it to adhere to SRP.
Example: Without SRP
public class Customer
{
public string Name { get; set; }
public string Email { get; set; }
public void Register()
{
// Register the customer
// Send welcome email
// Log registration activity
// Update customer statistics
// ...
}
}
In the above code example, the Customer class is responsible for both registering a customer and performing additional tasks like sending a welcome email, logging activity, and updating statistics. It violates SRP because the class has multiple responsibilities.
Example: Following SRP
public class Customer
{
public string Name { get; set; }
public string Email { get; set; }
}
public class CustomerRegistration
{
public void RegisterCustomer(Customer customer)
{
// Register the customer
}
}
public class EmailSender
{
public void SendWelcomeEmail(Customer customer)
{
// Send welcome email
}
}
public class ActivityLogger
{
public void LogRegistrationActivity(Customer customer)
{
// Log registration activity
}
}
public class StatisticsUpdater
{
public void UpdateCustomerStatistics(Customer customer)
{
// Update customer statistics
}
}
In the refactored code, we have separated the responsibilities into individual classes. The Customer class now solely focuses on representing customer data. The CustomerRegistration class handles registering the customer, the EmailSender class is responsible for sending welcome emails, the ActivityLogger class handles logging registration activity, and the StatisticsUpdater class updates customer statistics.
Understanding SRP Using Real World Example
Let’s consider a real-world scenario of an online shopping application. I’ll provide code examples demonstrating a Single Responsibility Principle (SRP) violation and then refactor the code to adhere to SRP.
Example 2: Without Single Responsibility Principle (SRP)
using System;
public class ShoppingCart
{
public void AddItem(Product product)
{
// Add item to the shopping cart
}
public void RemoveItem(Product product)
{
// Remove item from the shopping cart
}
public void CalculateTotal()
{
// Calculate total price
}
public void Checkout()
{
// Process the payment
// Send order confirmation email
// Update inventory
// Log order details
// ...
}
}
public class Product
{
public string Name { get; set; }
public decimal Price { get; set; }
}
In the above code example, the ShoppingCart class violates SRP
as it is responsible for managing the shopping cart, calculating the total price, and handling the checkout process, which involves payment processing, sending emails, updating inventory, and logging order details.
Example 2: With Single Responsibility Principle (SRP)
using System;
public class ShoppingCart
{
private readonly CartManager cartManager;
private readonly PaymentProcessor paymentProcessor;
public ShoppingCart()
{
cartManager = new CartManager();
paymentProcessor = new PaymentProcessor();
}
public void AddItem(Product product)
{
cartManager.AddItem(product);
}
public void RemoveItem(Product product)
{
cartManager.RemoveItem(product);
}
public decimal CalculateTotal()
{
return cartManager.CalculateTotal();
}
public void Checkout()
{
paymentProcessor.ProcessPayment();
}
}
public class CartManager
{
public void AddItem(Product product)
{
// Add item to the shopping cart
}
public void RemoveItem(Product product)
{
// Remove item from the shopping cart
}
public decimal CalculateTotal()
{
// Calculate total price
return 0;
}
}
public class PaymentProcessor
{
public void ProcessPayment()
{
// Process the payment
}
}
public class Product
{
public string Name { get; set; }
public decimal Price { get; set; }
}
In the refactored code, we have separated the responsibilities into individual classes. The ShoppingCart
class now delegates cart management tasks to the CartManager
class, which handles adding items, removing items, and calculating the total price. The PaymentProcessor
class is responsible for payment processing.
What are the Potential Downsides of the Single Responsibility Principle SRP?
While the Single Responsibility Principle (SRP) offers countless benefits, there are a few potential downsides to consider:
- Increased number of classes: Applying the
Single Responsibility Principle (SRP)
often leads to a larger number of smaller classes or modules, each dedicated to a specific responsibility. As a result, the code may expand, requiring additional effort to manage and navigate. - Higher complexity in relationships: When responsibilities are divided into separate classes, there is a possibility of increased complexity in managing relationships and dependencies between these classes.
- Over-engineering: In some cases, strictly sticking to SRP can lead to over-engineering, resulting in additional time and effort spent on designing and maintaining many small, specialized classes. It’s important to strike a balance between SRP and practicality, considering the project’s specific needs.
- Code duplication: One potential downside of applying the Single Responsibility Principle (SRP) is the risk of code duplication. When responsibilities are separated into distinct classes, there is a possibility of redundant code if similar or related functionalities are needed in multiple areas. So, Careful consideration and refactoring may be necessary to consolidate common code and avoid redundancy.
Summary:
The Single Responsibility Principle (SRP) is a fundamental principle in software development that emphasizes the need for classes and modules to have a single responsibility.
In C#, adhering to SRP leads to more maintainable, reusable, and readable code. By separating distinct responsibilities into separate classes, developers can achieve a modular design where each class focuses on a specific task.
This article explores the concept of SRP, providing an overview of its benefits and practical examples in C#. By following SRP, developers can improve code quality, facilitate collaboration, and ensure their code are adaptable to future changes.
FAQs
Q: What is the Single Responsibility Principle (SRP)?
The Single Responsibility Principle (SRP) is a software development principle that states that a class or module should have only one reason to change. It promotes a modular and maintainable design by separating responsibilities into individual classes.
Q: What are the challenges in applying SRP?
Applying SRP can sometimes be challenging, as it requires identifying the correct responsibility boundaries and ensuring that classes or modules are focused and cohesive. It may involve refactoring existing code and breaking down complex functionalities into separate parts.
Q: What is the difference between Singleton and Single Responsibility Principle?
The Singleton pattern and the Single Responsibility Principle (SRP) are two different concepts in software development.
The Singleton pattern ensures that a class has only one instance throughout the application. It is primarily concerned with controlling object creation, restricting access to a single instance, and providing a global access point to that instance.
On the other hand, the Single Responsibility Principle (SRP) is a design principle that emphasizes the need for classes and modules to have a single responsibility. It keeps classes focused on a specific task or functionality, promoting maintainability, reusability, and readability. SRP promotes dividing responsibilities into separate classes, each responsible for one particular task.
Q: Why SRP is important?
SRP is important because it helps make code more organized and manageable. By assigning a single responsibility to each class, it becomes easier to understand, modify, and test code.
Q: Can a class have multiple responsibilities under SRP?
No, SRP promotes the idea that a class should have a single responsibility. If a class has multiple responsibilities, separating them into distinct classes is recommended.
Recommended Articles:
- SOLID Design Principles in C#: A Complete Example
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- C# Dependency Injection
- Design Patterns
- Builder Design Pattern: A Comprehensive Guide with C# Code Examples
- Abstract Factory Design Pattern in C#
- Prototype Design Pattern
- Chain of Responsibility Design Pattern in C#
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025