is vs as operator in c#:
In C#, the IS and AS operators are essential keywords used to determine the type of an object during runtime.
The IS operator checks the type of an object and returns a boolean
value, which is true if the object is of the same type and false if not. On the other hand, the AS operator not only checks the type of an object but also performs a type conversion if the object is compatible with the given type.
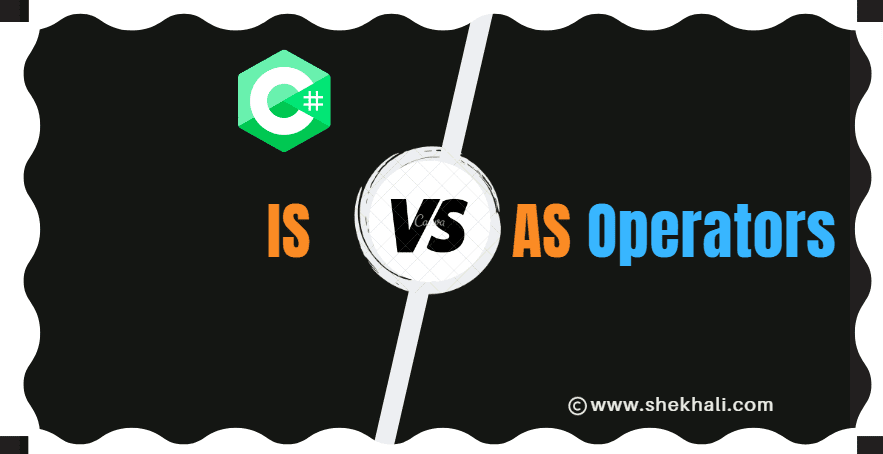
TypeCasting is a common practice in software development often used to convert one object type to another.
However, this can sometimes result in exceptions such as “Cannot implicitly convert type ‘Object one’ to ‘object two’ or InvalidCastException”.
C# provides the IS and AS operators to address this issue. It offers a more efficient and refined casting and object compatibility checking approach.
By the end of this post, you will better understand the is and as operators and the knowledge to utilize them effectively in your applications.
Table of Contents
- 1 C# IS Keyword:
- 2 AS Operator in C#
- 3 When to Use the is and as Operator in C#
- 4 Differences between IS and AS operator in C#
- 5 Example: is vs as operator in C#
- 6 Comparing IS and AS Operators in C#
- 7 Differences Between As and Is keywords in C#
- 8 Advantages of AS over IS Operator
- 9 Conclusion
- 10 FAQs
- 10.1 Q: Can the AS operator be used with value types?
- 10.2 Q: Can the IS operator be used with value types?
- 10.3 Q: What is the advantage of using the AS operator over an explicit cast operator?
- 10.4 Q: What is the advantage of using the IS Keyword in C#?
- 10.5 Q: What is the difference between the IS and AS operators in C#?
- 10.6 Related
C# IS Keyword:
The IS operator is a unary operator that determines whether an object is of a certain type. It returns a Boolean value (true or false) indicating whether the object is of the specified type or not.
Syntax
The syntax for the is operator is as follows:
object is Type
Example:
For example, Let’s consider the following code snippet to understand the is
operator:
object obj = "hello";
bool isString = obj is string; // true
The is operator is used in the above example to determine whether the object obj
is of the type string. The expression “obj is string” returns true, indicating that the object is of type string.
Here is another Example of IS operator.
using System;
namespace IsKeywordInCSharp
{
class Animal { }
class Dog : Animal { }
class Cat : Animal { }
class Program
{
static void Main(string[] args)
{
var animal = new Animal();
var dog = new Dog();
var cat = new Cat();
bool isAnimal = animal is Animal; // returns true
bool isDog = dog is Animal; // returns true
bool isCat = cat is Animal; // returns true
bool isCatDog = cat is Dog; // returns false
Console.WriteLine($" isAnimal: {isAnimal} ; isDog: {isDog} ; isCat: {isCat}; isCatDog: {isCatDog}");
Console.ReadLine();
}
}
}
The above example defines three classes: Animal, Dog, and Cat. We then create instances of each class and use the IS operator to check their types.
The first three lines of the code demonstrate that an object of each class can be identified as an instance of the Animal class.
The last line shows that an object of the type Cat cannot be identified as an instance of a Dog.
- Starting with C# 9.0, you can utilize a negation pattern for performing a non-null check using is operator, as demonstrated in the example below.
if (result is not null)
{
Console.WriteLine(result.ToString());
}
- We can use the
is
operator to check for null, as the following example shows:
if (input is null)
{
return;
}
- The
is
operator can be useful to check runtime type expressions:
using System;
public class IsOperatorExample
{
public static void Main(string[] args)
{
int i = 35;
object iBoxed = i;
int? jNullable = 45;
if (iBoxed is int a && jNullable is int b)
{
Console.WriteLine(a + b); // output 80
}
Console.ReadLine();
}
}
AS Operator in C#
The AS operator is a binary operator that performs a safe cast of an object to a specified type or returns null if the cast is not valid.
Syntax
The syntax for the as
operator is as follows:
object as Type
Example
For example, Let’s consider the following code snippet to understand the as
operator:
class Animal { }
class Dog : Animal { }
var animal = new Animal();
var dog = new Dog();
Animal animal1 = dog as Animal; // returns a valid cast
Dog dog1 = animal as Dog; // returns null
Output:
Valid cast
null
In the above example, we define two classes: Animal and Dog. We create instances of each class and use the AS operator to cast an object of type Dog to type Animal. Since Dog is a subclass of Animal, the cast is valid, and the Animal variable is assigned the dog object.
However, the second line shows that an object of type Animal cannot be cast as an instance of Dog, so the AS operator returns null.
Here is another example:
object obj = "hello";
string str = obj as string; // "hello"
In the above example, the as
operator is used to determine if the object “obj” is of string type and also performs a type conversion. The result of the expression “obj as string” is “hello”, which is the value of the object after the type conversion.
It is important to note that if the object is not of the given type then the as operator will return null. For example:
object obj = 1;
string str = obj as string; // null
When to Use the is and as Operator in C#
The is
operator is best used when you only need to check the type of an object and don’t need to perform any type conversions. The as
operator is best used when you need to check an object’s type and perform a type conversion if the object is of the specified type.
Differences between IS and AS operator in C#
The following are a few differences between the is and as keywords in C#:
Is Keyword in C# | As Keyword in C# |
---|---|
The is operator is used to determine if an object is of a certain type or not. | The AS operator determines if an object is of a certain type and performs a type conversion if the object is of the specified type. |
It returns a Boolean value(true/false) indicating whether the object is of the specified type or not. | The AS operator returns the object after the type conversion or null if the object is not of the specified type. |
Syntax: object is Type | Syntax:object as Type |
The IS operator can be best used when you only need to check the type of an object and don’t need to perform any type of conversions. | The AS operator can be best used when you need to check an object’s type and perform a type conversion if the object is of the specified type. |
The is operator is of boolean type. | However, as operator is not of boolean type. |
We can use IS operator only for reference, boxing, and unboxing conversions. | However, as operator can be used only for nullable, reference, and boxing conversions |
It’s important to note that the IS operator can be used in an if statement and As operator can be used in a try-catch statement.
Example: is vs as operator in C#
using System;
class Program
{
static void Main(string[] args)
{
// Declare an object variable
object obj = "hello";
// Check if the object is of type string using the "is" operator
if (obj is string)
{
Console.WriteLine("obj is of type string");
}
// Perform type conversion using the "as" operator
string str = obj as string;
// Check if the type conversion was successful
if (str != null)
{
Console.WriteLine($"obj as string: {str}");
}
else
{
Console.WriteLine("obj is not of type string");
}
obj = 10;
if (obj is string)
{
Console.WriteLine($"obj {obj} is of type string");
}
else
{
Console.WriteLine($"obj {obj} is not of type string");
}
str = obj as string;
if (str != null)
{
Console.WriteLine($"obj as string: {str}");
}
else
{
Console.WriteLine($"obj {obj} is not of type string");
}
Console.ReadLine();
}
}
The following output will be displayed once we run the above program:
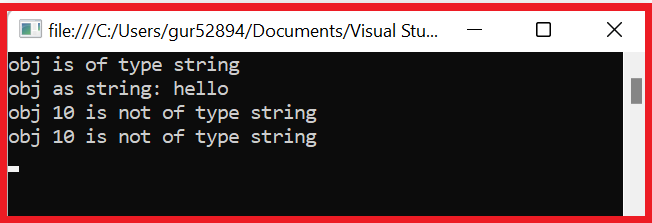
Comparing IS and AS Operators in C#
Now that we know what the IS and AS operators are and how they work let’s compare their differences.
The primary difference between the IS and AS operators is that the IS operator returns a Boolean value, while the AS operator returns either a valid cast or null. In other words, the IS operator checks if an object is of a certain type, while the AS operator checks if an object can be cast as a certain type.
Here is an example that illustrates the difference:
class Animal { }
class Dog : Animal { }
var animal = new Animal();
var dog = new Dog();
if (dog is Animal)
{
Animal animal1 = (Animal)dog;
// do something with animal1
}
Dog dog1 = animal as Dog;
if (dog1 != null)
{
// do something with dog1
}
Differences Between As and Is keywords in C#
Here are some other differences between the AS and IS operators in C#:
- We can only use the AS operator with reference types. We cannot use it with value types like int or float.
- We can use the IS operator with both reference and value types.
- The AS operator is more efficient than the IS operator since it only performs the cast once.
- The IS operator is more flexible than the AS operator since we can use it in a broader range of scenarios.
Advantages of AS over IS Operator
While both operators have their uses, there are some situations where the AS operator is preferred over the IS operator. Here are some advantages of AS over IS:
- The AS operator avoids the need for an explicit cast operator, making code more concise and readable.
- The AS operator provides a safe way to perform a cast and avoids potential runtime errors that can occur with an explicit cast operator.
- The AS operator allows for null values to be returned if the cast is not valid, avoiding the need for additional error handling code.
Conclusion
In conclusion, the IS and AS operators are essential tools in the C# developer’s toolbox. While they share some similarities, a few differences make them useful in different scenarios. The IS operator is useful for type checking, while the AS operator provides a safe way to perform a cast. Understanding these differences and use cases will allow you to write more efficient, concise, and error-free C# code.
FAQs
Q: Can the AS operator be used with value types?
No, We can only use the AS operator with reference types.
Q: Can the IS operator be used with value types?
The IS operator can be used with reference and value types.
Q: What is the advantage of using the AS operator over an explicit cast operator?
The AS operator provides a safe way to perform a cast and avoids potential runtime errors.
Q: What is the advantage of using the IS Keyword in C#?
The IS operator is useful for type checking and can be used in if statements or loops to check if an object is of a specific type.
Q: What is the difference between the IS and AS operators in C#?
The is operator is used to determine if an object is compatible with the given type and returns a Boolean value, whereas the operator is used to determine if an object is of a certain type and also performs a type conversion between compatible reference types or Nullable types.
It returns the object after the type conversion or null if the object is not of the specified type.
References-MSDN- is operator
You might also like:
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- C# Struct vs Class |Top 15+ Differences Between C# Struct and Class
- 10 Difference between interface and abstract class In C#
- C# Queue with examples
- Web API vs web service: Top 10+ Differences between web API and web service in C#
- C# Polymorphism: Different types of polymorphism in C# with examples
- C# Stack
- C# Hashtable
- C# Hashtable vs Dictionary vs HashSet
- Generic Delegates in C# With Examples
- Params Keyword in C# With Examples
- Advantages and Disadvantages of Non-Generic Collection in C#
We would love to hear your thoughts on this post. Please leave a comment below and share it with others.
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025