Overriding and Overloading the two important concepts of Polymorphism.Â
Overloading in C# is known as Static Polymorphism or Compile time Polymorphism. In Overloading we can create methods with the same name but different parameters within the same class.
On the other hand, Overriding is known as dynamic Polymorphism or Runtime Polymorphism which allows us to provide different implementation of a method in Inherited Classes.
In this article, we will learn the differences between overriding and overloading in C# with practical code examples.
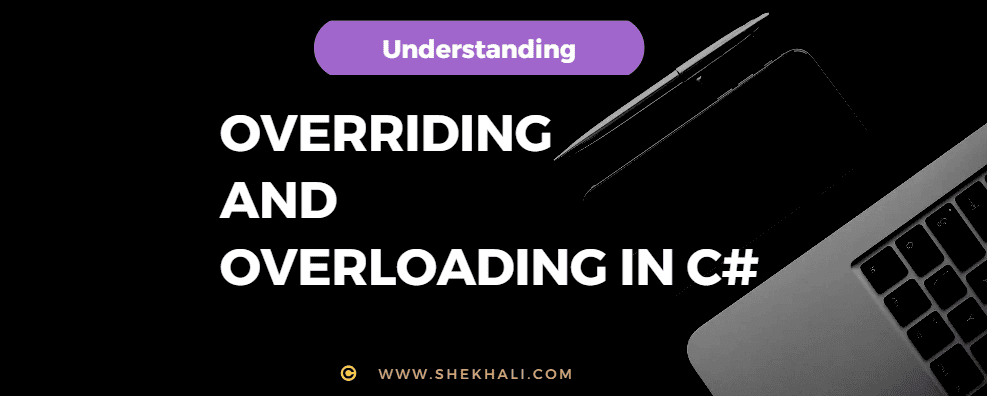
Table of Contents
What Are Overriding and Overloading?
Before we jump into the nitty-gritty details, let’s clarify what overriding and overloading actually mean in C#:
What Is Method Overloading In C#?
Overloading is a technique that allows you to define multiple methods in the same class with the same name but different parameters.
These parameters might be of different types, or they may have a different number of parameters. When you call an overloaded method, the compiler decides which one to execute based on the method’s parameters.
Overloading is also known as compile-time or static polymorphism because each of the different overloaded methods is resolved during the compilation of the application.
Example: Method Overloading:
In this code, we have a Calculator
class with three overloaded Add
methods, each methods accepting different data types.
using System;
namespace ExampleMethodOverloading
{
class Calculator
{
// Overloaded method to add two integers
public int Add(int a, int b)
{
return a + b;
}
// Overloaded method to add two doubles
public double Add(double a, double b)
{
return a + b;
}
// Overloaded method to add three floats
public float Add(float a, float b, float c)
{
return a + b + c;
}
}
class Program
{
static void Main(string[] args)
{
Calculator calculator = new Calculator();
// Using the first overloaded method (integers)
int result1 = calculator.Add(5, 10);
Console.WriteLine("Adding two integers: " + result1);
// Using the second overloaded method (doubles)
double result2 = calculator.Add(5.5, 10.5);
Console.WriteLine("Adding two doubles: " + result2);
// Using the third overloaded method (floats)
float result3 = calculator.Add(10.5f, 20.5f, 30.5f);
Console.WriteLine("Adding three floats: " + result3);
Console.ReadLine();
}
}
}
Output:
Adding two integers: 15
Adding two doubles: 16
Adding three floats: 61.5
Key Points About Method Overloading in C#
- Method overloading allows you to create multiple methods within the same class with the same name, but each method can perform a different task.
- Overloaded methods must have different parameter lists. You can achieve overloading by changing the number of parameters, using different data types, or altering the order of parameters.
- Method overloading is resolved at compile time, also known as early binding. The compiler determines which method to call based on the number and types of arguments provided when the method is invoked.
- Method overloading does not take into account the return type of the method. Two methods with the same name and the same parameter list but different return types are not considered overloaded, and they would cause a compile-time error.
What is method overriding?
Overriding is the ability to create a method in the derived class with the same signature as a method in the base class.
This means that when you override a method in a derived class, it must have the same method signature as the one in the base class. This ensures that you’re providing a specialized implementation while maintaining method compatibility.
Overriding is often referred to as runtime or dynamic polymorphism because the calling object is only known at runtime based on the specific method implementation.
Example: Method Overriding:
Let’s understand the method overriding with a simple C# code example:
using System;
class Animal
{
public virtual void MakeSound()
{
Console.WriteLine("The animal makes a generic sound.");
}
}
class Dog : Animal
{
public override void MakeSound()
{
Console.WriteLine("The dog barks.");
}
}
class Cat : Animal
{
public override void MakeSound()
{
Console.WriteLine("The cat meows.");
}
}
class Program
{
static void Main(string[] args)
{
Animal myAnimal = new Animal();
Animal myDog = new Dog();
Animal myCat = new Cat();
myAnimal.MakeSound(); // Output: The animal makes a generic sound.
myDog.MakeSound(); // Output: The dog barks.
myCat.MakeSound(); // Output: The cat meows.
}
}
Output:
The animal makes a generic sound.
The dog barks.
The cat meows.
Code Explanation:
In this code, we have a base class Animal
with a virtual method MakeSound
, which is overridden in the derived classes Dog
and Cat
.
By creating instances of these derived classes and calling the MakeSound
method, we observe how method overriding allows us to achieve polymorphic behavior, resulting in different animal sounds being produced based on the actual type of the object.
Important Key Points About Method Overriding in C#
Important key points about method overriding in C#:
- Inheritance Requirement: Method overriding is directly related to inheritance. It can only be applied to a method in a derived class that’s inherited from a base class.
- Keyword Usage: In C#, the base class method that you intend to override should be marked as
virtual
,abstract
, oroverride
. - Method Signature Preservation: When you override a method, the method name, return type, and parameter list must match exactly with the base class method. Any deviation in the method signature will result in a compile-time error.
- Runtime Polymorphism: The power of method overriding lies in its ability to achieve runtime polymorphism. When you call an overridden method on an object, the actual implementation that gets executed is determined by the type of the object at runtime, not at compile time.
- Hiding or Shadowing: Overriding is about replacing the base class method entirely, while shadowing creates a new method in the derived class with the same name, without any relation to the base class method.
- Sealed Methods: In some cases, you may want to prevent further overriding of a method. In such scenarios, you can mark the method in the derived class as
sealed
. This ensures that no further overrides are allowed. - Polymorphic Behavior: Method overriding facilitates the creation of polymorphic behavior, where you can work with objects of different derived classes through a common interface.
Overriding vs Overloading in C#
Here’ is the key differences between method overloading and method overriding in C#:
Aspect | Method Overloading | Method Overriding |
---|---|---|
Definition: | In C# Method Overloading, The methods have the same name but different types of parameters in the same class. | In C# Overriding, the methods have the same name, the same parameter types and the same number of parameters but in different classes. |
Inheritance: | Method Overriding is not directly related to inheritance. Overloaded methods exist within the same class. | Method Overriding Involves inheritance. The overridden method is in the base class, and the overriding method is in the derived class. |
Keyword Usage: | In Method overloading no specific keywords required. Methods are defined with the same name but different parameters. | In Method Overriding, The base class method should be marked as virtual or abstract , and the derived class method should be marked as override . |
Resolution: | Method Overloading resolved at compile-time (early binding) based on provided arguments to a method. | Method overriding resolved at runtime (late binding) based on the actual type of the object. |
Return Type: | Overloading doesn’t consider the return type of the method. Methods with the same name and parameter lists but different return types are not considered overloaded. | The return type of the overriding method should be compatible with the return type of the overridden method. |
Flexibility: | Method Overloading provides flexibility in terms of parameter variations (number, types, and order), making it suitable for handling different scenarios. | Method Overriding allows specialization in derived classes, enabling customized behavior while maintaining a common interface. |
Error Handling: | Compile-time error if no matching method signature is found based on the provided arguments. | No compile-time errors for method overriding as long as method signature requirements are met. |
Use Cases: | Commonly used when you want to create multiple methods with the same name to handle various inputs or scenarios efficiently. | Applied in inheritance scenarios where you want to provide distinct implementations for methods in derived classes while ensuring compatibility with the base class. |
FAQs:
Q: What is Method Overloading?
Method overloading in C# is a feature that allows you to define multiple methods with the same name in a class, each having different parameter lists.Â
Q: What is Method Overriding?
Method overriding is a concept in C# related to inheritance . It is one of the ways by which C# achieve Run Time Polymorphism or Dynamic Polymorphism.
It involves redefining a method in a derived class that already exists in the base class. This allows you to provide specialized implementations in derived classes while preserving the method name and signature.
Q: What Are the Keywords Used in Method Overloading and Method Overriding?
Method overloading does not require specific keywords. Methods are simply defined with the same name but different parameters. In method overriding, the base class method should be marked as virtual
or abstract
, and the derived class method should be marked as override
.
Q: When is Method Overloading Useful?
Method overloading is beneficial when you want to create multiple methods with the same name to handle different input scenarios efficiently.
References: Differencebetween.com , c-sharpcorner.com
Recommended Articles:
- C# Early Binding vs Late Binding
- Polymorphism in C#
- C# Struct vs Class |Top 15+ Differences Between C# Struct and Class
- Static vs Singleton in C#: Understanding the Key Differences
- Garbage Collection in C#
- SOLID Design Principles in C#: A Complete Example
- 10 Difference between interface and abstract class In C#
- Generic Delegates in C# With Examples: Func, Action, and Predicate delegates
- Local vs Global Variables
- Constructors in C#: A Comprehensive Guide with Code Examples
- C# Tutorial
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024
- How to Reverse an Array in C# with Examples - February 12, 2024