Encapsulation in C# is a fundamental principle that combines data and methods into a single unit called a class. It involves hiding the internal implementation details of the class and exposing only the essential functionalities through public properties and methods to the outside world.
It provides a clear boundary and prevents direct access to the data.
In this article, we will discuss about encapsulation in C#, including its definition, examples, benefits, and how to implement it in our code.
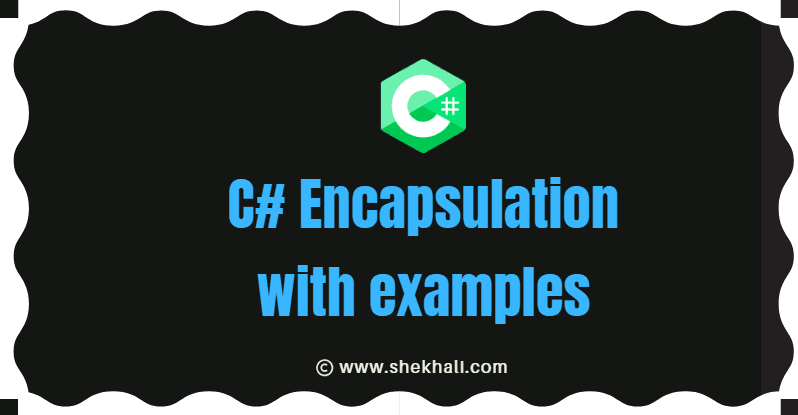
Table of Contents
- 1 What is Encapsulation in C#?
- 2 C# Encapsulation Example
- 3 Why Do We Need Encapsulation?
- 4 How to Achieve Encapsulation in C#?
- 5 What is Abstraction and Encapsulation in C#?
- 6 Difference Between Abstraction and Encapsulation in C#
- 7 Real-World Example of Encapsulation
- 8 Best Practices for Encapsulation in Specific Scenarios
- 9 How can we achieve abstraction in C#?
- 10 FAQs: C# Encapsulation
- 10.1 What is Encapsulation in C#?
- 10.2 Why is Encapsulation important in C#?
- 10.3 What is the difference between Abstraction and Encapsulation in C#?
- 10.4 How can I achieve Encapsulation in C#?
- 10.5 What are the best practices for C# Encapsulation?
- 10.6 How does encapsulation differ from abstraction in C#?
- 10.7 Related
What is Encapsulation in C#?
Encapsulation is a mechanism in OOP that binds the data and its associated behavior (methods) together in a single unit known as class. It is a technique of hiding the internal details of an object from the outside world and exposing only the necessary information or functionality to the user.
- Encapsulation provides a way to protect the data from unauthorized access or modification and ensures that the object’s state remains consistent.
- In technical terms, encapsulation ensures that the variables or data within a class are hidden from other classes, allowing access only through member functions within the same class where they are defined.
In C#, encapsulation is achieved by declaring class variables as private and providing public methods (getters and setters) to access and modify those variables.
Here is a simple code example of C# Encapsulation:
using System;
class EncapsulationExample
{
private string data; // private variable
// Public method to set the value of the private variable
public void SetData(string newData)
{
// Additional logic or validation can be added here if needed
data = newData;
}
// Public method to get the value of the private variable
public string GetData()
{
return data;
}
}
class Program
{
static void Main()
{
EncapsulationExample exampleObject = new EncapsulationExample();
// Set data using the public method
exampleObject.SetData("Hello, encapsulation!");
// Get data using the public method
string retrievedData = exampleObject.GetData();
Console.WriteLine("Retrieved Data: " + retrievedData);
}
}
Output:
Retrieved Data: Hello, encapsulation!
Code Explanation:
In this example, the data
variable is private, and its value can only be accessed or modified through the SetData
and GetData
public methods. This way, the internal details of the class are hidden, and access to the data is controlled, ensuring encapsulation.
C# Encapsulation Example
Let’s take a simple example to understand how encapsulation works in C#. Suppose you have a class named BankAccount
that contains one private field balance
and two public methods “Deposit” and “Withdraw” to manipulate the balance.
using System;
public class BankAccount
{
private decimal balance;
public void Deposit(decimal amount)
{
balance += amount;
}
public void Withdraw(decimal amount)
{
if (balance < amount)
{
Console.WriteLine("Insufficient funds.");
return;
}
balance -= amount;
}
public decimal GetBalance()
{
return balance;
}
}
class Program
{
static void Main(string[] args)
{
BankAccount account = new BankAccount();
account.Deposit(1000);
account.Withdraw(500);
decimal balance = account.GetBalance();
Console.WriteLine("Account balance is: " + balance);
}
}
In the above example, we can see that we can access the “Deposit” and “Withdraw” methods to modify the balance, but we cannot directly access the “balance” field.
We can use the GetBalance()
method to retrieve the balance information.
Output:
Account balance is: 500
Why Do We Need Encapsulation?
Encapsulation provides several benefits to C# developers, some of which are listed below:
- Security: Encapsulation in C# provides a way to protect the data from unauthorized access or modification, making the code more secure and robust.
- Modularity: Encapsulation promotes code modularity by grouping related data and behaviour into a single unit, making the code more organized and easier to maintain.
- Abstraction: Encapsulation provides a way to abstract the implementation details of a class from the outside world, making it easier to understand and use the class without worrying about its internal implementation.
- Flexibility: Encapsulation allows for changes to be made to the implementation of a class without affecting the code that uses the class. It makes the code more flexible and adaptable to changing requirements.
- Code Reusability: Encapsulation promotes code reusability by allowing the same class to be used in multiple contexts without modifying its internal implementation.
How to Achieve Encapsulation in C#?
The following are the ways we can use to achieve Encapsulation in C#:
- Access Modifiers: Access modifiers (public, private, protected, and internal) restrict the visibility of the class members from the outside world. Private members are only accessible within the same class, whereas public members are accessible from anywhere.
- Properties: Properties provide a way to encapsulate the data by providing a controlled way of accessing and modifying the class members. Properties can have getter and setter methods that enforce validation and data consistency.
- Interfaces: Interfaces provide a way to define a contract for a class without specifying its implementation details. It allows for loose coupling between classes and promotes code modularity and flexibility.
What is Abstraction and Encapsulation in C#?
Abstraction and encapsulation are two concepts in OOP that are often used together to promote code modularity and flexibility.
Abstraction is the process of hiding the implementation details of a class from the outside world and exposing only the essential features of the class. Abstraction is achieved through abstract classes and interfaces in C#.
Encapsulation, on the other hand, is the process of binding the data and its associated behaviour (methods) together in a single unit called a class. Encapsulation is achieved through access modifiers, properties, and interfaces in C#.
In summary, abstraction provides a way to define a contract for a class without specifying its implementation details, whereas encapsulation provides a way to hide the implementation details of a class from the outside world and expose only the necessary information or functionality.
Difference Between Abstraction and Encapsulation in C#
C# Abstraction | C# Encapsulation |
---|---|
Abstraction is the process of hiding the implementation details of a class from the outside world and exposing only the essential features of the class. | On the other hand, Encapsulation is the process of binding the data and its associated behaviour (methods) together in a single unit called a class. |
Abstraction is achieved through abstract classes and interfaces in C#. | Encapsulation is achieved through access modifiers, properties, and interfaces in C#. |
Abstraction provides a way to define a contract for a class without specifying its implementation details. | Encapsulation provides a way to hide the implementation details of a class from the outside world and expose only the necessary information or functionality. |
Abstraction is used to achieve loose coupling between classes and promotes code modularity and flexibility. | Encapsulation protects the data from unauthorized access or modification and ensures that the object’s state remains consistent. |
Real-World Example of Encapsulation
Here is an example of encapsulation in C# with a real-world scenario:
Let’s say we have a Car class with fields such as make, model, year, and color. We also have a method called Start() that starts the car’s engine.
To implement encapsulation, we can make the fields private and expose them through public methods or properties also called getters and setters.
For example, we can have a public method called SetMake()
that sets the value of a private field make
and a public method called GetMake()
that returns the value of the make
field.
using System;
public class Car
{
private string make;
private string model;
private int year;
private string color;
private bool isEngineOn;
public void SetMake(string make)
{
// Add validation logic here
this.make = make;
}
public string GetMake()
{
return make;
}
public void SetModel(string model)
{
// Add validation logic here
this.model = model;
}
public string GetModel()
{
return model;
}
public void SetYear(int year)
{
// Add validation logic here
this.year = year;
}
public int GetYear()
{
return year;
}
public void SetColor(string color)
{
// Add validation logic here
this.color = color;
}
public string GetColor()
{
return color;
}
public void TurnOnEngine()
{
// Add error handling logic here
isEngineOn = true;
Console.WriteLine("Engine is on.");
}
public void TurnOffEngine()
{
isEngineOn = false;
Console.WriteLine("Engine is off.");
}
}
class Program
{
static void Main(string[] args)
{
Car car = new Car();
car.SetMake("Toyota");
car.SetModel("Camry");
car.SetYear(2023);
car.SetColor("Blue");
Console.WriteLine("Car make: " + car.GetMake());
Console.WriteLine("Car model: " + car.GetModel());
Console.WriteLine("Car year: " + car.GetYear());
Console.WriteLine("Car color: " + car.GetColor());
car.TurnOnEngine();
car.TurnOffEngine();
}
}
Output:
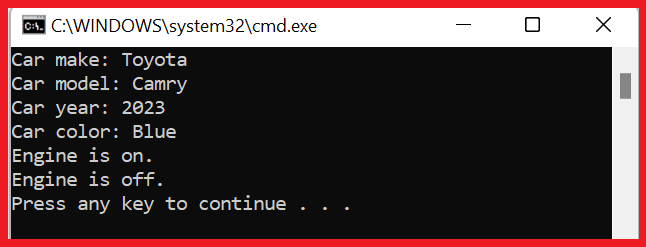
Best Practices for Encapsulation in Specific Scenarios
Encapsulation is a powerful concept in C# that promotes code modularity and maintainability. To ensure effective encapsulation in specific scenarios, consider the following best practices:
- Keep fields private and expose them through properties: Encapsulate the internal fields of a class by making them private and providing public properties to control access. It allows you to enforce validation, calculations, or additional logic when getting or setting the values.
- Use access modifiers wisely: Choose the appropriate access modifiers (private, protected, public) for fields, methods, and properties. Keep them as restricted as possible to minimize the exposure of internal implementation details.
- Avoid exposing mutable collections: When exposing collections from a class, consider using read-only interfaces (e.g.,
IReadOnlyList<T>
,IReadOnlyDictionary<TKey, TValue>)
or returning copies of the collection instead of providing direct access to the underlying mutable collection. It prevents unintended modification of the encapsulated data. - Be mindful of inheritance and polymorphism: When designing classes that are intended to be inherited, carefully consider which members to expose as protected or internal. Ensure that the encapsulated members provide the intended behavior and can be safely extended or overridden in derived classes.
- Encapsulate related functionality within cohesive classes: Group related methods and fields together in a single class to create cohesive units of functionality. It improves code organization and reduces dependencies between different parts of the system.
How can we achieve abstraction in C#?
Achieving abstraction in C# can be done through the use of abstract classes and interfaces. Here is a simple code example to help you understand:
using System;
// Abstract class
public abstract class Shape
{
public abstract void Draw(); // Abstract method
public void DisplayArea(float area)
{
Console.WriteLine("Area: " + area);
}
}
// Concrete class
public class Circle : Shape
{
public override void Draw()
{
Console.WriteLine("Drawing a circle");
}
}
// Concrete class
public class Rectangle : Shape
{
public override void Draw()
{
Console.WriteLine("Drawing a rectangle");
}
}
class Program
{
static void Main(string[] args)
{
Shape circle = new Circle();
circle.Draw(); // Output: Drawing a circle
circle.DisplayArea(25.5f); // Output: Area: 25.5
Shape rectangle = new Rectangle();
rectangle.Draw(); // Output: Drawing a rectangle
rectangle.DisplayArea(50.2f); // Output: Area: 50.2
}
}
Code Explanation:
In this example, we have an abstract class called Shape, which defines an abstract method, Draw()
, and a non-abstract method DisplayArea()
, that displays the area. The Draw() method is left abstract because each Shape will have its own drawing implementation.
The concrete classes Circle and Rectangle inherit from the Shape class and provide their own implementations of the Draw() method. They also inherit the DisplayArea() method.
In the Main()
method, we create instances of Circle and Rectangle and call their Draw() and DisplayArea() methods. Since the abstract class Shape provides a common interface, we can treat the objects of both classes as Shape objects, allowing for abstraction.
By using abstract classes and interfaces, we can achieve abstraction in C# by focusing on the essential features and behavior of objects while hiding the implementation details.
References: MSDN-OOPs
FAQs: C# Encapsulation
What is Encapsulation in C#?
Encapsulation in C# is a fundamental object-oriented programming (OO) concept that involves bundling the data (variables) and methods (functions) that operate on that data into a single unit, known as a class.
The key aspect of encapsulation is the restriction of access to the internal details of the class, allowing interaction with the data only through well-defined public methods (getters and setters).
In C#, encapsulation is typically achieved by declaring class variables as private, preventing direct access from outside the class.
Public methods are then provided to manipulate or retrieve the private data.
This approach enhances code modularity, security, and maintainability, as it shields the internal implementation details of a class and controls how external code can interact with the encapsulated data.
Why is Encapsulation important in C#?
Encapsulation is important in C# because it promotes code modularity, maintainability, and data integrity. It allows you to hide implementation details, control access to class members, and ensure consistent behavior throughout the application.
What is the difference between Abstraction and Encapsulation in C#?
In C#, abstraction focuses on hiding complex implementation details and highlighting essential features, allowing developers to work at a higher level of understanding. It’s achieved through interfaces, abstract classes, and inheritance.
Encapsulation, on the other hand, is about bundling data and methods into a single unit, typically a class, and hiding an object’s internal implementation details and only exposing necessary functionalities through well-defined interfaces, enhancing code maintainability, reusability, and security.
How can I achieve Encapsulation in C#?
In C#, We can achieve encapsulation by declaring class variables as private and providing public methods (getters and setters) to access and modify those variables.
What are the best practices for C# Encapsulation?
Some best practices for encapsulation in C# include keeping fields private and exposing them through properties, using access modifiers wisely, avoiding exposing mutable collections directly, being mindful of inheritance and polymorphism, and encapsulating related functionality within cohesive classes.
How does encapsulation differ from abstraction in C#?
Encapsulation and abstraction are two important concepts in C# programming. Here is a simple explanation:
Encapsulation is like putting your code in a box and controlling who can access and modify it. It allows you to hide the internal details of a class and only expose what’s necessary. It helps maintain the integrity of your code and prevents unintended changes from outside.
Abstraction, on the other hand, is about simplifying complex things. It’s like using a TV remote without worrying about the inner workings of the TV. In C#, abstraction allows you to focus on the essential features of an object or concept while ignoring the unnecessary details.
Recommended Articles:
- Abstraction Vs. Encapsulation in C#
- Object-oriented programming in C# with examples
- C# String Is Null or Empty
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- Understanding the Chain of Responsibility Design Pattern in C#
- Difference between interface and abstract class In C#
- C# Interface – Understanding Interfaces in C# (With Examples)
- C++ vs C#: Difference Between C# and C++
- C# Regex: Introduction to Regular Expressions in C# with Examples
- C# String Interpolation – Syntax, Examples, and Performance
- C# Stopwatch Explained – How to Measure Code Execution Time?
- C# String Vs StringBuilder
- C# Queue with examples
- Web API vs web service: Top 10+ Differences between web API and web service in C#
- C# Polymorphism: Different types of polymorphism in C# with examples
- What is a Full Stack Developer: Steps to become full stack developer in 2023
Please share this post and let us know what you think in the comments.
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024