Table of Contents
- 1 Introduction
- 2 What is a Copy Constructor in C#?
- 3 Syntax
- 4 Benefits of Using Copy Constructors:
- 5 How to create a copy constructor in C#?
- 6 How to write a copy constructor using ICloneable Interface?
- 7 Rules for copy constructors in C#
- 8 Types of constructors in C#
- 9 FAQs:
- 9.1 Q: What is the purpose of a copy constructor in C#?
- 9.2 Q: Can a copy constructor have a different name than the class or structure?
- 9.3 Q: Can a copy constructor return a value?
- 9.4 Q: Can a copy constructor be inherited in C#?
- 9.5 Q: Is it possible to declare a copy constructor as static?
- 9.6 Q: Can I modify the values of the copy without affecting the original object?
- 9.7 Q: Why Use a Copy Constructor?
- 9.8 Q: Why Use a Copy Constructor?
- 10 Conclusion:
Introduction
In object-oriented programming, a copy constructor plays a crucial role in creating a new object by copying the variables from an existing object.
Copy constructor allows us to initialize a fresh instance with the values of an already existing instance. Although C# doesn’t inherently provide a copy constructor, we can create one according to our specific requirements.
In this article, we’ll try to understand the concept of Copy constructors in C# with examples.
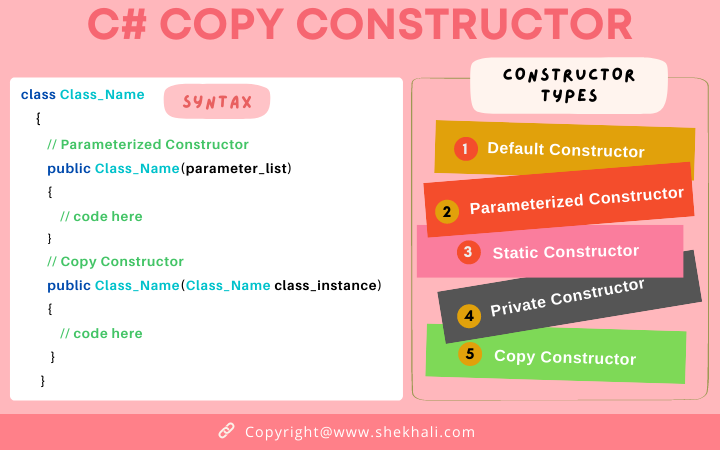
What is a Copy Constructor in C#?
A copy constructor in C# is a parameterized constructor that takes an instance of the same class as its argument. Its primary purpose is to create a new object by copying the data from an existing object.
It enables the creation of an independent object with the same values as the original, which can be modified without affecting the original object.
Let’s dive into the syntax and usage of a copy constructor.
Syntax
In C#, a copy constructor is declared using the class name followed by the constructor keyword, taking an instance of the same class as a parameter.
class MyClass
{
// Copy Constructor
public MyClass(MyClass original)
{
// Assign values from 'original' to the current object
}
}
In the above syntax, we are passing the same class type, ‘MyClass’ as a parameter in the copy constructor. This will create a new object with the same values as the existing object.
Benefits of Using Copy Constructors:
- Object Cloning: Copy constructors allow us to clone objects easily, ensuring independent copies with identical values.
- Immutable Objects: Copy constructors are useful when working with immutable objects, where you want to create new instances with the same state.
- Class Composition: When a class consists of other objects, a copy constructor ensures that all the composed objects are copied correctly.
How to create a copy constructor in C#?
To create a copy constructor, follow these steps:
- Define a class with a parameterized constructor (the instance constructor).
- Add another constructor that takes an instance of the same class as its argument (the copy constructor).
using System;
namespace CopyConstructor
{
// C# program demonstrating how to use the copy constructor
// Class
public class User
{
// Instance variables
public int Id;
public string Name;
// Parameterized Constructor (or Instance constructor)
public User(int id, string name)
{
this.Id = id;
this.Name = name;
}
// Copy Constructor
public User(User previousUser)
{
this.Id = previousUser.Id;
this.Name = previousUser.Name;
}
// Print user details
public void PrintDetails()
{
Console.WriteLine($"Id: {Id} Name: {Name}");
}
}
class Program
{
static void Main(string[] args)
{
// This will invoke the instance constructor
User user1 = new User(10, "Shekh Ali");
// This will invoke the copy constructor
// Creating another User object by copying user1
User user2 = new User(user1);
// Change user2 Id and Name
user2.Id = 20;
user2.Name = "Klara";
// Print details to verify that the
// Id and Name fields are unique.
user1.PrintDetails();
user2.PrintDetails();
Console.ReadLine();
}
}
}
/* Output:
Id: 10 Name: Shekh Ali
Id: 20 Name: Klara
*/
Output:
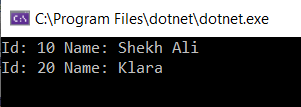
Here, we create a new user2
instance after passing the User1
object as a parameter in the copy constructor.
- All instance field values of the object
User1
have been copied to the new objectuser2
. - When we assign the new values in the object ‘user2’, it will not affect the existing object ‘user1’ values.
How to write a copy constructor using ICloneable Interface?
In the above program, we are using a copy constructor to clone one object to another, We can do the same thing by using the ICloneable
Interface.
Below is a simple example of cloning by using ICloneable Interface in C#.
using System;
namespace ShallowCopy
{
// C# program demonstrating how to use the copy constructor
// User Class implements ICloneable Interface
public class User: ICloneable
{
//Instance variables
public int Id;
public string Name;
// Parameterized Constructor
public User(int id, string name)
{
this.Id = id;
this.Name = name;
}
//Using Shallow Copy
public object Clone()
{
return this.MemberwiseClone();
}
//Print person details
public void PrintDetail()
{
Console.WriteLine($"Id: {Id} Name: {Name}");
}
}
class Program
{
static void Main(string[] args)
{
// This will invoke instance constructor
User user1 = new User(10,"Shekh Ali");
// Performing shallow copy of 'user1' and assign it to 'user2'.
User user2 = (User)user1.Clone();
// Change user2 Id and Name
user2.Id = 20;
user2.Name = "Klara";
// Print details to verify that the
// Id and Name fields are unique.
user1.PrintDetail();
user2.PrintDetail();
Console.ReadLine();
}
}
/* Output:
Id: 10 Name: Shekh Ali
Id: 20 Name: Klara
*/
}
In C#, The ICloneable
interface has a single method called Clone()
to create a copy of the existing object.
Here, we are using the MemberwiseClone()
method of the object class in the Clone
method.
The MemberwiseClone()
method creates a shallow copy by creating a new object and copying the non-static fields from the current object to the new object.
Rules for copy constructors in C#
- Purpose: The primary purpose of a copy constructor is to initialize a new instance of a class or structure with the values of an existing instance. It creates an independent copy that can be modified without affecting the original object.
- Name: The copy constructor in C# must have the same name as the class or structure it belongs to.
- Return Type: Unlike regular constructors, the copy constructor does not have a return type, not even
void
. It is solely responsible for initializing the new instance. - Inheritance: Copy constructors cannot be inherited in C#. If needed, each class or structure must define its own copy constructor.
- Static: Copy constructors cannot be declared as static. They are instance constructors and operate on specific objects.
Types of constructors in C#
There are five different types of constructors in C#.
- Default Constructor
- Parameterized Constructor
- Copy Constructor
- Static Constructor
- Private Constructor
FAQs:
Here are some frequently asked questions (FAQs)
Q: What is the purpose of a copy constructor in C#?
The main purpose of a copy constructor is to create a new instance of a class or structure with the same values as an existing instance.
It allows for the creation of independent copies that can be modified without affecting the original object.
Q: Can a copy constructor have a different name than the class or structure?
No, in C#, the copy constructor must have the same name as the class or structure it belongs to.
Q: Can a copy constructor return a value?
No, a copy constructor in C# has no return type, including “void.” Its purpose is to initialize the new instance, not return a value.
Q: Can a copy constructor be inherited in C#?
No, copy constructors cannot be inherited in C#. Each class or structure must define its own copy constructor if needed.
Q: Is it possible to declare a copy constructor as static?
No, copy constructors cannot be declared as static. They are instance constructors and operate on specific objects.
Q: Can I modify the values of the copy without affecting the original object?
Yes, the copy constructor creates an independent copy, allowing you to modify the values of the copy without affecting the original object.
Q: Why Use a Copy Constructor?
The main use of a copy constructor is to initialize a new instance with the values of an existing instance. It’s particularly handy when dealing with complex objects or when you want to create a deep copy of an object.
Q: Why Use a Copy Constructor?
The main use of a copy constructor is to initialize a new instance with the values of an existing instance. It’s particularly handy when dealing with complex objects or when you want to create a deep copy of an object.
Conclusion:
In conclusion, understanding copy constructors in C# is important for creating independent copies of objects.
This article has provided a comprehensive guide on copy constructors, explaining their purpose, rules, and usage with code examples.
By implementing copy constructors, you can easily create new instances with the same values as existing objects.
Recommended Articles:
- C# Tutorial
- C# Programs asked in Interviews
- Constructors in C# with Examples
- Array Vs List in C#
- Difference between var and dynamic in C#
- Generic Delegates in C# With Examples
- IEnumerable Interface in C# with examples
- Properties In C# with examples
- 10 Difference between interface and abstract class In C#
- Value Type and Reference Type in C#
- C# Dictionary with Examples
- C# Struct vs Class
- C# Hashtable vs Dictionary
- C# Events: Differences between delegates and events in C#
- Difference between Boxing and Unboxing in C#
We hope you find this article informative and helpful. If you have any questions, feel free to ask them in the comments section below.
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024