TRY CASTÂ function in SQL belongs to the Conversions category of functions and resembles the CAST() function. Its purpose is to transform an expression from one data type to another. If successful, the result will be the expression in the expected data type, but if it fails, the function will return null.
This blog post will dive into the TRY_CAST()
function in SQL Server, its syntax, and how it differs from the CAST()
function. We will use a few code examples to make it easy to implement this function in our SQL Server database.
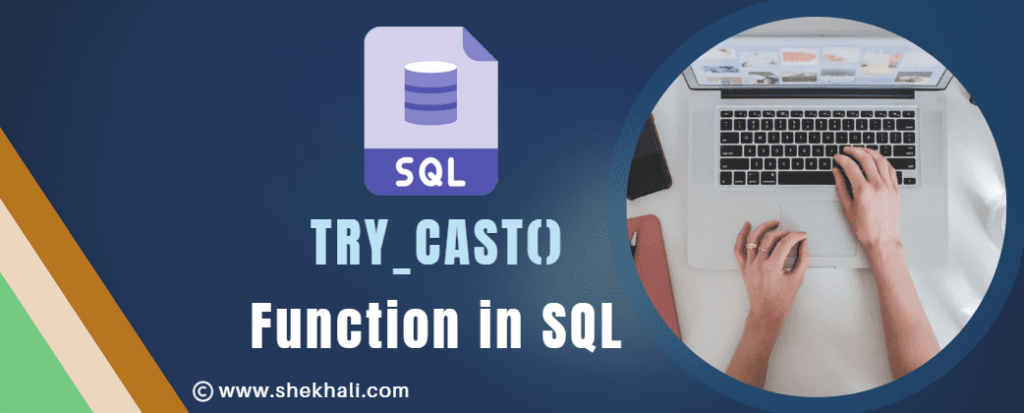
Table of Contents
- 1 Understanding the TRY_CAST() Function in SQL Server
- 2 Syntax of the TRY_CAST() Function
- 3 The Difference Between CAST() and TRY_CAST()
- 4 Example: TRY_CAST() Function
- 4.1 Example 1: Try to cast a string to an integer
- 4.2 Example 2: Try to cast a string to a date
- 4.3 Example 3: Try to cast a string to a float
- 4.4 Example 4: Try to cast a string to an integer but with a value that cannot converted
- 4.5 Example 5: Try to cast a string to date but with a value that cannot be converted
- 4.6 Example 6: TRY_CAST returns null
- 5 Summary:
- 6 FAQs
- 6.1 Q: What is the SQL Server TRY_CAST() function?
- 6.2 Q: What are some examples of how to use the TRY_CAST() function in SQL Server?
- 6.3 Q: What are the key differences between the CAST() and TRY_CAST() functions in SQL Server?
- 6.4 Q: How can you use the TRY_CAST() function to optimize SQL Server queries?
- 6.5 Related
Understanding the TRY_CAST() Function in SQL Server
The TRY_CAST() function in SQL Server is used to convert an expression of one data type to another data type. This function returns null if the conversion fails, which is a significant advantage over the CAST() function.
Syntax of the TRY_CAST() Function
The syntax of the TRY_CAST() function is straightforward.
TRY_CAST(expression AS data_type)
The expression is the value you want to convert, and the data_type is the data type to which you want to convert it.
The Difference Between CAST() and TRY_CAST()
The CAST() and TRY_CAST() functions in SQL Server have much in common. They are both used to convert an expression of one data type to another. However, the TRY_CAST() function has advantages over the CAST() function. The following are few differences between the two functions:
- The TRY_CAST() function returns null if the conversion fails, while the CAST() function returns an error.
- The TRY_CAST() function is more flexible than the CAST() function. It can handle a broader range of data types, and it can also handle null values.
- The TRY_CAST() function is slower than the CAST() function. That is because it has to check for null values and perform additional checks to ensure that the conversion is successful.
Example: TRY_CAST() Function
Now that we understand the TRY_CAST() function’s basics let’s look at some examples.
Example 1: Try to cast a string to an integer
SELECT TRY_CAST('123' AS INT);
In this example, the TRY_CAST() function tries to cast the string ‘123’ to an integer. Since we can convert this string to an integer, the output will be 123.
Example 2: Try to cast a string to a date
SELECT TRY_CAST('2021-08-20' AS DATE);
In this example, the TRY_CAST() function tries to cast the string ‘2021-08-20’ to a date. Since this string is in the correct date format, the output will be 2021-08-20.
Example 3: Try to cast a string to a float
SELECT TRY_CAST('12.34' AS FLOAT);
In this example, the TRY_CAST() function tries to cast the string ‘12.34’ to a float. Since we can convert this string to a float, the output will be 12.34.
Example 4: Try to cast a string to an integer but with a value that cannot converted
SELECT TRY_CAST('abc' AS INT);
In this example, the TRY_CAST() function tries to cast the string ‘abc’ to an integer. Since we cannot convert this string to an integer, the output will be NULL.
Example 5: Try to cast a string to date but with a value that cannot be converted
SELECT TRY_CAST('2021-08-20 13:00:00' AS DATE);
In this example, the TRY_CAST() function tries to cast the string ‘2021-08-20 13:00:00’ to a date. Since this string is not in the correct date format, the output will be NULL.
Example 6: TRY_CAST returns null
The following example will demonstrate that TRY_CAST() function returns null when the cast fails.
SELECT
CASE WHEN TRY_CAST('mytext' AS float) IS NULL
THEN 'Cast failed'
ELSE 'Cast succeeded'
END AS Result;
The above SQL query will return the following result set.
Result
-----------
Cast failed
These examples demonstrate how the TRY_CAST() function can safely convert data types in SQL Server, even when the input data is not in the expected format.
Summary:
In this post, we explored the SQL Server TRY_CAST()
function and its usage. TRY_CAST() is a built-in function in SQL Server that is used to convert an expression of one data type to another safely.
It is similar to the CAST()
function. Still, it has the added benefit of returning NULL if the cast fails rather than throwing an error.
We also discussed the key differences between the CAST()
and TRY_CAST()
functions and how we can use them to optimize SQL Server queries. The post includes examples of TRY_CAST() in action, explanations, and code snippets. Using the TRY_CAST() function, we can ensure that data is converted safely without causing errors or unexpected results.
FAQs
The following are frequently asked questions and answers about the TRY_CAST() Function in SQL Server.
Q: What is the SQL Server TRY_CAST() function?
The TRY_CAST() function is a built-in function in SQL Server that can safely convert an expression of one data type to another. It is similar to the CAST() function but with the added benefit of returning NULL if the cast fails rather than throwing an error.
Q: What are some examples of how to use the TRY_CAST() function in SQL Server?
Here are some examples of how to use the TRY_CAST() function in SQL Server:
SELECT TRY_CAST(‘123’ AS INT); — Output: 123
SELECT TRY_CAST(‘2021-08-20’ AS DATE); — Output: 2021-08-20
SELECT TRY_CAST(‘12.34’ AS FLOAT); — Output: 12.34
SELECT TRY_CAST(‘100.34’ AS INT); — Output: 100
SELECT TRY_CAST(‘abc’ AS INT); — Output: NULL
SELECT TRY_CAST(‘2021-08-20 13:00:00’ AS DATE); — Output: NULL
Q: What are the key differences between the CAST() and TRY_CAST() functions in SQL Server?
The main difference between the two functions is that the CAST()
function will throw an error if the cast fails, while the TRY_CAST()
function will return NULL. That means that the TRY_CAST()
function can safely convert data types without worrying about errors that could cause a query to fail.
Q: How can you use the TRY_CAST() function to optimize SQL Server queries?
By using the TRY_CAST() function in your SQL Server queries, you can ensure that data is converted safely without causing errors or unexpected results. That can help improve your queries’ performance and reliability and make it easier to work with data of different types.
References: MSDN-TRY_CAST() IN SQL
You might want to read this too:
- Having VS Where Clause in SQL
- Types of Joins in SQL Server
- Function vs Stored procedure in SQL Server
- Stored Procedure in SQL Server With Examples
- Create, Alter, and Drop Database In SQL Server
- SQL Comparison Operators (Less than, Greater than, Equal, Not Equal operators)
- Primary Key Constraint In SQL Server
- View In SQL | Types Of Views In SQL Server
- SQL Server Trigger Update, Insert, Delete Examples
- SQL pivot tables
- Different ways to delete duplicate rows in SQL Server
- UNION vs UNION ALL in SQL SERVER
- SQL Server Try Catch: Error Handling in SQL Server With [Examples]
- Group by Month in SQL
Let others know about this post by sharing it and leaving your thoughts in the comments section.
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025