In this post, We will learn about the SortedList and SortedDictionary in C# using some code examples. Furthermore, we will compare these two collections in a tabular format which will help us to understand the fundamental difference between SortedList and SortedDictionary in C#.
By the end of this post, you should have a good understanding of when to use SortedList and when to use SortedDictionary in your code.
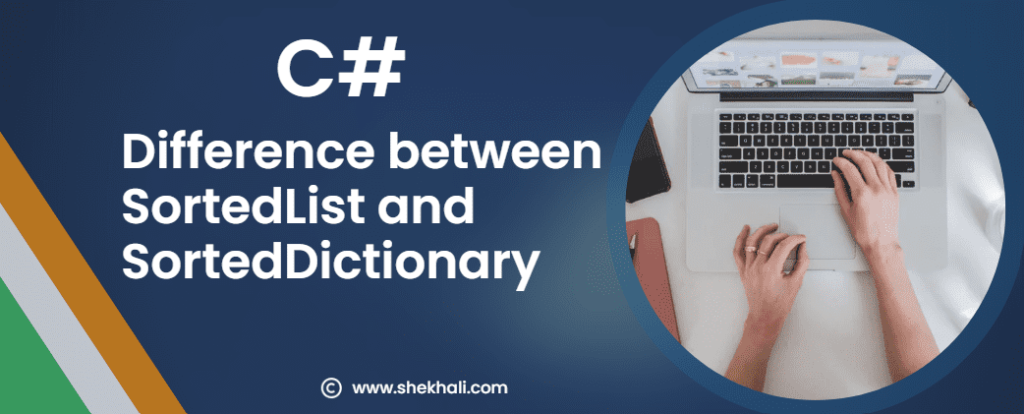
Table of Contents
- 1 What is SortedList in C#?
- 2 What is SortedDictionary in C#?
- 3 Key Differences between SortedList and SortedDictionary in C#
- 4 When to use SortedList in C#?
- 5 When to use SortedDictionary in C#?
- 6 FAQ:
- 6.1 Q: What are SortedList and SortedDictionary in C#?
- 6.2 Q: What is the difference between SortedList and SortedDictionary in C#?
- 6.3 Q: When should you use SortedList and when should you use SortedDictionary in C#?
- 6.4 Q: How do you check if a key is present in SortedList and SortedDictionary in C#?
- 6.5 Q: How do you iterate through SortedList and SortedDictionary in C#?
- 6.6 Related
What is SortedList in C#?
In C#, SortedList<TKey,TValue> is a collection of key/value pairs that are sorted according to unique keys. By default, this collection sorts the key/value pairs in ascending order. It is available in both generic and non-generic types of collections.
The generic version of SortedList can be found in the System.Collections.Generic
namespace while the non-generic SortedList is available in the System.Collections
namespace.
Syntax:
SortedList<TKey, TValue> variableName = new SortedList<TKey, TValue>();
Example: Creating a SortedList in C#
The following is an example of the generic SortedList in C#.
using System;
using System.Collections.Generic;
namespace SortedListExample
{
class Program
{
static void Main(string[] args)
{
// Create a new SortedList
SortedList<string, int> sortedList = new SortedList<string, int>();
// Add key-value pairs to the SortedList
sortedList.Add("Shekh", 29);
sortedList.Add("Mary", 30);
sortedList.Add("Jim", 35);
// Access the values in the SortedList
Console.WriteLine("Shekh's age: " + sortedList["Shekh"]);
Console.WriteLine("Mary's age: " + sortedList["Mary"]);
Console.WriteLine("Jim's age: " + sortedList["Jim"]);
// Remove a key-value pair from the SortedList
sortedList.Remove("Mary");
// Check if a key is present in the SortedList
if (sortedList.ContainsKey("Mary"))
{
Console.WriteLine("Mary's age: " + sortedList["Mary"]);
}
else
{
Console.WriteLine("Mary is not present in the SortedList");
}
// Iterate through the SortedList
foreach (KeyValuePair<string, int> item in sortedList)
{
Console.WriteLine(item.Key + "'s age: " + item.Value);
}
// Clear the SortedList
sortedList.Clear();
// Check if the SortedList is empty
if (sortedList.Count == 0)
{
Console.WriteLine("The SortedList is empty");
}
Console.ReadLine();
}
}
}
Output:
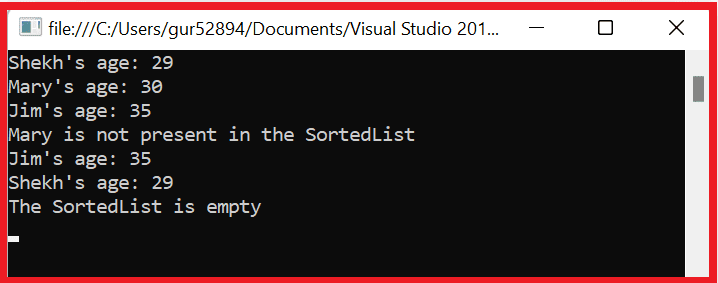
What is SortedDictionary in C#?
In C#, SortedDictionary<TKey,TValue> class represents a collection of key/value pairs sorted by key and is available in the System.Collections.Generic
namespace. Like SortedList, it stores key-value pairs, where each key must be unique and the data is sorted based on the keys.
Syntax:
SortedDictionary<TKey, TValue> variableName = new SortedDictionary<TKey, TValue>();
Example: Creating a SortedDictionary in C#
The following code example will show you how to create a generic SortedDictionary collection in C#:
using System;
using System.Collections.Generic;
namespace SortedDictionaryExample
{
class Program
{
static void Main(string[] args)
{
// Create a new SortedDictionary
SortedDictionary<int, string> sortedDictionary = new SortedDictionary<int, string>();
// Add key-value pairs to the SortedDictionary
sortedDictionary.Add(1, "Shekh Ali");
sortedDictionary.Add(2, "Deepak");
sortedDictionary.Add(3, "Jim");
// Access the values in the SortedDictionary
Console.WriteLine("Name: " + sortedDictionary[1]);
Console.WriteLine("Name: " + sortedDictionary[2]);
Console.WriteLine("Name: " + sortedDictionary[3]);
// Remove a key-value pair from the SortedDictionary
sortedDictionary.Remove(3);
// Check if a key is present in the SortedDictionary
if (sortedDictionary.ContainsKey(3))
{
Console.WriteLine("Jim's Id: " + sortedDictionary[3]);
}
else
{
Console.WriteLine("Jim is not present in the SortedDictionary");
}
// Iterate through the SortedDictionary
foreach (KeyValuePair<int, string> item in sortedDictionary)
{
Console.WriteLine($" Id :{item.Key} Name: {item.Value}");
}
// Clear the SortedDictionary
sortedDictionary.Clear();
// Check if the SortedDictionary is empty
if (sortedDictionary.Count == 0)
{
Console.WriteLine("The SortedDictionary is empty");
}
Console.ReadLine();
}
}
}
Output:
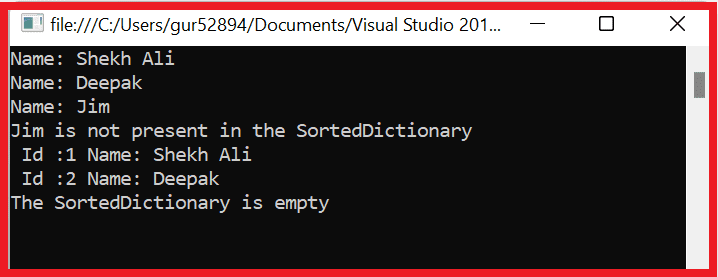
Key Differences between SortedList and SortedDictionary in C#
Here are some of the key differences between SortedList and SortedDictionary in C#:
Feature | SortedList | SortedDictionary |
---|---|---|
Performance: | SortedList is faster for data retrieval but slower for inserting new items compared to SortedDictionary. | SortedDictionary is faster for inserting new items, but slower for lookups compared to SortedList. |
Memory usage: | SortedList uses more memory than SortedDictionary. | SortedDictionary uses less memory than SortedList |
Access elements: | In a SortedList , elements can be retrieved through an index. | However, in a SortedDictionary , elements can be retrieved through the index or a key. The key-based method of accessing elements is sufficient, making the use of the index redundant. |
Sorting: | In SortedList, the data is by default arranged in sorted order. | On the other hand, the data in SortedDictionary is not arranged in any specific order and needs to be sorted before it can be used. |
Data type: | SortedList supports non-generic and generic data types | SortedDictionary only supports generic data types |
When to use SortedList in C#?
SortedList is a good choice when:
- You need to store data in a sorted manner and want to retrieve data quickly.
- You are working with non-generic data types.
- You have a limited amount of items to store and don’t mind using more memory
When to use SortedDictionary in C#?
SortedDictionary is a good choice when:
- You need to store data in a sorted manner and perform insertions quickly.
- You are working with generic data types.
- You have a large number of items and want to minimize memory usage.
FAQ:
The following are some frequently asked questions about the “Difference between SortedList and SortedDictionary in C#”:
Q: What are SortedList and SortedDictionary in C#?
SortedList and SortedDictionary are both collection classes in C# that allow you to store key-value pairs in a sorted manner and don’t allow duplicate keys.
Q: What is the difference between SortedList and SortedDictionary in C#?
A SortedList
is a collection that allows you to retrieve the key-value data using indexes very quickly. A SortedDictionary, on the other hand, allows you to retrieve data using either keys or indexes and offers faster insertion and removal of unsorted data than a SortedList. A SortedList can retrieve data faster than SortedDictionary but it consumes more memory than the SortedDictionary.
Q: When should you use SortedList and when should you use SortedDictionary in C#?
You can use SortedList If you need to access values using an index. SortedDictionary is a good choice if you want to prioritize performance and memory usage.
Q: How do you check if a key is present in SortedList and SortedDictionary in C#?
You can check if a key is present in SortedList and SortedDictionary using the ContainsKey method.
Q: How do you iterate through SortedList and SortedDictionary in C#?
You can iterate through SortedList and SortedDictionary using a foreach
loop and access the key-value pairs using the KeyValuePair
structure.
References: MSDN-Sorted Collection, SortedDictionary
You might also like:
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- Is vs As operator in C#
- C# Struct vs Class |Top 15+ Differences Between C# Struct and Class
- 10 Difference between interface and abstract class In C#
- C# Queue with examples
- Web API vs web service: Top 10+ Differences between web API and web service in C#
- C# Polymorphism: Different types of polymorphism in C# with examples
- C# Stack
- C# Hashtable
- C# Hashtable vs Dictionary vs HashSet
- Generic Delegates in C# With Examples
- Params Keyword in C# With Examples
- Jump Statements in C# (Break, Continue, Goto, Return, and Throw)
- Understanding the Difference between int, Int16, Int32, and Int64 in C#
We would love to hear your thoughts on this post. Please leave a comment below and share it with others.
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025