C# provides several data types to store numerical values, and choosing the right one is important. In this article, we will try to understand the difference between int, Int16, Int32, and Int64 in C#.
You will learn about the size, minimum, and maximum values for each data type and when to use them appropriately.
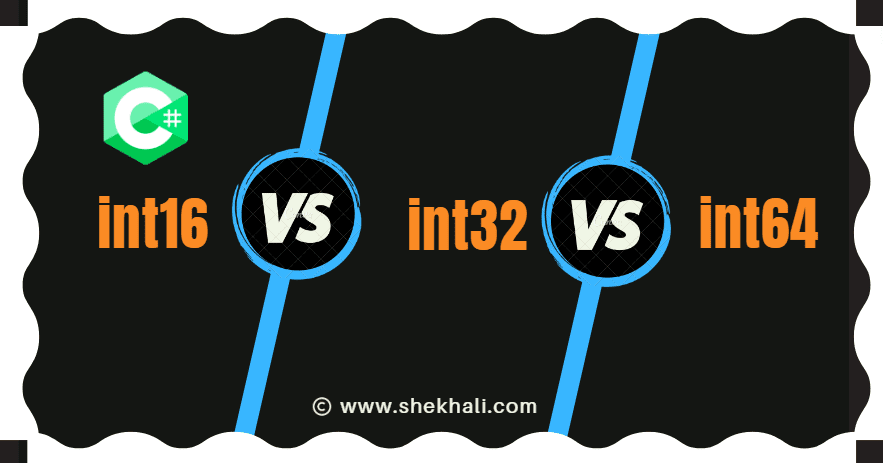
Table of Contents
- 1 What is int in C#?
- 2 What is Int16 in C#?
- 3 What is Int32 in C#?
- 4 What is Int64 in C#?
- 5 int vs Int16 vs Int32 vs Int64 in C#
- 6 Properties and Methods of Int16, Int32, and Int64 in C#
- 7 Conclusion
- 8 FAQs
- 8.1 Q: What is the difference between int, Int16, Int32, and Int64 in C#?
- 8.2 Q: What is the range of values that can be stored in ‘int’?
- 8.3 Q: What is the range of values that can be stored in Int16?
- 8.4 Q: What is the range of values that can be stored in Int32?
- 8.5 Q: What is the range of values that can be stored in Int64?
- 8.6 Q: Is ‘int’ an alias for Int32?
- 8.7 Q: Can Int16 store negative values?
- 8.8 Q: Can Int64 store larger values than Int32?
- 8.9 Q: What is the difference between int and Int32?
- 8.10 Q: When should I use Int16 instead of int?
- 8.11 Q: Why would I use Int64 instead of int?
- 8.12 Q: Are there performance differences between int, Int32, and Int64?
- 8.13 Q: What happens if I exceed the limits of int or Int32?
- 8.14 Q: Can I convert int to Int64?
- 8.15 Related
What is int in C#?
In C#, int is a keyword that represents a 32-bit signed integer. It is an alias for the Int32 structure in the .NET Framework. The int type can store values ranging from -2,147,483,648 to 2,147,483,647.
- The int data type is a 32-bit signed integer
- It is the default data type for integral values in C#
- Int can store values ranging from -2,147,483,648 to 2,147,483,647
- The keyword ‘int’ is an alias for the
System.Int32
data type. So, int and int32 are the same data types.
Example of int in C#
using System;
namespace ExampleInt
{
class Program
{
static void Main(string[] args)
{
// Displaying information about int data type
Console.WriteLine("Int occupies {0} bytes", sizeof(int));
Console.WriteLine("Int belongs to the {0} type", typeof(int));
Console.WriteLine("Int MIN value: {0}", int.MinValue);
Console.WriteLine("Int MAX value: {0}", int.MaxValue);
Console.WriteLine();
// int variables
int a = -2147483648;
int b = 2147483647;
Console.WriteLine($"a = {a}, b = {b}");
Console.ReadLine();
}
}
}
Output:
Int occupies 4 bytes
Int belongs to the System.Int32 type
Int MIN value: -2147483648
Int MAX value: 2147483647
a = -2147483648, b = 2147483647
What is Int16 in C#?
 is a structure in the .NET Framework that represents a 16-bit signed integer. It is part of theÂ
Int16System
 namespace. The Int16
 type can store values ranging from -32,768 to 32,767. It is useful when you need to save memory and the range of values fits within the limits of a 16-bit integer.
- The Int16 data type is a 16-bit signed integer
- Int16 can store values ranging from -32,768 to 32,767
- The keyword ‘Int16’ is an alias for the
System.Int16
data type
Example of Int16 in C#
using System;
using System.Text;
namespace ExampleInt16
{
class Program
{
static void Main(string[] args)
{
// Displaying information about Int16
Console.WriteLine("Int16 occupies {0} bytes", sizeof(Int16));
Console.WriteLine("Int16 belongs to the {0} type", typeof(Int16));
Console.WriteLine("Int16 MIN value: {0}", Int16.MinValue);
Console.WriteLine("Int16 MAX value: {0}", Int16.MaxValue);
Console.WriteLine();
// Int16 variables
Int16 a = -32768;
Int16 b = 32767;
Console.WriteLine($"a = {a}, b = {b}");
Console.ReadLine();
}
}
}
Output:
Int16 occupies 2 bytes
Int16 belongs to the System.Int16 type
Int16 MIN value: -32768
Int16 MAX value: 32767
a = -32768, b = 32767
What is Int32 in C#?
Int32 is a structure in the .NET Framework that represents a 32-bit signed integer. It is part of the System namespace. The Int32 type provides methods and properties for working with 32-bit integers, and it is used interchangeably with int.
You must remember that int
is an alias of Int32, So, int and Int32 are the same data type.
- The Int32 data type is a 32-bit signed integer
- Int32 can store values ranging from -2,147,483,648 to 2,147,483,647
- The keyword ‘Int32’ is an alias for the
System.Int32
data type
Example of Int32 (or int) in C#
using System;
namespace ExampleInt32
{
class Program
{
static void Main(string[] args)
{
// Displaying information about Int32
Console.WriteLine("Int32 occupies {0} bytes", sizeof(Int32));
Console.WriteLine("Int32 belongs to the {0} type", typeof(Int32));
Console.WriteLine("Int32 MIN value: {0}", Int32.MinValue);
Console.WriteLine("Int32 MAX value: {0}", Int32.MaxValue);
Console.WriteLine();
// Int32 variables
Int32 a = -2147483648;
Int32 b = 2147483647;
Console.WriteLine($"a = {a}, b = {b}");
Console.ReadLine();
}
}
}
Output:
Int32 occupies 4 bytes
Int32 belongs to the System.Int32 type
Int32 MIN value: -2147483648
Int32 MAX value: 2147483647
a = -2147483648, b = 2147483647
What is Int64 in C#?
Int64
 is a structure in the .NET Framework that represents a 64-bit signed integer. It is also part of the System
 namespace. The Int64
 type can store much larger values than Int32
, ranging from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807.
- The Int64 data type is a 64-bit signed integer
- Int64 can store values ranging from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807
- The keyword ‘Int64’ is an alias for the
System.Int64
data type
Example of Int64 in C#
using System;
namespace ExampleInt64
{
class Program
{
static void Main(string[] args)
{
// Displaying information about Int64
Console.WriteLine("Int64 occupies {0} bytes", sizeof(Int64));
Console.WriteLine("Int64 belongs to the {0} type", typeof(Int64));
Console.WriteLine("Int64 MIN value: {0}", Int64.MinValue);
Console.WriteLine("Int64 MAX value: {0}", Int64.MaxValue);
Console.WriteLine();
// Int64 variables
Int64 a = -9223372036854775808;
Int64 b = 9223372036854775807;
Console.WriteLine($"a = {a}, b = {b}");
Console.ReadLine();
}
}
}
Output:
Int64 occupies 8 bytes
Int64 belongs to the System.Int64 type
Int64 MIN value: -9223372036854775808
Int64 MAX value: 9223372036854775807
a = -9223372036854775808, b = 9223372036854775807
int vs Int16 vs Int32 vs Int64 in C#
Data Type | Size | Minimum Value | Maximum Value |
---|---|---|---|
int | 32-bit | -2,147,483,648 | 2,147,483,647 |
int16 | 16-bit | -32,768 | 32,767 |
int32 | 32-bit | -2,147,483,648 | 2,147,483,647 |
int64 | 64-bit | -9,223,372,036,854,775,808 | 9,223,372,036,854,775,807 |
Properties and Methods of Int16, Int32, and Int64 in C#
Int16, Int32, and Int64 in C# are integral data types that are used to store numerical values. They are derived from the System.Int16
, System.Int32
, and System.Int64
classes respectively, and they inherit some common properties and methods from these classes.
Here are a few commonly used properties and methods of these data types:
Properties:
- MaxValue: This property returns the maximum value that can be stored in the respective data type.
- MinValue: This property returns the minimum value that can be stored in the respective data type.
Methods:
- Parse(string): This method converts a string representation of a number to its equivalent integral value.
- ToString(): This method converts and returns a string representation of the numerical value stored in the respective data type.
- TryParse(string, out TResult): This method attempts to convert a string representation of a number to its equivalent integral value and returns a Boolean indicating whether the conversion was successful or not.
It’s important to note that these properties and methods are common for all integral data types in C# and not just for Int16, Int32, and Int64.
Conclusion
- Knowing the differences between int, Int16, Int32, and Int64 in C# helps us in making informed decisions when choosing the right data type for a given situation
- Choosing a data type that is too large might lead to wasted memory while choosing a data type that is too small might result in overflow errors
- Always consider the range of values to be stored and choose the appropriate data type accordingly.
FAQs
Q: What is the difference between int, Int16, Int32, and Int64 in C#?
In short, Int16 can only store values up to 32,767, Int32 can store values up to 2,147,483,647 and Int64 can store values up to 9,223,372,036,854,775,807. Any values larger than these maximum values will not be able to be stored in their respective data types.
Q: What is the range of values that can be stored in ‘int’?
‘int’ is a 32-bit signed integer and can store values ranging from -2,147,483,648 to 2,147,483,647.
Q: What is the range of values that can be stored in Int16?
Int16 is a 16-bit signed integer and can store values ranging from -32,768 to 32,767.
Q: What is the range of values that can be stored in Int32?
Int32 is a 32-bit signed integer and can store values ranging from -2,147,483,648 to 2,147,483,647.
Q: What is the range of values that can be stored in Int64?
Int64 is a 64-bit signed integer and can store values ranging from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807.
Q: Is ‘int’ an alias for Int32?
Yes, ‘int’ is an alias for the System.Int32
data type.
Q: Can Int16 store negative values?
Yes, Int16 is a signed integer and can store both positive and negative values.
Q: Can Int64 store larger values than Int32?
Yes, Int64 is a 64-bit signed integer and can store larger values than Int32 which is a 32-bit signed integer.
Q: What is the difference between int and Int32?
int is simply an alias for Int32 in C#. They are functionally the same, and you can use either interchangeably. However, int is more commonly used for simplicity.
Q: When should I use Int16
instead of int
?
Use Int16
when you need to store small integer values within the range of -32,768 to 32,767 and want to save memory. This can be beneficial in performance-critical applications or when dealing with large arrays of small integers.
Q: Why would I use Int64
instead of int
?
Use Int64
when you need to store large integer values that exceed the range of int
(Int32
). This is useful in scenarios requiring high precision, such as certain financial calculations, large-scale data processing, or timestamp representations.
Q: Are there performance differences between int, Int32, and Int64?
In general, int and Int32 have the same performance since they are the same type. However, Int64 uses more memory (8 bytes) compared to int and Int32 (4 bytes), which can affect performance in scenarios where large arrays of integers are involved.
Q: What happens if I exceed the limits of int or Int32?
If you exceed the limits of int or Int32, you will encounter an OverflowException if you have checked for overflow using the checked keyword. If you do not check for overflow, the value will wrap around to the minimum value.
Q: Can I convert int to Int64?
Yes, you can convert int to Int64 implicitly without any special syntax, as Int64 can hold all values of int. However, converting from Int64 to int requires an explicit cast as it may lead to data loss if the Int64 value exceeds the range of int.
Articles you might also like:
- C# Abstract class Vs Interface
- C# Array Vs List
- Is vs As operator in C#
- Jump Statements in C# (Break, Continue, Goto, Return, and Throw)
- Singleton Design Pattern in C#
- Value Type and Reference Type in C#
- C# Stack Class With Push And Pop Examples
- C# Struct vs Class
- Difference between Boxing and Unboxing in C#
- C# dispose vs finalize
- ref and out keyword
- C# Tutorial
We would love to hear your thoughts on this post. Please leave a comment below and share it with others.
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025