C# TextWriter is a class that provides a way to write text or characters to a file, a stream, or a network socket.
The TextWriter class is part of the System.IO
namespace in C#. It is an abstract class that provides a way to write characters to a file or stream. It has several derived classes that provide different ways of writing text to a file, such as StreamWriter, StringWriter, and BinaryWriter.
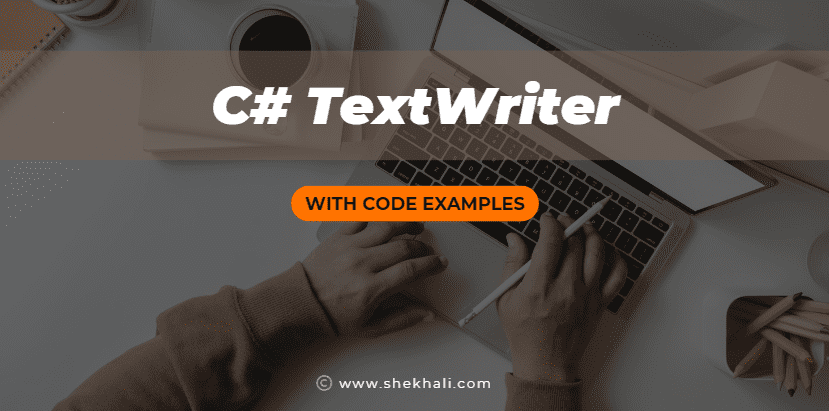
The TextWriter class provides several methods for writing text to a stream, such as Write and WriteLine. These methods are used to write strings or characters to a stream synchronously.
We can also use the async and await keywords to write text to a stream asynchronously using the WriteAsync and WriteLineAsync methods of the TextWriter class.
Table of Contents
- 1 C# TextWriter Syntax
- 2 How TextWriter works in C#?
- 3 C# TextWriter Example
- 4 C# TextWriter Methods
- 5 WriteLineAsync method of the TextWriter class
- 6 Points to Remember:
- 7 Summary
- 8 FAQs
- 8.1 Q: What is TextWriter in C#?
- 8.2 Q: What is the difference between Write and WriteLine methods in TextWriter class?
- 8.3 Q: How can the TextWriter class write text to a file asynchronously in C#?
- 8.4 Q: What are some of the derived classes of TextWriter in C#?
- 8.5 Q: Can TextWriter be used to write data to a database?
- 8.6 Q: Can TextWriter be used for writing binary data?
- 8.7 Related
C# TextWriter Syntax
The syntax for creating a TextWriter object is as follows:
TextWriter tw = new StreamWriter("filename.txt");
How TextWriter works in C#?
C# TextWriter class provides a simple and efficient way to write text to a file or stream. We can use the Write and WriteLine methods of the TextWriter class to write text to a file. The Write method writes a string or character to the file, while the WriteLine method writes a string or character and adds a new line to the file.
We can use the TextWriter class to write text to a file synchronously or asynchronously. The synchronous writing method blocks the program execution until the text is written to the file. On the other hand, the asynchronous writing method writes the text to the file without blocking the program execution.
C# TextWriter Example
Let’s look at an example of how to use the TextWriter class to write text to a file.
using System;
using System.IO;
class Program
{
static void Main()
{
// Create a new file to write to.
using (TextWriter tw = new StreamWriter("c://output.txt"))
{
// Write a string to the file.
tw.Write("Hello, World!");
// Write a string and a new line to the file.
tw.WriteLine("This is a test file.");
}
Console.WriteLine("Data written successfully to the file.");
}
}
Output:
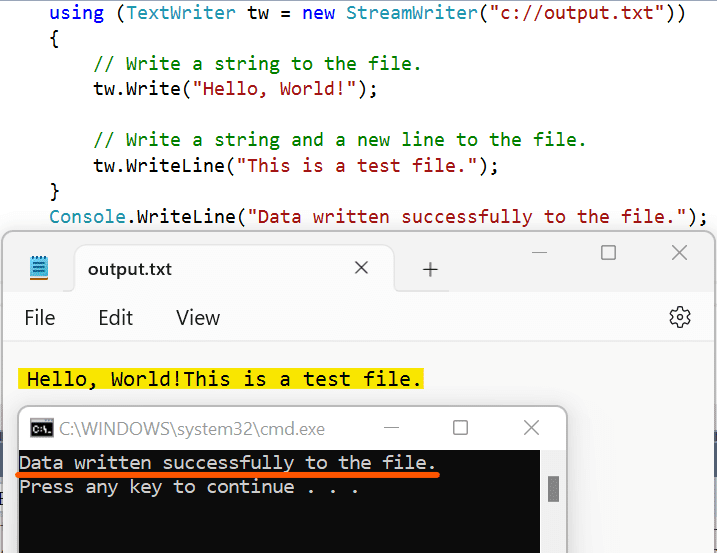
In this example, we create a new file named “output.txt” and use the StreamWriter
class to write text to it.
C# TextWriter Methods
The TextWriter class provides several methods to write text to a file. The most commonly used methods are the Write and WriteLine methods. The following table lists some of the methods provided by the TextWriter class, along with their descriptions:
The following are the list of C# TextWriter methods and their descriptions:
Method | Description |
---|---|
Synchronized(TextWriter) | It is use to Return a thread-safe wrapper for the TextWriter instance. |
Close() | This method is used to Close the TextWriter instance and the underlying stream. |
Dispose() | It releases all resources used by the TextWriter instance. |
Flush() | It is used to Flush the buffer of the TextWriter instance and writes any buffered data to the underlying stream. |
Write(Char) | It is used to write a single character to the TextWriter instance. |
Write(String) | It is used to write a string to the TextWriter instance. |
WriteAsync(Char) | It Asynchronously writes a single character to the TextWriter instance. |
WriteLine() | It is used to write a newline character to the TextWriter instance. |
WriteLineAsync(String) | It Asynchronously writes a string, followed by a newline character, to the TextWriter instance. |
C# TextWriter Explanation
- The Synchronized method returns a thread-safe wrapper for the TextWriter instance, which can be useful when multiple threads need to write to the same stream.
- The Close method closes the TextWriter instance and the underlying stream, flushing any buffered data to the stream before closing it.
- The Dispose method releases all resources the TextWriter instance uses, including any buffers or streams created.
- The Flush method flushes the buffer of the TextWriter instance and writes any buffered data to the underlying stream.
- The Write method writes a single character or a string to the TextWriter instance.
- The WriteAsync method asynchronously writes a single character to the TextWriter instance, which can be useful when the calling thread needs to continue executing while the write operation completes in the background.
- The WriteLine method writes a newline character to the TextWriter instance, which is equivalent to calling the Write method with the “\n” character.
- The WriteLineAsync method asynchronously writes a string, followed by a newline character, to the TextWriter instance. It can be useful when multiple threads must write to the same stream without blocking each other.
WriteLineAsync method of the TextWriter class
Here is an example of C# program that demonstrates the use of the WriteLineAsync
method of the TextWriter class:
using System;
using System.IO;
using System.Threading.Tasks;
class Program
{
static async Task Main()
{
using (var writer = new StreamWriter("output.txt"))
{
await writer.WriteLineAsync("This is the first line.");
await writer.WriteLineAsync("This is the second line.");
await writer.WriteLineAsync("This is the third line.");
}
Console.WriteLine("Done writing to the text file.");
}
}
The above program demonstrates how the WriteLineAsync method can be used to write text to a file asynchronously, which can be useful in scenarios where the main thread needs to continue executing while write operations complete in the background.
Points to Remember:
- TextWriter is a class in C# that provides a way to write text to a file or stream.
- TextWriter is an abstract class with several derived classes, such as StreamWriter, StringWriter, and BinaryWriter.
- We can use the Write and WriteLine methods of the TextWriter class to write text to a file synchronously.
- We can also use the async and await keywords to write text to a file asynchronously using the WriteAsync and WriteLineAsync methods of the TextWriter class.
Summary
In this article, we discussed the TextWriter class in C# and how to use it to write text to a file. We looked at examples of using the TextWriter class to write text to a file synchronously and asynchronously. We also discussed the different methods provided by the TextWriter class and their descriptions.
FAQs
Q: What is TextWriter in C#?
TextWriter is a class in the System.IO
namespace of C# that allows writing characters to a stream, such as a file or a network socket.
Q: What is the difference between Write and WriteLine methods in TextWriter class?
The Write method writes a string or character to the file, while the WriteLine method writes a string or character and adds a new line to the file.
Q: How can the TextWriter class write text to a file asynchronously in C#?
We can use the async and await keywords to write text to a file asynchronously using the WriteAsync
and WriteLineAsync
methods of the TextWriter
class.
Q: What are some of the derived classes of TextWriter in C#?
Some of the derived classes of TextWriter in C# are StreamWriter
, StringWriter
, and BinaryWriter
.
Q: Can TextWriter be used to write data to a database?
No, TextWriter is specifically designed for writing data to streams and is not suitable for writing data to a database. It would be best to use a database-specific API or ORM to write data to a database.
Q: Can TextWriter be used for writing binary data?
No, TextWriter is specifically designed for writing text data, and is not suitable for writing binary data. For writing binary data, you should use the BinaryWriter class instead.
References: MSDN-C# TextWriter
Recommended Articles:
- DateTime Format In C# (with examples)
- Interface in C# with examples
- IEnumerable Interface with code examples
- Array Vs List in C#
- Understanding IsNullOrEmpty and IsNullOrWhiteSpace
- C# Collection with code examples
- C# Dependency Injection with example
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- Difference between interface and abstract class In C#
- C++ vs C#: Difference Between C# and C++
- C# Regex: Introduction to Regular Expressions in C# with Examples
- C# String Interpolation – Syntax, Examples, and Performance
- C# Stopwatch Explained – How to Measure Code Execution Time?
- C# String Vs StringBuilder
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024