C# Stopwatch is a powerful class provided by the .NET Framework that we can use to measure the execution time of a specific code block.
It is commonly used in performance testing and benchmarking applications to measure the time a code snippet takes to execute.
The Stopwatch class is part of the System.Diagnostics
namespace and is available in all .NET programming languages, including C#.
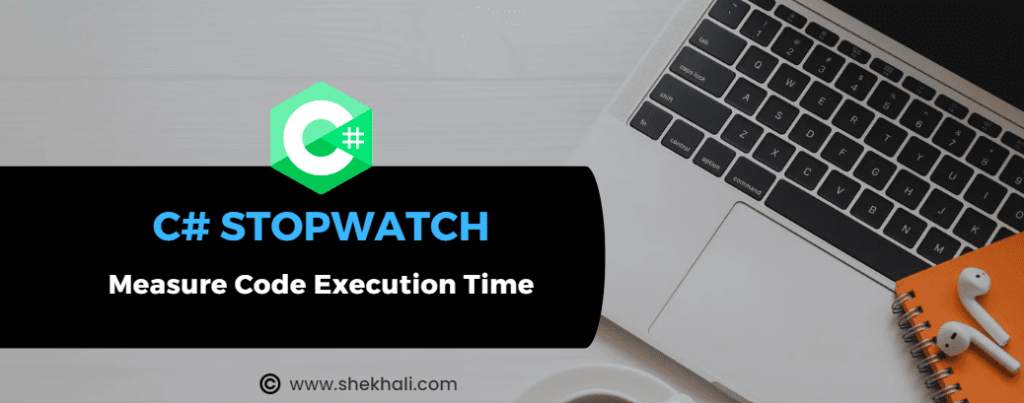
Table of Contents
- 1 Syntax:
- 2 Example:
- 3 Stopwatch Methods
- 4 Stopwatch Properties/Fields
- 5 Using the IsHighResolution Property of Stopwatch
- 6 Using the Restart() Method to Measure Multiple Operations
- 7 FAQs
- 7.1 Q: What is a Stopwatch in C#?
- 7.2 Q: How do I use the Stopwatch class in C#?
- 7.3 Q: What is the resolution of the Stopwatch class in C#?
- 7.4 Q: What is the difference between Elapsed and ElapsedMilliseconds properties in the Stopwatch class?
- 7.5 Q: Can you use the Stopwatch class to measure the performance of my code?
- 7.6 Related
Syntax:
The syntax for creating a Stopwatch object is as follows:
Stopwatch stopwatch = new Stopwatch();
Example:
Here is an example of how to use the Stopwatch class in C#:
using System;
using System.Diagnostics;
public class Program
{
public static void Main()
{
// Example usage of the Measure method
Console.WriteLine("Method 1");
Measure(() => Method1());
Console.WriteLine("Method 2");
Measure(() => Method2());
Console.ReadKey();
}
public static void Measure(Action action)
{
Stopwatch stopwatch = new Stopwatch();
stopwatch.Start();
action();
stopwatch.Stop();
Console.WriteLine($"Execution time: {stopwatch.ElapsedMilliseconds} ms");
}
public static void Method1()
{
// Code to be measured
for (int i = 0; i < 1000000; i++)
{
int x = i + i;
}
}
public static void Method2()
{
// Code to be measured
for (int i = 0; i < 10000000; i++)
{
int x = i * i;
}
}
}
Output:
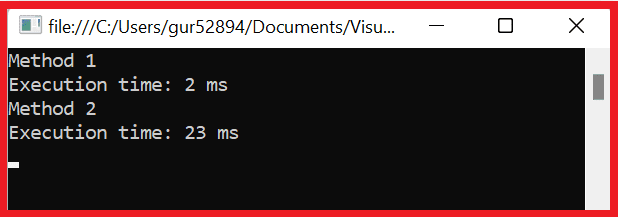
Stopwatch Methods
The Stopwatch class provides various methods to control the stopwatch and retrieve the elapsed time.
Here are some of the important methods:
Method | Description |
---|---|
Start(): | Starts the stopwatch. |
Stop(): | Stops the stopwatch. |
Reset(): | Resets the stopwatch to its initial state. |
Restart(): | Restart the stopwatch. |
StartNew(): | The StartNew() method initializes a fresh instance of the Stopwatch class, sets the elapsed time property to zero, and begins measuring the duration of elapsed time. |
GetTimestamp(): | The method GetTimestamp() retrieves the current tick count from the timer mechanism. |
Stopwatch Properties/Fields
In addition to the methods, the Stopwatch class provides properties that can retrieve information about the stopwatch. Here are some of the important properties:
Property | Description |
---|---|
Frequency: | Gets the frequency of the timer as the number of ticks per second. |
IsHighResolution: | Gets a value indicating whether the timer is based on a high-resolution performance counter. |
Elapsed: | Gets the total elapsed time measured by the current instance. |
ElapsedMilliseconds: | Gets the total elapsed time measured by the current instance in milliseconds. |
ElapsedTicks: | Gets the total elapsed time measured by the current instance in timer ticks. |
IsRunning: | The IsRunning property of the Stopwatch class indicates whether the timer is currently active or not. It returns a boolean value that is true if the timer is running and false if it has been stopped. |
Using the IsHighResolution Property of Stopwatch
IsHighResolution property of the Stopwatch class checks if high-resolution timing is supported on the system. We can measure the execution time of an operation with high accuracy using the ElapsedTicks property and the system’s performance counter frequency.
If high-resolution timing is not supported, the Stopwatch class returns to using the system’s standard timer, which has a lower resolution and may not provide accurate timing measurements.
Example:
using System;
using System.Diagnostics;
class Program
{
static void Main()
{
Stopwatch stopwatch = new Stopwatch();
if (Stopwatch.IsHighResolution)
{
stopwatch.Start();
// Perform operation
stopwatch.Stop();
// Get elapsed time in ticks
long elapsedTicks = stopwatch.ElapsedTicks;
double elapsedNanoseconds = (double)elapsedTicks / Stopwatch.Frequency * 1000000000;
Console.WriteLine($"Time taken: {elapsedNanoseconds} ns");
}
else
{
Console.WriteLine("High-resolution timing is not supported on this system.");
}
Console.ReadKey();
}
}
Output:
Time taken: 6000 ns
Please note that the output may vary when running the code on different systems or under different conditions.
Using the Restart() Method to Measure Multiple Operations
When measuring the execution time of multiple operations, you should restart the Stopwatch object instead of creating a new one for each operation. The Restart()
method resets the elapsed time and starts the Stopwatch again. Here is an example:
using System;
using System.Diagnostics;
public class Program
{
public static void Main()
{
Stopwatch stopwatch = new Stopwatch();
// First operation to measure
stopwatch.Start();
Method1();
stopwatch.Stop();
Console.WriteLine($"Execution time for Method1: {stopwatch.ElapsedMilliseconds} ms");
// Second operation to measure
stopwatch.Restart();
Method2();
stopwatch.Stop();
Console.WriteLine($"Execution time for Method2: {stopwatch.ElapsedMilliseconds} ms");
Console.ReadKey();
}
public static void Method1()
{
// Code for first operation
for (int i = 0; i < 1000000; i++)
{
int x = i + i;
}
}
public static void Method2()
{
// Code for second operation
for (int i = 0; i < 10000000; i++)
{
int x = i * i;
}
}
}
Output:
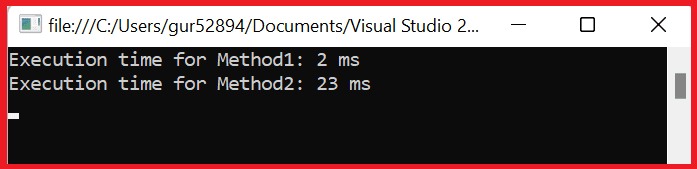
Refrences:Msdn – C# Stopwatch
FAQs
Q: What is a Stopwatch in C#?
A Stopwatch class in C# provides a high-resolution timer to measure elapsed time for code execution or other operations.
Q: How do I use the Stopwatch class in C#?
To use the Stopwatch class, you must create a new class instance. You can then call the Start method to start the timer and the Stop method to stop the timer and measure the elapsed time.
Q: What is the resolution of the Stopwatch class in C#?
The resolution of the Stopwatch class depends on the system hardware and operating system. It typically has a resolution of around 1 nanosecond.
Q: What is the difference between Elapsed and ElapsedMilliseconds
properties in the Stopwatch class?
The Elapsed property returns the elapsed time as a TimeSpan
object, which provides more precision than the ElapsedMilliseconds
property, which returns the elapsed time as a long integer in milliseconds.
Q: Can you use the Stopwatch class to measure the performance of my code?
Yes, the Stopwatch class is often used to measure code performance and identify areas that can be optimized for better performance.
You might also like:
- C# String Interpolation – Syntax, Examples, and Performance
- C# String Vs StringBuilder
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- 10 Difference between interface and abstract class In C#
- C# Queue with examples
- Web API vs web service: Top 10+ Differences between web API and web service in C#
- C# Polymorphism: Different types of polymorphism in C# with examples
- C# Stack
- Understanding the Difference between == and Equals() Method in C#
- Difference between SortedList and SortedDictionary in C#
- C# Hashtable vs Dictionary vs HashSet
- C# stack vs heap
- Format String in C#: A Comprehensive Guide to Understand String Formatting in C#
- Fibonacci sequence: Fibonacci series in C# (with examples)
- Different Ways to Calculate Factorial in C# (with Full Code Examples)
- Advantages and Disadvantages of Non-Generic Collection in C#
- Difference between readonly and const keywords in C#
Let others know about this post by sharing it and leaving your thoughts in the comments section.
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025