Here we will learn how to write a program to copy all elements of an array into another array using different programming languages like C
, C#
, Python
, Java
, and PHP
.
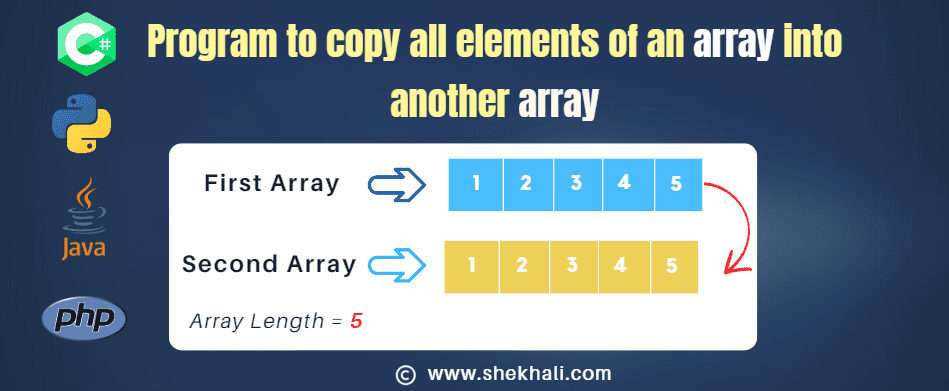
Copying all elements from one array into another is a common programming task.
Let’s take a look at some examples in different programming languages to understand how to copy all elements from one array to another.
C# Example:
using System;
class Program
{
static void Main()
{
// Declare and initialize the first array
int[] arr1 = {1, 2, 3, 4, 5};
// Declare and initialize the second array with the same length as the first array
int[] arr2 = new int[arr1.Length];
// Copy all elements of arr1 to arr2 using the Array.Copy() method
Array.Copy(arr1, arr2, arr1.Length);
// Print the elements of the first array
Console.WriteLine("Elements of first array:");
foreach (int i in arr1)
{
Console.Write(i + " ");
}
// Print the elements of the second array
Console.WriteLine("\nElements of second array:");
foreach (int i in arr2)
{
Console.Write(i + " ");
}
}
}
Output:
Elements of first array:
1 2 3 4 5
Elements of second array:
1 2 3 4 5
Explanation:
In this example, we create two integer arrays – arr1 and arr2. We use the Array.Copy()
method to copy all elements from arr1 to arr2. Finally, we print the elements of both arrays using a loop.
Python Example:
Here is an example of how to copy all elements of one Array into another array using Python.
# create a list named arr1 with some elements
arr1 = [1, 2, 3, 4, 5]
# use the copy() method to copy all elements from arr1 to arr2
arr2 = arr1.copy()
# print the elements of arr1 using a for loop
print("Elements of first array:")
for i in arr1:
print(i, end=' ')
# print the elements of arr2 using a for loop
print("\nElements of second array:")
for i in arr2:
print(i, end=' ')
Output:
Elements of first array:
1 2 3 4 5
Elements of second array:
1 2 3 4 5
In the above example, we create a list named arr1
with five elements. We use the copy()
method to create a new list named arr2
and copy all elements from arr1 to arr2.
Then, we print the elements of both lists using for
loops. The end parameter of the print()
function is set to a space, so the elements are printed in a single line separated by spaces.
C Example:
Here is an example of how to copy all elements of an array into another array using C programming.
#include <stdio.h>
void main()
{
int arr1[] = {1, 2, 3, 4, 5};
int arr2[5];
for(int i=0; i<5; i++)
{
arr2[i] = arr1[i];
}
printf("Elements of first array:\n");
for(int i=0; i<5; i++)
{
printf("%d ", arr1[i]);
}
printf("\nElements of second array:\n");
for(int i=0; i<5; i++)
{
printf("%d ", arr2[i]);
}
}
Java Example:
Here is an example of how to copy all elements of one array into another array using Java.
public class Main
{
public static void main(String[] args)
{
// Create an integer array called "arr1" with initial values
int[] arr1 = {1, 2, 3, 4, 5};
// Create a new integer array called "arr2" with the same length as "arr1"
int[] arr2 = new int[arr1.length];
// Copy all the elements from "arr1" to "arr2"
System.arraycopy(arr1, 0, arr2, 0, arr1.length);
// Print the elements of "arr1"
System.out.println("Elements of first array:");
for(int i=0; i<arr1.length; i++)
{
System.out.print(arr1[i] + " ");
}
// Print the elements of "arr2"
System.out.println("\nElements of second array:");
for(int i=0; i<arr2.length; i++)
{
System.out.print(arr2[i] + " ");
}
}
}
Output:
Elements of first array:
1 2 3 4 5
Elements of second array:
1 2 3 4 5
In this code, we create two integer arrays – arr1 and arr2. We use the System.arraycopy()
method to copy all elements from arr1 to arr2. Finally, we print the elements of both arrays using a for
loop.
PHP Example:
Here is an example of how to copy all elements of one array into another array using PHP.
<?php
// Initialize the first array with some elements
$arr1 = array(1, 2, 3, 4, 5);
// Initialize an empty second array
$arr2 = array();
// Loop through each element of the first array
foreach($arr1 as $value)
{
// Add the current element to the second array using the array_push() function
array_push($arr2, $value);
}
// Print the elements of the first array
echo "Elements of first array:\n";
foreach($arr1 as $value)
{
echo $value . " ";
}
// Print the elements of the second array
echo "\nElements of second array:\n";
foreach($arr2 as $value)
{
echo $value . " ";
}
?>
Output:
Elements of first array:
1 2 3 4 5
Elements of second array:
1 2 3 4 5
Recommended Articles:
- Program to copy all elements of an array into another array
- C# Program to Convert Decimal to Binary with Examples
- C# Program to Convert Binary to Decimal with Examples
- C# Program to Convert Hexadecimal to Decimal
- How to remove duplicate characters from a String in C#
- C# program to count the occurrences of each character in a String
- C# Program to Print Multiplication Table of a Given Number
- Program to print prime numbers from 1 to N
- C# Program to Check if a Given Number is Even or Odd
- Program to copy all elements of an array into another array
- Different Ways to Calculate Factorial in C# (with Full Code Examples)
- C# Programs asked in Interviews
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024
- How to Reverse an Array in C# with Examples - February 12, 2024