The If-Else and Switch statements allow you to make decisions based on the outcome of an expression. If-else operates through linear search whereas the Switch statement uses binary search. “If-else” and “switch” are conditional statements, but they work in different ways.
In this article, we will understand the key differences between If-Else and Switch statements in C#.
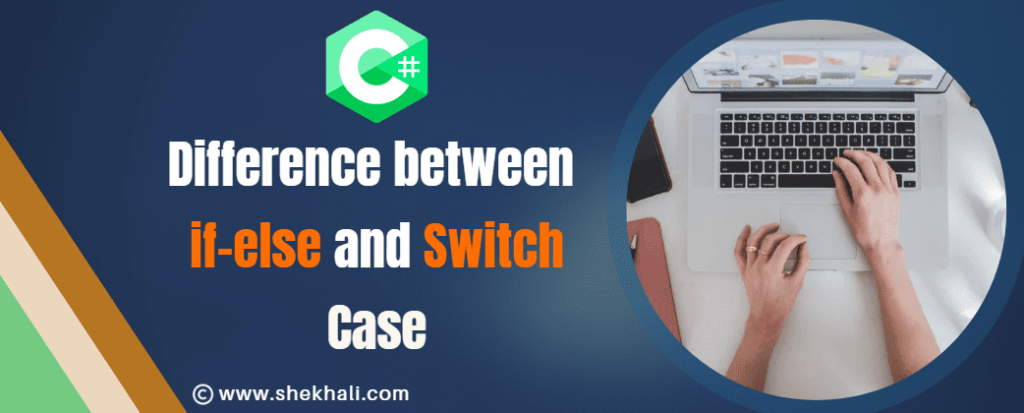
Table of Contents
What is an If-Else statement?
The C# if-else statement checks a Boolean expression and executes code depending on whether the expression is true or false. If the expression returns as true, the code within the if block will be executed. However, if the expression is false, the code within the else block will be executed.
Syntax:
if(condition)
{
//code executes if condition is true
}
else
{
//code executes if condition is false
}
The basic structure of an If-Else statement is that if the condition is true, the code inside the if statement will be executed and If the condition is false, then the code inside the else block will be executed.
Example:
The following code demonstrates how to use an If-Else statement to determine whether a number is even or odd:
using System;
class Program
{
static void Main(string[] args)
{
int num = 6;
if (num % 2 == 0)
{
Console.WriteLine("The number is even.");
}
else
{
Console.WriteLine("The number is odd.");
}
}
}
// Output: The number is even.
Diagram: if-else statement
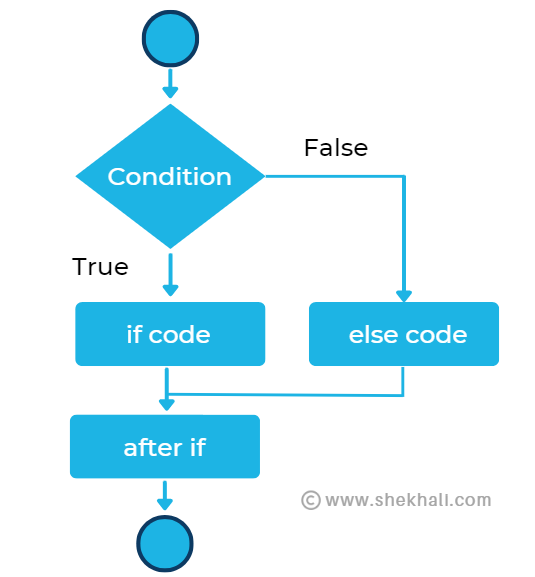
What is a Switch Case Statement?
The Switch statement is another control flow structure used to make decisions based on the value of an expression. Unlike the If-Else statement, the Switch statement allows you to evaluate an expression and execute one of many blocks of code based on the result.
Syntax:
switch(expression)
{
case x:
// code block
break;
case y:
// code block
break;
case z:
// code block
break;
default:
// code block
break;
}
Example:
The following code example will demonstrate how to use a Switch statement to determine the name of a day of the week based on a number:
int day = 3;
switch (day)
{
case 1:
Console.WriteLine("Monday");
break;
case 2:
Console.WriteLine("Tuesday");
break;
case 3:
Console.WriteLine("Wednesday");
break;
// Add additional cases as needed
default:
Console.WriteLine("Invalid day.");
break;
}
Flowchart: C# Switch Statement
The following is the flowchart of the Switch statement in C#.
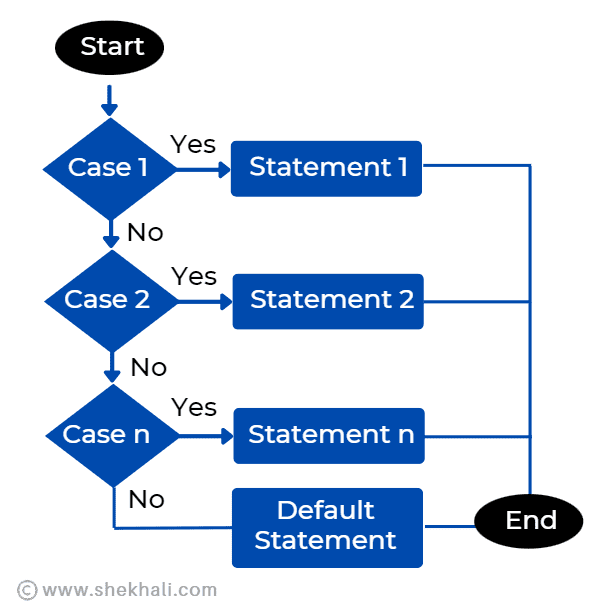
Difference between If-Else and Switch statements in C#
Feature | If-Else Statement | Switch Statement |
---|---|---|
Syntax: | It is complex and requires multiple lines of code to define each branch of the decision. | It is simple and allows you to define each branch of the decision using a single line of code. |
Definition: | The execution of the if and else blocks is based on the condition specified in the if statement. | Meanwhile, the switch statement has several cases, and the code block associated with the selected case will be executed. |
Evaluation: | If-else basically evaluates an expression and executes one of two blocks of code based on the result. | On the other hand, the Switch statement evaluates an expression and executes one of many blocks of code based on the result. |
Performance: | It can be slower than the Switch statement in certain scenarios, as it requires multiple comparisons to determine which branch of the decision to execute. | It is faster than the If-Else statement, as it only requires a single comparison to determine which branch of the decision to execute. |
Search: | The If statement uses Linear search. | The switch statement uses Binary search. |
Suitability: | if-else statement is best suited for simple decisions with two branches. | whereas, Case statement is best suited for complex decisions with multiple branches. |
Expression: | support multiple statements for multiple decisions. | In Switch case, we can have single statements for multiple decisions. |
Data Types: | The if condition can be used with data types such as float, double, char, int, and so on, | whereas only int and char, long, or string data types can be utilized in the switch block. |
If-Else vs Switch statements in C#?
The key differences between If-Else and Switch statements in C# are:
- The syntax of the
If-Else
statement is more complex than theSwitch
statement, as it requires multiple lines of code to define each branch of the decision. - When the condition in an if statement is false, the statements in the corresponding else block is executed. In a switch statement, if the condition is false, the default statements will be executed.
- For multiple statements, you can use multiple if statements. In contrast, for multiple statements in a Switch, you only have a single expression.
- The If-Else statement evaluates an expression and executes one of two blocks of code based on the outcome or result. on the other hand, the Switch statement evaluates an expression and executes one of many blocks of code based on the result.
- The values of an if-else statement are determined by constraints, while the values of a switch case are based on the user’s preferences.
- The If-Else statement can be slower than the Switch statement in certain scenarios, as it requires multiple comparisons to determine which branch of the decision to execute. on the other hand, the Switch statement is generally faster than the If-Else statement, as it only requires a single comparison to determine which branch of the decision to execute.
Rules for switch statement:
- The
switch
statement must have an expression, which is usually variable, that is used to determine which case is executed. - The expression must be of an integral type (such as int, char, or long) or a string type.
- The switch statement consists of multiple cases which are marked by the case keyword.
- The case statement must include a value that matches the expression in the switch statement.
- The switch statement includes a default case that is executed if no other cases match the expression.
- The case statements are followed by a colon and then a block of code to be executed if the case is selected.
- The break statement is used at the end of each case to exit the switch statement and avoid fall-through.
- If the break statement is not used, the flow of control falls through to the next case statement, executing all the code in that case and subsequent cases.
Summary:
In summary, The If-Else statements are best suited for simple decisions with two branches, on the other hand, the Switch statements are best suited for complex decisions with multiple branches.
FAQs
Q: What is an If-Else statement in C#?
The If-Else
statement is a basic control flow structure in C# that allows you to make decisions based on the outcome of an expression. It evaluates the expression and executes one of two blocks of code based on the result.
Q: What is a Switch statement?
The Switch statement is another control flow structure in C# that allows you to make decisions based on the value of an expression. Unlike the If-Else statement, the Switch statement allows you to evaluate an expression and execute one of many blocks of code based on the result.
Q: When should you use an If-Else statement in C#?
The If-Else statement is best suited for simple decisions with two branches. For example, determining whether a number is even or odd.
Q: When should you use a Switch statement in C#?
The Switch statement is best suited for complex decisions with multiple branches. For example, determining the name of a day of the week based on a number.
References: MSDN-if, else and switch statements
You might also like:
- C# switch Statement (With Examples)
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- C# Struct vs Class |Top 15+ Differences Between C# Struct and Class
- 10 Difference between interface and abstract class In C#
- Local vs Global Variables
- C# stack vs heap
- Readonly vs const in C#
- Fields vs Properties In C#: Understanding the difference between Field and Property in C#
Let others know about this post by sharing it and leaving your thoughts in the comments section.
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024
- How to Reverse an Array in C# with Examples - February 12, 2024