A Hashtable, Dictionary, and HashSet are all data structures that store and retrieve data based on keys. However, there are some important differences between them which we learn in this post.
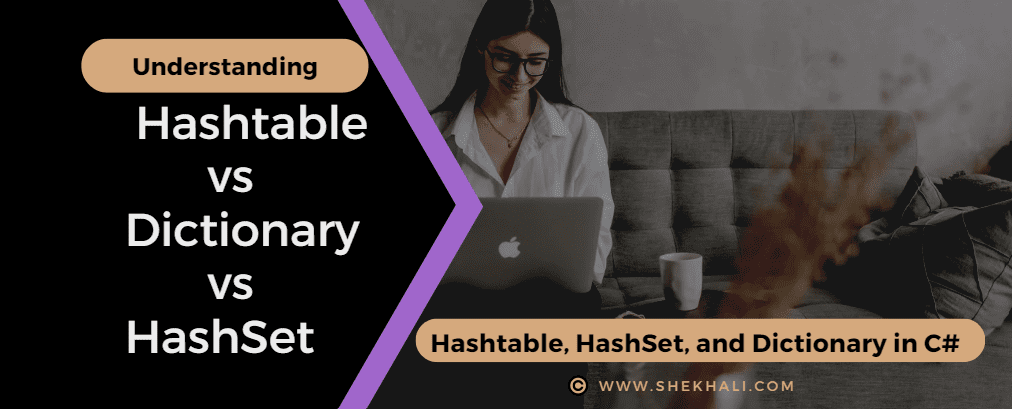
A Hashtable, Dictionary, and HashSet are all data structures that store and retrieve data based on keys. However, there are some important differences between them which we learn in this post.
C# Events: The Events enable a class or object to notify other classes or objects when some interesting thing happens to the object. A class that sends or raises the event is called the publisher, and the classes that receive or handle the event are referred to as subscribers.
There can be multiple subscribers to a single event. Typically, when an action takes place, a publisher raises an event. The subscribers who want to be notified when an action takes place should register or subscribe to handle specific events.
The following diagram shows the event in C#.
Comments are used in a program to make a section of code easier to understand. They’re used to make the code more readable and provide a formal description of it and how it works.
The compiler ignores comments, so you may put them wherever in a program and not worry about them breaking it.
In this post, we’ll look at a simple but difficult interview question in C#: Call By Value vs Call By Reference.
This is a question that both fresher and experienced developers are asked in interviews. It’s one of the most frequently asked questions by technical interviewers.
In C#, the key difference between abstraction and encapsulation is that encapsulation wraps data and methods into a single unit called class, whereas abstraction hides implementation details and just shows users the functionality.
A collection in C# is a class that represents a group of objects. Collections can be used to perform various operations on objects, such as storing, updating, deleting, retrieving, searching, and sorting.
Collections are defined in the System.Collections
namespace. They are a predefined set of classes for storing multiple items in a single unit.
WCF (Windows Communication Foundation) is a framework for building service-oriented applications. It allows communication between applications on different devices, across computers and networks. WCF is part of the .NET framework, and it provides a runtime environment for applications that use distributed communication.
Web API is a framework for building HTTP services. It is a part of the ASP.NET platform and it allows you to create RESTful (representational state transfer) services, which can be consumed by a wide range of clients including web browsers and mobile devices.
This article will provide a practical comparison of WCF and Web API to help you decide which is the better option for your needs. We will discuss the differences between these two frameworks in detail.
Difference Between Struct and Class in C#:
One key difference between structs and classes is that Structs are value types, whereas classes are reference types.
In the case of structs, they are copied by value, meaning that a complete replica of the data is created when passed or assigned. On the other hand, classes are copied by reference, meaning that only a reference to the original data is passed around rather than a full duplication.
One of the fundamental features of C# is the use of access modifiers. These modifiers control the accessibility of classes, methods, and variables in your code.
In this article, we will take a comprehensive look at C# access modifiers, exploring their various types, their effects on code, and best practices for using them.
A Dictionary in C# is a collection that stores a set of keys and values, similar to a Hashtable. Dictionaries are useful for storing data that needs to be quickly retrieved using a key.
Dictionary<TKey, TValue>
is a generic collection that stores key/value pairs data organized by the unique key.System.Collections.Generic
namespace. It is a dynamic collection that can be resized on-the-fly, allowing you to add or remove items as needed. It means that you don’t have to pre-define the size of the dictionary, and it can grow or shrink depending on the data being stored.An exception is defined as an event that occurs during the program execution that is unexpected by the program code. Exception handling is important because it handles an unwanted exception so that your program code does not crash and remains meaningful to the user.
In this article, we will discuss the exception types and exception handling in C# using try, catch, and finally blocks.
An enum, or enumeration, is a value type in C# that consists of a set of named constants called the members of the enum. Enums are useful when you have a fixed set of values that a variable can take on, such as the days of the week or a set of predefined options.
Enums are value types, so they are stored in the stack rather than the heap. This means that they are faster to access than reference types, but they also take up more memory.
In this post, we will learn the proper usage of an enum in c# with multiple examples.
The lock
keyword in C# is used to place around a critical section of code, where we want to allow only one thread to access the resource at a time. Any other thread cannot access the lock and it waits for the lock to be released.
In this post series, we will go through the understanding of the lock
keyword, monitor
, mutex
, and semaphore
available in C#.
All of these classes (lock, monitor, mutex, and semaphore) provide a synchronization mechanism to protect the shared code or resources in a multithreaded application.
In C#, multithreading is the ability of a central processing unit (CPU), or a single-core or multi-core processor, to execute multiple threads concurrently. This allows the program to perform multiple tasks simultaneously, making the program more efficient and responsive.
Reflection in C# is the process of retrieving metadata of types, modules, assemblies, and more during runtime. With reflection, you can dynamically create an instance of a type, associate a type with an existing object, obtain the type of an existing object, and call its methods, fields, and properties.
In this article, we will explore the concept of C# Reflection, its hierarchy, when to use it, and some examples of its implementation.
A Private Constructor is an instance constructor used to prevent creating an instance of a class if it has no instance fields or methods. It is used in classes that contain only static members.
In this article, we will explore the concept of a private constructor, its uses, and how it can be implemented in C# programming.
Last updated on March 13th, 2023 at 07:28 pm A constructor in C# is a special method used to initialize …
In C#, the keywords static
, const
, readonly
, and static readonly
are frequently used, but they can be confusing. Today, we will discuss these keywords and use some examples to better understand them.