In C#, the var keyword is used to declare a variable whose type is implicitly determined by the compiler at compile-time. On the other hand, the dynamic keyword allows for variables whose type can change at runtime. It gives us the flexibility to work with different types of values dynamically.
So, var is for automatically determining the type at compile-time, while dynamic is for having variables that can change their type at runtime.
In this article, we will explore the differences between var and dynamic keywords in C# with multiple code examples.
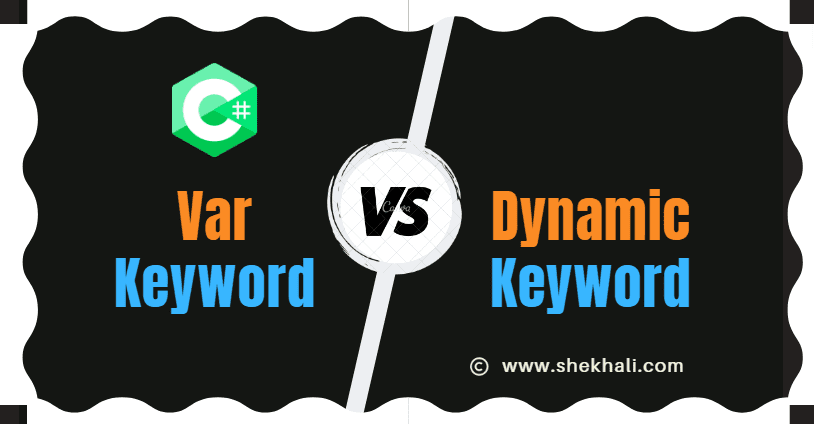
Table of Contents
- 1 What is the var keyword?
- 2 Feature of var keyword in C#:
- 3 What is the dynamic keyword?
- 4 Feature of Dynamic keyword in C#:
- 5 Example to understand Var and Dynamic Keywords in C#
- 6 Var VS Dynamic in C#
- 7 Which is better var or dynamic in C#?
- 8 Dynamic variable with different types of values
- 9 FAQs
- 9.1 Q: Can var be used with method return types?
- 9.2 Q: What is the difference between var and dynamic in C#?
- 9.3 Q: When should I use var and dynamic?
- 9.4 Q: Is it advisable to use the dynamic type in C#?
- 9.5 Q: Can I assign any value to a variable declared with the dynamic keyword?
- 9.6 Q: Does the var keyword make my code dynamically typed?Â
- 10 Conclusion: Var and Dynamic Keywords in C#
What is the var keyword?
The var keyword was introduced in C# 3.0, which allows developers to declare implicitly typed local variables without explicitly specifying their types.
The compiler determines the type of var variable based on the assigned value during compile time.
Here is an example to illustrate the usage of the var variable in C#:
using System;
class program
{
public static void Main()
{
var name = "Roman";
var age = 30;
Console.WriteLine("Name: " + name.GetType());
Console.WriteLine("Age: " + age.GetType());
}
}
Output:
Name: System.String
Age: System.Int32
In the above example, we have declared two variables name
and age
using the var
keyword. The type of name
is inferred as string
, and the type of age
is inferred as int
.
Using the keyword “var” saves you from repeating long and complicated type names. Instead, you can focus on the values you are assigning.
Var variable support IntelliSense feature
Visual Studio shows IntelliSense in the given graphic for var variables because the compiler can determine the type of the assigned variable during compile time.
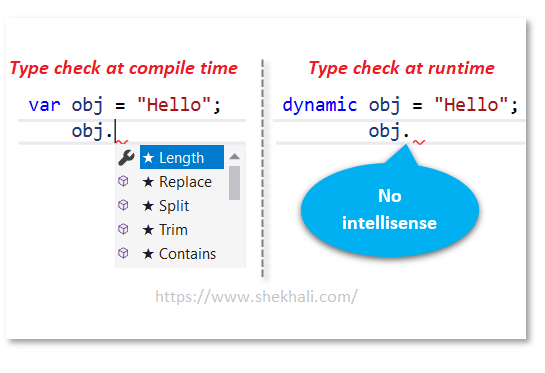
Feature of var keyword in C#:
- Version: Var keyword was introduced in C# 3.0.
- Type: Var is resolved at compile-time.
- Type Alteration: Once the var variable is assigned, the type of the value cannot be changed. Type consistency is enforced.
- Scope: Var variable is limited to local variables within methods. It cannot be used for properties or return values from methods.
- Type checking: Infers type from the assigned value.
- Error: Compile-time error for incompatible types.
- Performance: Efficient due to compile-time type checking.
- Initialization: Throws an error if a variable is not initialized at declaration.
- IntelliSense Support: Var supports IntelliSense in Visual Studio.
Refer to this link to learn more about var keyword in C#
What is the dynamic keyword?
The dynamic keyword is used to declare variables with a dynamic type. When you declare a variable using the dynamic keyword, the type of the variable is determined at runtime. Unlike other variables, dynamic variables allows to hold values of different types at runtime.
The type checking for dynamic variables occurs at runtime rather than during compile time.
It means that the compiler doesn’t perform static type checking on dynamic variables. It provides more flexibility in programming but can also lead to runtime errors if not used carefully.
Let’s explore an example to understand it better.
using System;
class program
{
public static void Main()
{
dynamic name = "John";
dynamic age = 30;
Console.WriteLine("Name: " + name.GetType());
Console.WriteLine("Age: " + age.GetType());
// Dynamic type can be change at runtime
dynamic item = "Hello";
Console.WriteLine("item type: " + item.GetType());
// Changing type
item = 100.5;
Console.WriteLine("item type: " + item.GetType());
}
}
Output:
Name: System.String
Age: System.Int32
item type: System.String
item type: System.Double
Dynamic is beneficial when dealing with scenarios where the type is unknown or might change dynamically, such as interacting with dynamic languages or working with external APIs.
Feature of Dynamic keyword in C#:
- Version: Introduced in C# 4.0.
- Type: Dynamic variable resolved at runtime.
- Type Alteration: Dynamic variable allows changing the type of the assigned value after the initial assignment.
- Scope: Dynamic variable allows creating properties and returning values from functions.
- Type checking: Dynamic variable does not perform static type checking.
- Error: Runtime error for incompatible operations.
- Performance: Potential runtime overhead due to dynamic type checking.
- Initialization: No error if the dynamic variable is not initialized at the declaration time.
- IntelliSense Support: Visual Studio does not support IntelliSense for dynamic variables.
Refer to this link to learn more about Dynamic keyword in C#
Example to understand Var and Dynamic Keywords in C#
To further illustrate the differences between var and dynamic, let’s consider an example where we have a list of integers and calculate their sum using both keywords:
var numbers = new List<int> { 1, 2, 3, 4, 5 };
var sum = 0;
foreach (var number in numbers)
{
sum += number;
}
Console.WriteLine("Sum using var: " + sum);
dynamic numbersDynamic = new List<int> { 1, 2, 3, 4, 5 };
dynamic sumDynamic = 0;
foreach (dynamic number in numbersDynamic)
{
sumDynamic += number;
}
Console.WriteLine("Sum using dynamic: " + sumDynamic);
Output:
Sum using var: 15
Sum using dynamic: 15
Var VS Dynamic in C#
To summarize the differences between var and dynamic, let’s compare them side by side.
Parameters | Var | Dynamic |
---|---|---|
Version: | var in C# language was introduced in C# 3.0 | On the other hand, the dynamic keyword was introduced in C# 4.0 |
Type: | Var type is resolved at compile-time | Dynamic type is resolved at runtime |
Type Alteration: | Once a value is assigned to a variable using var, the type of that value cannot be changed. For example, if a var variable is assigned with an integer value, it can’t be changed to a string. | On the other hand, when using dynamic, the type of the assigned value can be changed after the initial assignment. |
Scope: | The var keyword is limited to being used as a local variable within a method and cannot be utilized for properties or as return values from methods. | Conversely, dynamic variables offer the flexibility to create properties and return values from functions. |
Usage: | Infers type from assigned value | Does not perform static type checking |
Error: | Compile-time error for incompatible types | Runtime error for incompatible operations |
Performance: | Var is efficient due to compile-time type checking | Potential runtime overhead due to dynamic type checking |
Initialization: | Var throws an error if a variable is not initialized at the time of declaration. | There will be no error if the dynamic variable is not initialized. |
IntelliSense Support: | In Visual Studio, var supports IntelliSense. | Visual Studio does not support IntelliSense for Dynamic variables. |
Exception: | Variables declared with the var keyword in C# will throw an exception if they are not initialized at the time of declaration. | On the other hand, variables declared with the dynamic keyword do not throw any exception if they are not initialized at the time of their declaration. |
Which is better var or dynamic in C#?
Dynamic variables can be used to create properties and return values from a method. On the other hand, var variables are limited to being used as local variables within a method. They cannot be used for properties or return values similarly.
Here is an example:
using System;
public class Person
{
dynamic name;
dynamic age;
public dynamic Name
{
get { return name; }
set { name = value; }
}
public dynamic Age
{
get { return age; }
set { age = value; }
}
public dynamic GetDetails()
{
return $"Name: {Name}, Age: {Age}";
}
}
public class Program
{
public static void Main()
{
Person person = new Person();
person.Name = "Shekh Ali";
person.Age = 25;
Console.WriteLine(person.GetDetails());
}
}
Output:
Name: Shekh Ali, Age: 25
Dynamic variable with different types of values
Here is an example code snippet in C# where a dynamic variable is assigned with different types of values:
using System;
public class Program
{
public static void Main()
{
dynamic myVariable;
// Assigning an integer value
myVariable = 10;
Console.WriteLine("Value: " + myVariable + ", Type: " + myVariable.GetType());
// Assigning a string value
myVariable = "Hello, World!";
Console.WriteLine("Value: " + myVariable + ", Type: " + myVariable.GetType());
// Assigning a boolean value
myVariable = true;
Console.WriteLine("Value: " + myVariable + ", Type: " + myVariable.GetType());
}
}
Output:
Value: 10, Type: System.Int32
Value: Hello, World!, Type: System.String
Value: True, Type: System.Boolean
FAQs
Here are some frequently asked questions related to var and dynamic keywords in C#:
Q: Can var be used with method return types?
Yes, var
can be used to declare variables with return types of methods. The compiler infers the type based on the method’s return value.
Q: What is the difference between var and dynamic in C#?
Variables declared using the var keyword in C# are statically typed, meaning their types are determined by the compiler at compile time. On the other hand, variables declared using the dynamic keyword are dynamically typed, and their types are determined by the compiler at runtime.
In summary, var is statically typed and resolved at compile-time, while dynamic is dynamically typed and resolved at runtime.
Q: When should I use var and dynamic?
Use var when the type can be easily inferred from the assigned value, enhancing code readability. Use dynamic when dealing with scenarios that require runtime flexibility, such as interacting with dynamic languages or COM APIs.
Q: Is it advisable to use the dynamic type in C#?
The dynamic type can be beneficial in certain scenarios where you need flexibility and late binding, such as when interacting with dynamic languages, COM objects, or when the type is not known until runtime. It allows for dynamic dispatch and provides a way to handle dynamic scenarios that would be challenging with static typing.
However, it’s important to consider the potential downsides. The dynamic type bypasses static type checking, which can increase the risk of runtime errors and make code harder to debug and maintain. It also incurs a performance cost due to runtime type checking.
Q: Can I assign any value to a variable declared with the dynamic keyword?
Yes, you can assign values of different types to a variable declared with the dynamic keyword. It allows storing values of various types and performs type checking at runtime.
Q: Does the var keyword make my code dynamically typed?Â
No, the var keyword does not make your code dynamically typed. It still enforces static typing, where the variable’s type is determined at compile-time and cannot be changed afterward.
Conclusion: Var and Dynamic Keywords in C#
In conclusion, the var and dynamic keywords in C# offer different approaches to typing variables.
The var keyword allows for implicit typing, where the compiler determines the type based on the assigned value during compilation. It simplifies code, reduces redundancy, and enhances readability, especially in cases where the type is evident from the initializer expression.
On the other hand, the dynamic keyword provides dynamic typing, enabling variables to hold values of different types and performing type checking at runtime. It offers flexibility when dealing with dynamic languages, interacting with APIs returning dynamic objects, or handling scenarios where the type is unknown or may change dynamically.
Recommended Articles:
- Array Vs List in C#
- Var keyword in C# with examples
- Dynamic keyword in C# with examples
- Interface in C# with examples
- IEnumerable Interface with code examples
- C# Collection with code examples
- DateTime Format In C# (with examples)
- C# Dependency Injection with example
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- SOLID Design Principles in C#: A Complete Example
- Difference between interface and abstract class In C#
- C++ vs C#: Difference Between C# and C++
- C# Regex: Introduction to Regular Expressions in C# with Examples
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024
- How to Reverse an Array in C# with Examples - February 12, 2024