As a programming language, C# has gone through several iterations, and with each new version, we see new features and enhancements that make programming with it even better.
C# 10, the latest language version, is no exception.
In this article, we will explore the new features of C# 10 and provide examples of how to use them in your code.
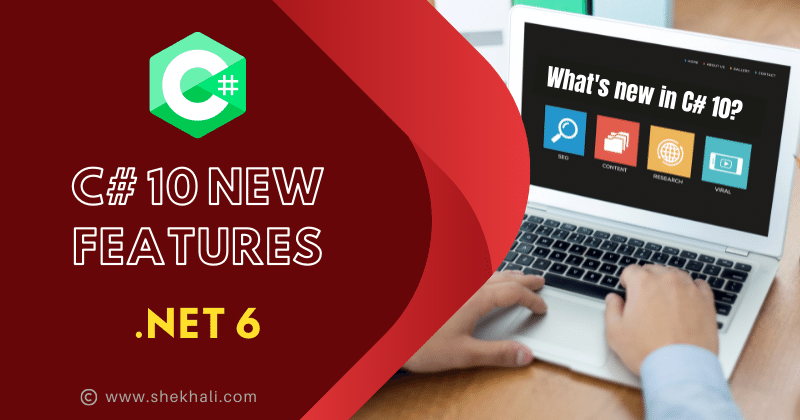