C# Field Vs Property: The main difference between a Field and a Property in C# is that a field is simply a variable of any type declared within a class or struct, whereas a property is a class member that offers a convenient way to access, modify, or calculate the value of a private field.
Fields are standard class variables, and properties are an abstraction for accessing their values.
In this tutorial, you will learn about the difference between field and property in C# and how to use them effectively.
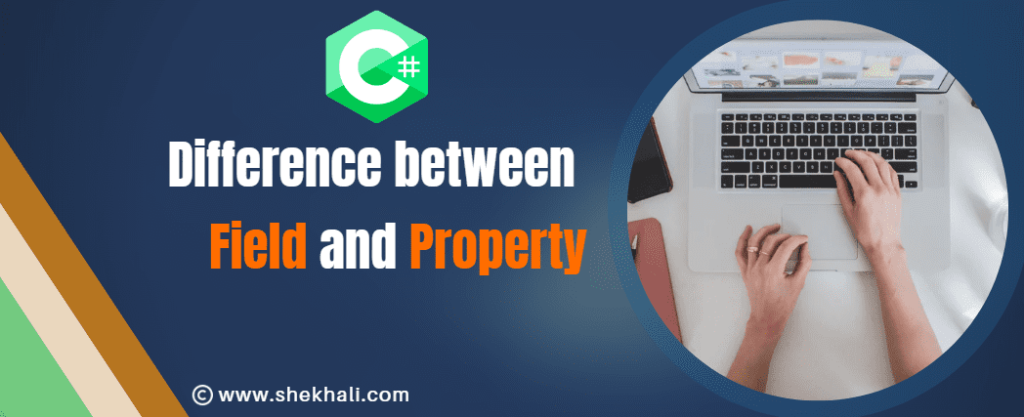