Calculating factorial is a common task in programming. In C#, a factorial can be calculated in several ways, including loops and recursion. In this article, we will explore different ways to calculate factorial in C# and provide full code examples with explanations.
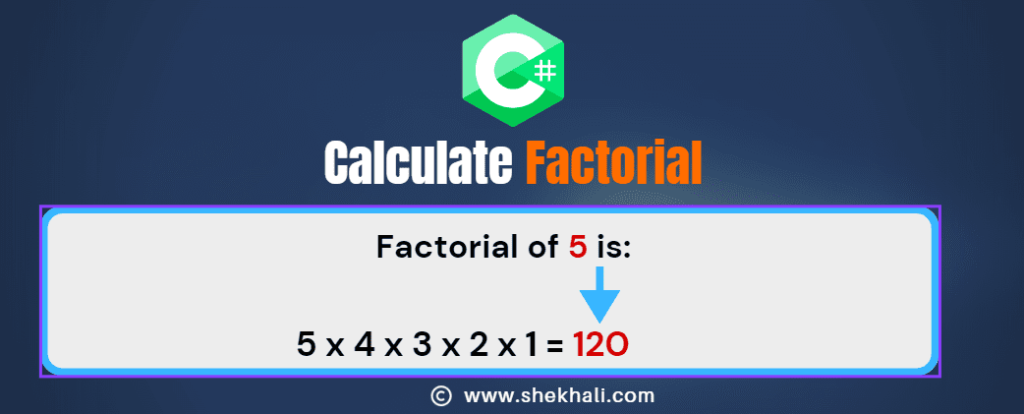