Generics in C# allow you to write type-safe, reusable, and efficient code. In this comprehensive guide, you will learn everything you need to know about generics including how to create generic classes, methods, collections, and generic constraints. This article contains valuable information about C# generics that will help both beginners and experienced C# developers enhance their coding abilities.
C# Generics allow you to reuse the same code (class or method) with different data types. It refers to a generalized version of something rather than a specialized version.
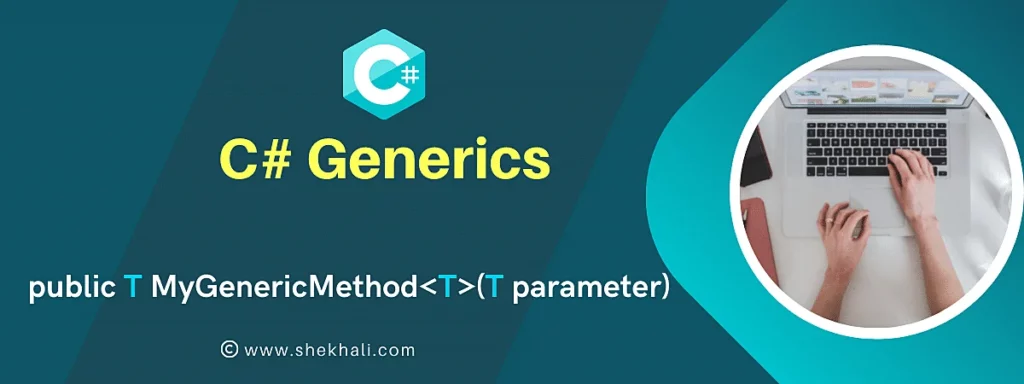