C# Partial classes and partial methods will allow you to split the implementation of a class or a method across multiple files. This can be useful when working with automatically generated code, as it allows you to make changes to the generated code without losing them when the code is regenerated.
The partial
keyword allows you to split the definition of a class, struct, interface, or method into multiple source(.cs) files. Each file contains a part of the definition, and all the parts are combined when the application is compiled.
This article will provide an overview of partial classes and partial methods in C#, including how and why they are implemented.
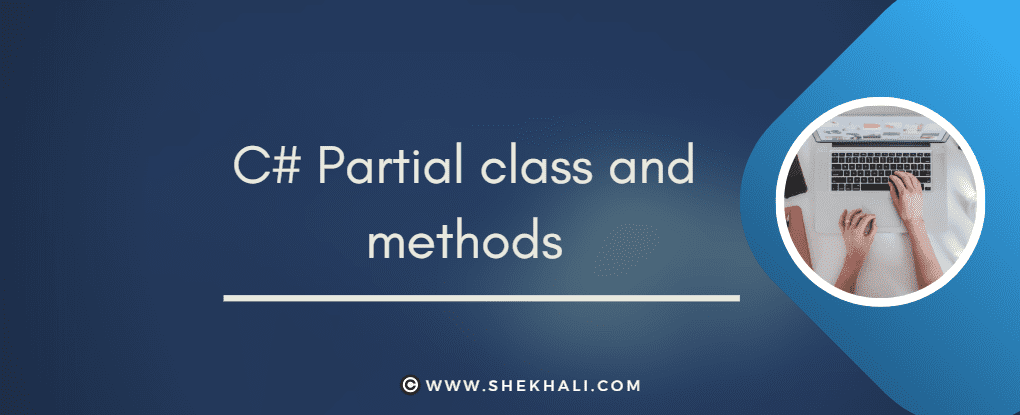